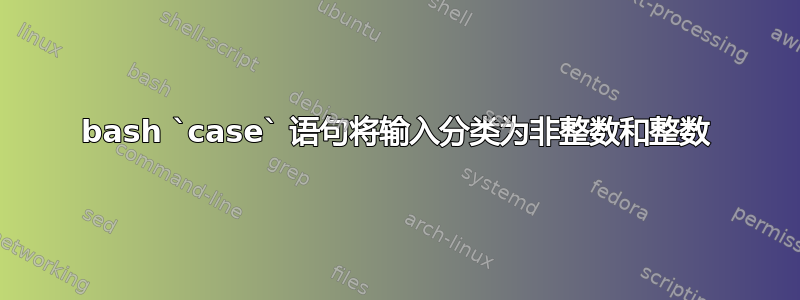
概括:我想使用一个bash
case
语句(在其他代码中)对输入进行分类,以确定它们是否是
- 正整数
- 负整数
- 零
- 一个空字符串
- 非整数字符串
下面是可执行代码,它正确地对以下输入进行分类:
- ”
word
a\nmultiline\nstring
2.1
-3
但将以下两者分类为......负整数:-(
- 0
- 42
细节:
将以下内容保存到文件(例如/tmp/integer_case_statement.sh
),chmod
然后运行它:
#!/usr/bin/env bash
### This should be a simple `bash` `case` statement to classify inputs as
### {positive|negative|zero|non-} integers.
### Trying extglob, since my previous integer-match patterns failed.
### Gotta turn that on before *function definition* per https://stackoverflow.com/a/34913651/915044
shopt -s extglob
declare cmd=''
function identify() {
local -r it=${1} # no quotes in case it's multiline
# shopt -s extglob # can't do that here
case ${it} in
'')
# empty string, no need for `if [[ -z ...`
>&2 echo 'ERROR: null arg'
;;
?(-|+)+([[:digit:]]))
# it's an integer, so just say so and fallthrough
>&2 echo 'DEBUG: int(it), fallthrough'
;&
-+([[:digit:]]))
# it's negative: just return it
>&2 echo 'DEBUG: int(it) && (it < 0), returning it'
echo "${it}"
;;
0)
# it's zero: that's OK
>&2 echo 'DEBUG: int(it) && (it == 0), returning it'
echo '0'
;;
++([[:digit:]]))
# it's positive: just return it
>&2 echo 'DEBUG: int(it) && (it > 0), returning it'
echo "${it}"
;;
*)
# not an integer, just return it
>&2 echo 'DEBUG: !int(it)'
echo "${it}"
;;
esac
} # end function identify
echo -e "'bash --version'==${BASH_VERSION}\n"
echo "identify '':"
identify ''
echo
# > ERROR: null arg
echo 'identify word:'
identify word
echo
# > DEBUG: !int(it)
# > word
echo 'identify a
multiline
string:'
identify 'a
multiline
string'
echo
# > DEBUG: !int(it)
# > a
# > multiline
# > string
echo 'identify 2.1:'
identify 2.1
echo
# > DEBUG: !int(it)
# > 2.1
echo 'identify -3:'
identify -3
echo
# > DEBUG: int(it), fallthrough
# > DEBUG: int(it) && (it < 0), returning it
# > -3
echo 'identify 0:'
identify 0
echo
# > DEBUG: int(it), fallthrough
# > DEBUG: int(it) && (it < 0), returning it
# > 0
echo 'identify 42:'
identify 42
echo
# > DEBUG: int(it), fallthrough
# > DEBUG: int(it) && (it < 0), returning it
# > 42
exit 0
当前输出内联在文件中,但为了便于阅读,以下是我当前的输出:
'bash --version'==4.3.30(1)-release
identify '':
ERROR: null arg
identify word:
DEBUG: !int(it)
word
identify a
multiline
string:
DEBUG: !int(it)
a
multiline
string
identify 2.1:
DEBUG: !int(it)
2.1
identify -3:
DEBUG: int(it), fallthrough
DEBUG: int(it) && (it < 0), returning it
-3
identify 0:
DEBUG: int(it), fallthrough
DEBUG: int(it) && (it < 0), returning it
0
identify 42:
DEBUG: int(it), fallthrough
DEBUG: int(it) && (it < 0), returning it
42
后两个输入是我的问题:为什么 case 语句标识
- 0 作为负整数(而不是 0)
- 42 作为负整数(而不是正整数)
?感谢您的帮助。
答案1
概括:谢谢
我还添加了一个附加子句来检测带符号的零,以及更多测试用例。
细节:
将这个改进的代码保存到一个文件(例如/tmp/integer_case_statement.sh
),chmod
然后运行它:
#!/usr/bin/env bash
### Simple `bash` `case` statement to classify inputs as {positive|negative|zero|non-} integers.
### Trying extglob, since my previous integer-match patterns failed.
### Gotta turn that on before *function definition* per https://stackoverflow.com/a/34913651/915044
shopt -s extglob
declare input=''
### For `case` *patterns* (NOT regexps), see
### https://www.gnu.org/software/bash/manual/html_node/Pattern-Matching.html
function identify() {
local -r it=${1} # no quotes in case it's multiline
# shopt -s extglob # can't do that here
case ${it} in
'')
# empty string, no need for `if [[ -z ...`
>&2 echo 'ERROR: null arg'
;;
[+-]0)
>&2 echo 'ERROR: zero should not be signed'
;;
?(-|+)+([[:digit:]]))
# it's an integer, so just say so and fallthrough
>&2 echo 'DEBUG: int(it), fallthrough'
# ;& # this only runs the next clause, thanks https://unix.stackexchange.com/users/30851/frostschutz
;;& # works
-+([[:digit:]]))
>&2 echo 'DEBUG: it < 0'
;;
0)
>&2 echo 'DEBUG: it == 0'
echo '0'
;;
?(+)+([[:digit:]])) # thanks https://unix.stackexchange.com/users/332764/freddy
>&2 echo 'DEBUG: it > 0'
;;
*)
>&2 echo 'DEBUG: !int(it)'
;;
esac
} # end function identify
echo -e "'bash --version'==${BASH_VERSION}\n"
for input in \
'' \
'@#$%^&!' \
'word' \
'a
multiline
string' \
'2.1' \
'-3' \
'+3' \
'+0' \
'0' \
'-0' \
'42' \
; do
echo "identify '${input}'"
identify "${input}"
ret_val="${?}"
if [[ "${ret_val}" -ne 0 ]] ; then
>&2 echo "ERROR: retval='${ret_val}', exiting ..."
exit 3
fi
echo # newline
done
exit 0
在这个 Debian 工作站上,以上当前输出:
'bash --version'==4.3.30(1)-release
identify ''
ERROR: null arg
identify '@#$%^&!'
DEBUG: !int(it)
identify 'word'
DEBUG: !int(it)
identify 'a
multiline
string'
DEBUG: !int(it)
identify '2.1'
DEBUG: !int(it)
identify '-3'
DEBUG: int(it), fallthrough
DEBUG: it < 0
identify '+3'
DEBUG: int(it), fallthrough
DEBUG: it > 0
identify '+0'
ERROR: zero should not be signed
identify '0'
DEBUG: int(it), fallthrough
DEBUG: it == 0
0
identify '-0'
ERROR: zero should not be signed
identify '42'
DEBUG: int(it), fallthrough
DEBUG: it > 0
感谢您的帮助!