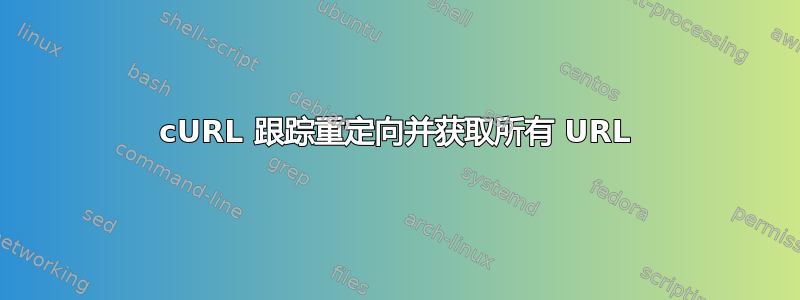
我编写了一个简单的 bash 脚本,它获取 URL 列表并输出一个 CSV,其中包含每个 URL 的一些数据:url、状态代码和目标 url:
while read url
do
urlstatus=$(curl -H 'Cache-Control: no-cache' -o /dev/null --silent --head --insecure --write-out '%{http_code} , %{redirect_url}' "$url" -I )
echo "$url , $urlstatus" >> "$1-out.csv"
done < $1
有时一个 URL 有 2 或 3 个重定向,我想获取所有重定向并将它们打印在输出文件中。
我找到了最后一个 URL 的-L
选项和过滤器:%{url_effective}
urlstatus2=$(curl -H 'Cache-Control: no-cache' -o /dev/null --silent --head --insecure --write-out ' , %{url_effective}' "$url" -L -I )
但我想拥有从起始地址到最终地址的所有 URL,并将它们添加到 csv 中。
答案1
制作一个递归函数:
#!/bin/bash
get_redirects(){
i=${2:-1}
read status url <<< $(curl -H 'Cache-Control: no-cache' -o /dev/null --silent --head --insecure --write-out '%{http_code}\t%{redirect_url}\n' "$1" -I)
printf '%d: %s --> %s\n' "$i" "$1" "$status";
if [ "$1" = "$url" ] || [ $i -gt 9 ]; then
echo "Recursion detected or more redirections than allowed. Stop."
else
case $status in
30*) get_redirects "$url" "$((i+1))"
;;
esac
fi
}
用法:
$ get_redirects https://aep-beta.onpc.fr/lycees/dom/region/DOM/ECOL
https://aep-beta.onpc.fr/lycees/dom/region/DOM/ECOL --> 301
https://aep-beta.onpc.fr/onglet/lycee/dom --> 301
https://aep-beta.onpc.fr/onglet/lycee/outre-mer --> 200
答案2
建立在@pLumo的答案,这个函数消除了递归性并允许将自定义参数提供给curl
#!/bin/bash
get_redirects(){
url=$1
declare -a params=("${@:2}")
for i in {1..100}; do
read status url <<< $(curl "$url" -H 'Cache-Control: no-cache' -o /dev/null --silent --write-out '%{http_code}\t%{redirect_url}\n' "${params[@]}");
printf '%d: %s --> %s\n' "$i" "$url" "$status";
if (( status < 300 || status >= 400 )); then break; fi
done
}
用法:
get_redirects http://example.com/endpoint -X POST -H "Authentication: Bearer ..." -d "param=value"