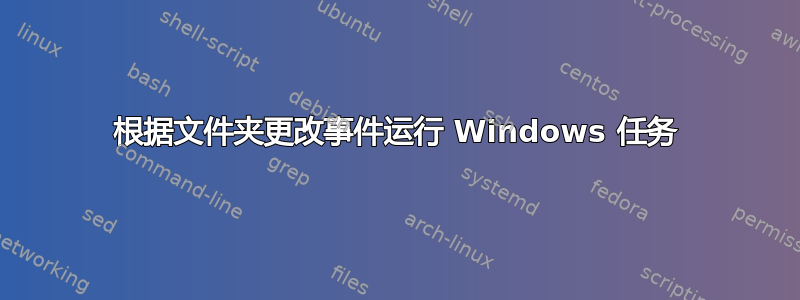
我一直在尝试找到一种基于事件运行 Windows 任务的方法。事件是文件夹中正在更改的文件。该文件正在被我无法控制的另一个进程更改。但是当该文件发生更改时,我需要触发某个 Windows 任务。最好使用 C# 编写解决方案,因为我们公司的政策不允许运行 PowerShell 脚本。
非常感谢您的帮助。
谢谢 Bheki
答案1
我一直在努力寻找这个问题的答案,经过大量研究后,我结合信息和我的经验,找到的答案如下:
//Purpose: Monitor changes to a folder and write to event log when a change
// happens.
//Last Mod date: 09/01/2019
//Author: Bheki
//Notes: The target folder is given in the parameter file. The first time the application runs must be run as an administrator.
using System;
using System.IO;
using System.Security.Permissions;
using System.Diagnostics;
using System.Threading;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Collections.Generic;
using System.Windows;
public class Watcher
{
public static void Main()
{
Run();
}
[PermissionSet(SecurityAction.Demand, Name = "FullTrust")]
public static void Run()
{
// Create a new FileSystemWatcher and set its properties.
FileSystemWatcher watcher = new FileSystemWatcher();
watcher.Path = Watcher.GetTargetFolder().Item1;
/* Watch for changes in LastAccess and LastWrite times, and
the renaming of files or directories. */
watcher.NotifyFilter = NotifyFilters.LastAccess | NotifyFilters.LastWrite
| NotifyFilters.FileName | NotifyFilters.DirectoryName;
// Only watch text files.
watcher.Filter = Watcher.GetTargetFolder().Item2;
// Add event handlers.
watcher.Changed += new FileSystemEventHandler(OnChanged);
watcher.Created += new FileSystemEventHandler(OnChanged);
watcher.Deleted += new FileSystemEventHandler(OnChanged);
//watcher.Renamed += new RenamedEventHandler(OnRenamed);
// Begin watching.
watcher.EnableRaisingEvents = true;
// Wait for the user to quit the program.
Console.WriteLine("Press \'q\' to quit the sample.");
while (Console.Read() != 'q') ;
}
public static Tuple<string,string> GetTargetFolder()
{
string currentRecordOpenFile;
char ColumnDelimter = ","[0];
string TargetFolder = "";
string FixSelected = "";
string FileExtension = "";
string SecondHeader = "";
TargetFolder = System.IO.Directory.GetCurrentDirectory().Replace("\\", "/");
string ParameterFile = TargetFolder + "/ParameterFile.txt";
using (StreamReader objReader = new StreamReader(ParameterFile.Replace("\\", "/")))
{
while (!objReader.EndOfStream)
{
currentRecordOpenFile = objReader.ReadLine(); //this reads a whole line of input from the file
string[] LineOfFile = currentRecordOpenFile.Split(ColumnDelimter);
if (LineOfFile[0] == "Pathtofiles")
{
//we can skip the heading
continue;
}
else
{
TargetFolder = LineOfFile[0].Replace("\\", "/") + "/"; //this can be changed depending on how the output looks
FixSelected = LineOfFile[1];
FileExtension = LineOfFile[2];
SecondHeader = LineOfFile[3];
}
}
return Tuple.Create(TargetFolder, FileExtension);
}//End of stream for reading the parameter file
}
// Define the event handlers.
private static void OnChanged(object source, FileSystemEventArgs e)
{
// Specify what is done when a file is changed, created, or deleted.
Console.WriteLine("File: " + e.FullPath + " " + e.ChangeType);
string vLog = "Application";
string vSource = "BCMPR";
if (!EventLog.SourceExists(vSource))
{
//An event log source should not be created and immediately used.
//There is a latency time to enable the source, it should be created
//prior to executing the application that uses the source.
//Execute this sample a second time to use the new source.
EventLog.CreateEventSource(vSource, vLog);
Console.WriteLine("CreatedEventSource");
Console.WriteLine("Exiting, execute the application a second time to use the source.");
// The source is created. Exit the application to allow it to be registered.
return;
}
// Create an EventLog instance and assign its source.
EventLog myLog = new EventLog();
myLog.Source = vSource;
Console.WriteLine("File: " + e.FullPath + " " + e.ChangeType);
Console.WriteLine("Writting to event log");
// Write an informational entry to the event log.
myLog.WriteEntry("The folder has been changed", EventLogEntryType.Information, 1307);
System.Environment.Exit(0);
}
}
在 C# 中构建应用程序后,将其放置在与应用程序相同的位置。请放置 ParameterFile.txt 文件,其内容如下:
Pathtofiles,FixSelected,FileExtension,SecondHeader
C:\Data\TEST,ALL,*.txt,TEST
请注意,文件夹(C:\Data\TEST)和文件扩展名(*.txt)可以根据用户要求进行更改。
//Windows 事件查看器和设置
运行此代码两次并对文件夹进行更改后,即可在 Windows 事件查看器中看到我们的事件。请按照图中的说明进行操作: Windows 事件日志和任务设置