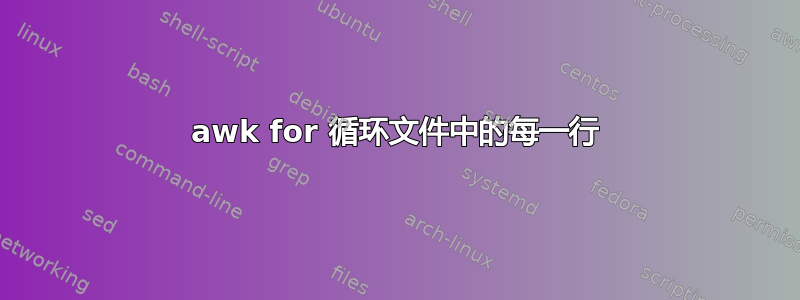
我需要弄清楚如何循环遍历文本文件,并为每一行获取 name 、project # 和 email 字段,并将它们替换到要发送的电子邮件模板中。
这是名为 send.txt 的文本文件:
Project 1,Jack,Chen,06,12,[email protected]
Project 2,Emily,Weiss,06,12,[email protected]
Project 3,Mary,Gonzalas,06,12,[email protected]
这是名为 Reminder.email 的电子邮件模板:
Dear __FULLNAME__:
This is a kindly reminder that our __Project__ meeting will be held on today.
Best Regards,
CIS5027
因此,对于文本文件中的每一行,我需要在此电子邮件模板中替换以下字段全名: , 和项目。使用我可以为第一行执行的相应值,但是我无法为每一行执行此操作。
这是我的脚本
#
!/bin/sh
#Start your code from here
date= date "+%m/%d/%y"
print $date
#The following line is to scan a file called Event-member.data and return any lines with todays date and save them to a file called sendtoday.txt
grep -n $(date +"%m,%d") Event-member.data > sendtoday.txt
#The following line is to remove the first to characters of the file created above sendtoday.txt and output that to a file called send.txt.
cat sendtoday.txt | sed 's/^..//' > send.txt
#This is where im having trouble. When storing the values for the variables below of name, email, project #. The value of NR==1 thus it never goes through the rest of the lines. I've tried different solutions but none seem to work.
p=$(awk -F ',' 'NR==1{print $1}' send.txt)
n=$(awk -F ',' 'NR==1{print $2}' send.txt)
l=$(awk -F ',' 'NR==1{print $3}' send.txt)
e=$(awk -F ',' 'NR==1{print $6}' send.txt)
echo $p $n $l $e
#This part is to replace the values in the email template using sed and save the modified template as sendnow.txt.
sed -e "s/__FULLNAME__:/\ $n $l :/g;s/__Project__/\ $p /g" Reminder.email > sendnow.txt
cat sendnow.txt
#Yet to be written ... send out modified email templates.
exit 0
########
这是它产生的输出:
06/12/14
Project 1 Jack Chen [email protected]
Dear Jack Chen :
This is a kindly reminder that our Project 1 meeting will be held on today.
Best Regards,
CIS5027
正如你所看到的,它确实正确地取代了字段,但仅限于陈杰克。 send.txt 文件中有 3 行,因此上述模板必须有 3 个修改版本。
答案1
没有理由使用awk
它。您可以直接在 shell 中使用read
。一般格式是read foo bar
将第一个字段保存为 ,$foo
每行的其余部分保存为$bar
。因此,就您而言,您会执行以下操作:
while IFS="," read p n l foo bar e; do
sed -e "s/__FULLNAME__:/\ $n $l :/g;s/__Project__/\ $p /g" Reminder.email;
done < file
是IFS
输入字段分隔符,当设置为,
读取逗号分隔字段时。这使您可以获取每个字段并将其存储在变量中。请注意,我使用了两个额外的变量foo
和bar
。这是因为每个字段都需要自己的变量名,而您有 6 个字段。如果您只给出 4 个变量名称,则第 4 个 ( $e
) 将包含字段 4 到最后一个。
现在,您的脚本中存在各种其他语法错误。首先shebang行是错误的,你需要,在和之间#! /bin/sh
不能有空行。另外,为了分配#!
/bin/sh
输出将命令传递给变量时,需要使用var=`command`
or ,最好使用var=$(command)
格式。否则,命令本身作为字符串而不是其输出分配给变量。最后,print
不是你想的那样。您正在寻找printf
或echo
。因此,编写脚本的更好方法是:
#!/bin/sh
date=$(date "+%m/%d/%y")
echo $date
## The following line is to scan a file called Event-member.data
## and return any lines with todays date and save them to a file
## called sendtoday.txt
grep -n $(date +"%m,%d") Event-member.data > sendtoday.txt
## The following line is to remove the first to characters of the file
## created above sendtoday.txt and output that to a file called
## send.txt.
## I rewrote this to avoid the useless use of cat.
sed 's/^..//' sendtoday.txt > send.txt
## This is where you use read
while IFS="," read p n l foo bar e; do
sed -e "s/__FULLNAME__:/\ $n $l :/g;s/__Project__/\ $p /g" Reminder.email > sendnow.txt
cat sendnow.txt
## This is where you need to add the code that sends the emails. Something
## like this:
sendmail $e < sendnow.txt
done < send.txt
exit 0
########
答案2
您使用了 NR==1 条件NR==1{print $1}
。这意味着它将考虑 的第一行send.txt
。使用 NR==2 条件获取第二行,依此类推。或者使用循环遍历所有行,例如
while read line
do
p=`echo $line | awk -F '.' '{print $1}'`
n=`echo $line | awk -F '.' '{print $2}'`
l=`echo $line | awk -F '.' '{print $3}'`
e=`echo $line | awk -F '.' '{print $1}'`
sed -e "s/\__FULLNAME\__:/\ $n $l :/g;s/\__Project__/\ $p /g" Reminder.email > sendnow.txt
done<send.txt
答案3
也许将你的脚本包装成类似的东西
for i in $(cat send.txt); do echo "line: $i"; done
?
答案4
cat xtmp | awk '{s++; do_aything_you_want_here }'
**s++ 遍历每一行 **
cat xtmp
111
222
333
444
cat xtmp | awk '{s++; print($1,"QQQ") }'
111 QQQ
222 QQQ
333 QQQ
444 QQQ
cat xtmp | awk '{s++; print($1+3,"QQQ") }'
114 QQQ
225 QQQ
336 QQQ
447 QQQ
cat xtmp | awk '{s++; for(i=1; i<=4 ; i++) print $1 }' # this will repeat each line 4 time
cat tmp | awk '{s++; a=a+$1; print(s" "$1" "a)}' instead of a=a+$1 you can use a+=$1
1 111 111
2 222 333
3 333 666
4 444 1110