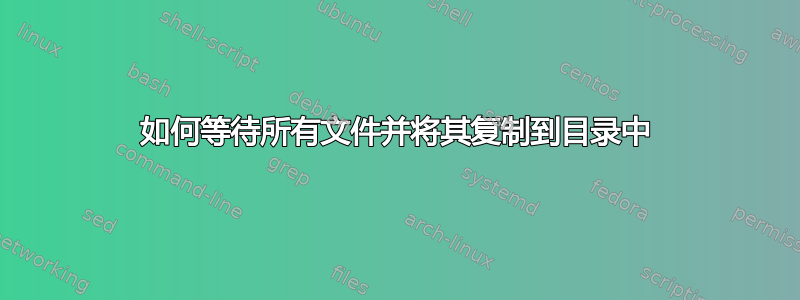
我有 3 个文件,每天都会出现,文件名中带有日期。我需要检查所有 3 个文件的日期是否与今天的日期匹配。并且需要等待3个文件,因为所有文件可能不会同时到达。如果所有文件都到达,则只需将所有 3 个文件复制到目标目录。
下面的示例代码适用于所有文件(如果有)。但我需要制作一些 while 循环或某些 sleep 命令,其中我的脚本可以等待/查找 src 目录中的所有 3 个文件,然后将所有文件一起移动。
#!/bin/ksh
src_dir=/data/SIN/src;
tgt_dir=/data/SIN/tmp;
cd $src_dir;
RUN_DATE=`date +%Y%m%d`;
file1=file1_${RUN_DATE}.txt
file2=file2_${RUN_DATE}.txt
file3=file3_${RUN_DATE}.txt
file_count=`ls -lrt ${src_dir}/*.txt | grep $RUN_DATE | wc -l` ;
if [ "$file_count" == 3 ]
then
echo "all 3 files are available";
cp ${src_dir}/${file1} $tgt_dir;
cp ${src_dir}/${file2} $tgt_dir;
cp ${src_dir}/${file3} $tgt_dir;
echo "files copied";
else
echo "file missing";
fi
答案1
尝试
file_count=$(ls -lrt ${src_dir}/*.txt | grep -c $RUN_DATE)
while [ "$file_count" != 3 ]
do
echo "file missing";
sleep 60
file_count=$(ls -lrt ${src_dir}/*.txt | grep -c $RUN_DATE)
else
echo "all 3 files are available";
cp ${src_dir}/${file1} $tgt_dir;
cp ${src_dir}/${file2} $tgt_dir;
cp ${src_dir}/${file3} $tgt_dir;
echo "files copied";
return
done
注意
- 解析 ls 的输出是一个坏主意,除非你确定你没有任何有趣的字符(空格,新行......)
- 我替换
grep | wc -l
为grep -c
它做同样的事情 - 以同样的方式我将反引号替换为
$( )
编辑:缺少哪个文件?
代替
echo "file missing";
经过
test -f ${src_dir}/${file1} || echo ${file1} missing
test -f ${src_dir}/${file2} || echo ${file2} missing
test -f ${src_dir}/${file3} || echo ${file3} missing
答案2
你已经很接近了,只需if
用循环切换你的语句while
,或者只是按照你的建议添加一个 while 循环就可以解决你的问题。
#!/bin/ksh
src_dir=/data/SIN/src;
tgt_dir=/data/SIN/tmp;
cd $src_dir;
RUN_DATE=`date +%Y%m%d`;
file1=file1_${RUN_DATE}.txt
file2=file2_${RUN_DATE}.txt
file3=file3_${RUN_DATE}.txt
file_count=`ls -lrt ${src_dir}/*.txt | grep $RUN_DATE | wc -l` ;
# check every 5 seconds if the files are all there
while [ "$file_count" -ne 3 ]; do
sleep 5
file_count=`ls -lrt ${src_dir}/*.txt | grep $RUN_DATE | wc -l`
done
if [ "$file_count" == 3 ]
then
echo "all 3 files are available";
cp ${src_dir}/${file1} $tgt_dir;
cp ${src_dir}/${file2} $tgt_dir;
cp ${src_dir}/${file3} $tgt_dir;
echo "files copied";
else
echo "file missing";
fi
这可以工作,但如果该目录中有超过 3 个文件,或者其中一个或多个文件从未出现,它就会挂起。所以我建议在 while 循环中添加一个中断:
# check every 5 seconds if the files are all there, up to 10 times
count=0
while [ "$file_count" -ne 3 ]; do
sleep 5
file_count=`ls -lrt ${src_dir}/*.txt | grep $RUN_DATE | wc -l`
found_files=$(ls -lrt ${src_dir}/*.txt | grep $RUN_DATE)
echo -e "files found:\n\t$found_files" # print the files that have been found so you know which are missing
((count++))
if [ "$count" = 10 ]; then
break
fi
done