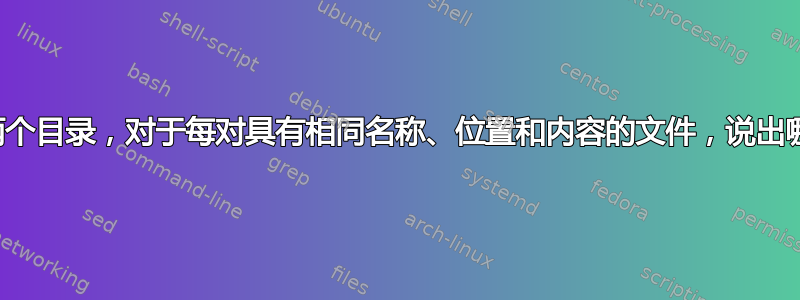
在Linux shell中,如何递归比较两个目录,并且对于两个目录中位置相同(包括名称)且内容相同、修改时间不同的每一对文件(包括符号链接和目录),说出哪个文件是年纪大了?如果一对中的两个文件具有相同的年龄,则该对应该没有输出。如果一对中的两个文件具有不同的内容(且位置相同),则该对不应有输出。
使用示例:
# First, ensure that /tmp/d1, /tmp/d2, ~/d3, and ~/f don't exist. Then:
$ cd /tmp
$ mkdir d1 d2 ~/d3
$ touch d1/f && sleep 1 && touch d2/f ~/d3/f ~/f
$ echo "g1" > d1/g
$ echo "g2" > d2/g
$ echo "g3" > ~/d3/g
$ compare_times.sh d1 d2
d1/f is older than d2/f
$ cd d1
$ compare_times.sh . ../d2
f is older than ../d2/f
$ cd ../d2
$ compare_times.sh . ../d1
../d1/f is older than f
$ cd ..
$ compare_times.sh /tmp/d1 d2
/tmp/d1/f is older than d2/f
$ compare_times.sh d1 /tmp/d2
d1/f is older than /tmp/d2/f
$ compare_times.sh d1 ~/d3
d1/f is older than ~/d3/f
$ cd d1
$ compare_times.sh ~ .
f is older than ~/f
我们的比较脚本compare_times.sh
(当然,compare_times.zsh
如果您碰巧在桀骜代替短跑或者巴什) 应该接受两个参数,通常可以是目录的绝对路径或相对路径(包括简单的.
),可能以 结尾/
。
不带引号的字符串~
应像往常一样在两个参数中解释为主目录。在输出中实际打印主目录可能有点过分了;~
输出应该简洁。
输出应该像往常一样简洁:例如,路径
答案1
该脚本将根据您的要求比较两个目录树。文件名不受限制。 (如果您没有 GNU comm
、find
andsort
或其他可以处理 NULL 终止记录的等效工具,您将不得不放弃处理文件名中换行符的能力。)
#!/bin/bash
#
d1=$1 d2=$2
# Identify the set of matching file names
LC_ALL=C comm -z -12 \
<( cd -P -- "$d1" && find . -type f -print0 | LC_ALL=C sort -z ) \
<( cd -P -- "$d2" && find . -type f -print0 | LC_ALL=C sort -z ) |
while IFS= read -rd '' fn
do
# Tidy the filenames
fn=${fn#./}
f1="$d1/$fn"
f2="$d2/$fn"
# Compare content
if cmp -s -- "$f1" "$f2"
then
# Report older/newer file pairs (not those the same)
[[ "$f1" -ot "$f2" ]] && printf '%s is older than %s\n' "${f1#./}" "${f2#./}"
[[ "$f2" -ot "$f1" ]] && printf '%s is older than %s\n' "${f2#./}" "${f1#./}"
fi
done
答案2
和zsh
:
#! /bin/zsh -
d1=${1?} d2=${2?}
# All regular files in $d1, with $d1/ removed from the start of their
# path in the second step
l1=( ${d1?}/**/*(ND-.) ); l1=( ${l1#$d1/} )
# same for $2
l2=( ${d2?}/**/*(ND-.) ); l2=( ${l2#$d2/} )
# loop over the intersection of the $l1 and $l2 arrays
for f ( ${l1:*l2} ) {
f1=$1/$f f2=$2/$f
cmp -s -- $f1 $f2 || continue
if [[ $f1 -nt $f2 ]] {
print -r -- $f1 is newer than $f2
} elif [[ $f1 -ot $f2 ]] {
print -r -- $f1 is older than $f2
} else {
print -r -- $f1 and $f2 are the same age
}
}
我并不是想/home/your-home-dir
用~
或 ./file
用file
或/path/to/current/dir/foo/bar
用替换foo/bar
,因为在我看来,这只会引入歧义和混乱,还会使代码更加复杂。
如果您仍然愿意,可以采取多种方法。使用将存储ind1=${1:P}
的绝对规范路径。作为完整路径将更容易识别其中的, ,但如果或 的扩展实际上包含符号链接组件,则会崩溃。符号链接已解析的事实很容易让用户感到困惑。$1
$d1
~
~user
$HOME
~user
折衷方案是仅进行最安全的路径简化,例如简化 to //./././/
,并从路径的开头/
删除$PWD/
和,然后使用./
D
参数扩展标志~user
在路径中进行识别。例如,您可以使用以下内容计算$display_f1
from $f1
(对于 也同样$f2
):
set -o extendedglob
display_f1=${(D)${${f1//\/((.|)\/)##/\/}#$PWD/}#./}
例如:
$ f1='///home/././chazelas//foo/bar baz'
$ pwd
/home/chazelas
$ display_f1=${(D)${${f1//\/((.|)\/)##/\/}#$PWD/}#./}
$ typeset display_f1
display_f1='foo/bar\ baz'
$ cd /
$ display_f1=${(D)${${f1//\/((.|)\/)##/\/}#$PWD/}#./}
$ typeset display_f1
display_f1='~/foo/bar\ baz'
$ f1='./~/foo'
$ display_f1=${(D)${${f1//\/((.|)\/)##/\/}#$PWD/}#./}
$ typeset display_f1
display_f1='\~/foo'
但:
$ cd /home
$ f1=./chazelas/foo
$ display_f1=${(D)${${f1//\/((.|)\/)##/\/}#$PWD/}#./}
$ typeset display_f1
display_f1=chazelas/foo
(并不是~/foo
)。
包含该内容的完整解决方案如下所示:
#! /bin/zsh -
set -o extendedglob
d1=${1?} d2=${2?}
# All regular files in $d1, with $d1/ removed from the start of their
# path in the second step
l1=( ${d1?}/**/*(ND-.) ); l1=( ${l1#$d1/} )
# same for $2
l2=( ${d2?}/**/*(ND-.) ); l2=( ${l2#$d2/} )
# loop over the intersection of the $l1 and $l2 arrays
for f ( ${l1:*l2} ) {
f1=$1/$f f2=$2/$f
display_f1=${(D)${${f1//\/((.|)\/)##/\/}#$PWD/}#./}
display_f2=${(D)${${f2//\/((.|)\/)##/\/}#$PWD/}#./}
cmp -s -- $f1 $f2 || continue
if [[ $f1 -nt $f2 ]] {
print -r -- $display_f1 is newer than $display_f2
} elif [[ $f1 -ot $f2 ]] {
print -r -- $display_f1 is older than $display_f2
} else {
print -r -- $display_f1 and $display_f2 are the same age
}
}
答案3
对于常规文件、符号链接和目录,基于http://stackoverflow.com/a/66468913和http://unix.stackexchange.com/a/767825(感谢两位!):
#!/bin/bash
# Syntax: compare_times.sh directory_1 directory_2
# Semantics: for pairs of files, directories, and symlinks with the same path at any depth in both directory_1 and directory_2 and the same type, compare their modification times and, if they differ, say which file/dir/symlink is older.
# thanks to http://stackoverflow.com/a/66468913. The original is in http://github.com/python/cpython/blob/main/Lib/posixpath.py#L377 .
normpath() {
local IFS=/ initial_slashes='' comp comps=()
if [[ $1 == /* ]]; then
initial_slashes='/'
[[ $1 == //* && $1 != ///* ]] && initial_slashes='//'
fi
for comp in $1; do
if [[ -n ${comp} && ${comp} != '.' ]]; then
if [[ ${comp} != '..' || (-z ${initial_slashes} && ${#comps[@]} -eq 0) || (${#comps[@]} -gt 0 && ${comps[-1]} == '..') ]]; then
comps+=("${comp}")
elif ((${#comps[@]})); then
unset 'comps[-1]'
fi
fi
done
comp="${initial_slashes}${comps[*]}"
printf '%s\n' "${comp:-.}"
}
# thanks to http://unix.stackexchange.com/a/767825
if [[ $# -eq 2 ]]; then
dir1=$1
if [[ -d $dir1 ]]; then
dir2=$2
if [[ -d $dir2 ]]; then
# Identify the set of matching names of regular files:
LC_ALL=C comm -z -12 \
<( cd -P -- "$dir1" && find . -type f -print0 | LC_ALL=C sort -z ) \
<( cd -P -- "$dir2" && find . -type f -print0 | LC_ALL=C sort -z ) |
while IFS= read -rd '' fn; do
# Tidy the file names:
file1="$(normpath "$dir1/$fn")"
file2="$(normpath "$dir2/$fn")"
# Compare file contents:
if cmp -s -- "$file1" "$file2"; then
# Report older/newer regular-file pairs:
if [[ "$file1" -ot "$file2" ]]; then
printf '%s is older than %s\n' "$file1" "$file2"
elif [[ "$file2" -ot "$file1" ]]; then
printf '%s is older than %s\n' "$file2" "$file1"
fi
fi
done
# Identify the set of matching names of symlinks:
LC_ALL=C comm -z -12 \
<( cd -P -- "$dir1" && find . -type l -print0 | LC_ALL=C sort -z ) \
<( cd -P -- "$dir2" && find . -type l -print0 | LC_ALL=C sort -z ) |
while IFS= read -rd '' fn; do
# Tidy the link names:
link1="$(normpath "$dir1/$fn")"
link2="$(normpath "$dir2/$fn")"
# Compare the symlinks:
if cmp -s <(readlink -- "$link1") <(readlink -- "$link2"); then
## Report older/newer symlink pairs:
# if (find "$link2" -prune -newer "$link1" -printf 'a\n' | grep -q a) then
# printf '%s is older than %s\n' "$link1" "$link2"
# elif (find "$link1" -prune -newer "$link2" -printf 'a\n' | grep -q a) then
# printf '%s is older than %s\n' "$link2" "$link1"
# fi
find "$link2" -prune -newer "$link1" -printf "$link1 is older than $link2\n"
find "$link1" -prune -newer "$link2" -printf "$link2 is older than $link1\n"
fi
done
# Identify the set of matching names of directories:
LC_ALL=C comm -z -12 \
<( cd -P -- "$dir1" && find . -type d -print0 | LC_ALL=C sort -z ) \
<( cd -P -- "$dir2" && find . -type d -print0 | LC_ALL=C sort -z ) |
while IFS= read -rd '' fn; do
# Tidy the directory names:
subdir1="$(normpath "$dir1/$fn")"
subdir2="$(normpath "$dir2/$fn")"
# Compare the directory contents:
if cmp -s <(ls -Al --full-time -- "$subdir1") <(ls -Al --full-time -- "$subdir2"); then
# Report older/newer directory pairs:
if [[ "$subdir1" -ot "$subdir2" ]]; then
printf '%s is older than %s\n' "$subdir1" "$subdir2"
elif [[ "$subdir2" -ot "$subdir1" ]]; then
printf '%s is older than %s\n' "$subdir2" "$subdir1"
fi
fi
done
else
printf '%s is not an existing directory.\n' "$dir2"
exit 66 # EX_NOINPUT
fi
else
printf '%s is not an existing directory.\n' "$dir1"
exit 66 # EX_NOINPUT
fi
else
echo "Wrong number of arguments." >&2
exit 64 # EX_USAGE
fi
欢迎改进。