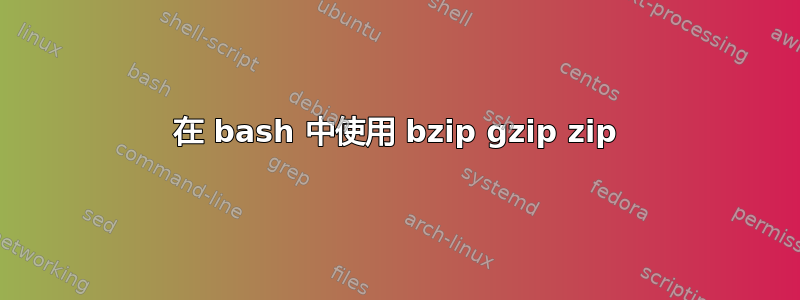
#!/bin/bash
# check if the user put a file name
if [ $# -gt 0 ]; then
# check if the file is exist in the current directory
if [ -f "$1" ]; then
# check if the file is readable in the current directory
if [ -r "$1" ]; then
echo "File:$1"
echo "$(wc -c <"$1")"
# Note the following two lines
comp=$(bzip2 -k $1)
echo "$(wc -c <"$comp")"
else
echo "$1 is unreadable"
exit
fi
else
echo "$1 is not exist"
exit
fi
fi
目前我的问题是我可以通过bzip将$1
文件压缩成一个$1.c.bz2
文件,但是如果我来捕获压缩文件的大小怎么办?我的代码显示没有此类文件。
答案1
我为你清理了代码:
#!/bin/bash
#check if the user put a file name
if [ $# -gt 0 ]; then
#check if the file is exist in the current directory
if [ -f "$1" ]; then
#check if the file is readable in the current directory
if [ -r "$1" ]; then
echo "File:$1"
echo "$(wc -c <"$1")"
comp=$(bzip2 -k $1)
echo "$(wc -c <"$comp")"
else
echo "$1 is unreadable"
exit
fi
else
echo "$1 is not exist"
exit
fi
fi
fi
请注意倒数第二行中的附加内容。
现在,第 11 行没有多大意义,因为bzip2 -k test.file
没有创建任何输出。因此该变量comp
只是空的。
一个简单的方法是简单地知道扩展名是.bz2
,所以你可以这样做:
echo "$(wc -c <"${1}.bz2")"
comp
并且根本不使用该变量。
答案2
bzip2 -k
不输出任何内容,因此在命令替换中运行它并捕获变量中的输出comp
将使该变量为空。这个空变量是您的主要问题。使用它也stat
比wc -c
随意获取文件的大小更好,因为更有效,stat
只是从文件的元数据中读取文件大小,而不必费心从文件中读取所有数据。
您还对文件存在性和可读性进行了许多不必要的检查,这些检查分散了脚本的注意力。这些检查bzip2
无论如何都会执行,您可以利用这一事实来避免自己测试其中的大部分内容。
建议:
#!/bin/sh
pathname=$1
if ! bzip2 -k "$pathname"; then
printf 'There were issues compressing "%s"\n' "$pathname"
echo 'See errors from bzip2 above'
exit 1
fi >&2
size=$( stat -c %s "$pathname" )
csize=$( stat -c %s "$pathname.bz2" )
printf '"%s"\t%s --> %s\n' "$pathname" "$size" "$csize"
该脚本使用 的退出状态bzip2
来确定压缩文件是否顺利。如果失败,它会输出一条诊断消息,以补充bzip2
终端中已生成的诊断消息。
由于您使用-k
withbzip2
来保留未压缩的文件,因此可以在语句之后获得两个文件的大小if
。如果要从-k
命令中删除,您自然必须在if
语句之前获取未压缩文件的大小。
脚本中使用的方式stat
假设该脚本运行在Linux系统上。该stat
实用程序是非标准的,并与其他系统上的其他选项一起实现。例如,在 macOS 或 OpenBSD 上,您必须使用stat -f %z
来代替stat -c %s
.
测试:
$ ./script
bzip2: Can't open input file : No such file or directory.
There were issues compressing ""
See errors from bzip2 above
$ ./script nonexisting
bzip2: Can't open input file nonexisting: No such file or directory.
There were issues compressing "nonexisting"
See errors from bzip2 above
$ ./script file
"file" 600 --> 43
$ ./script file
bzip2: Output file file.bz2 already exists.
There were issues compressing "file"
See errors from bzip2 above
$ rm -f file.bz2
$ chmod u-r file
$ ./script file
bzip2: Can't open input file file: Permission denied.
There were issues compressing "file"
See errors from bzip2 above