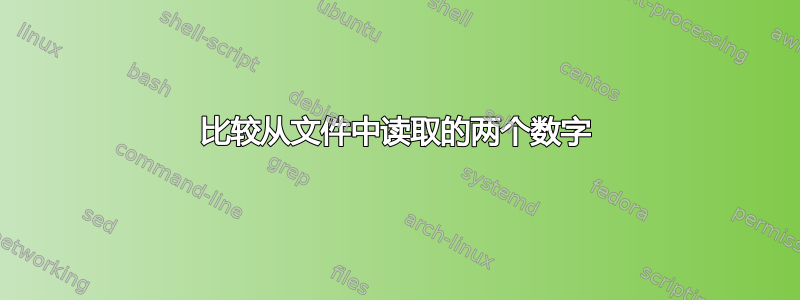
我有一个脚本可以读取标准格式的文件,其中第 9 个单词是数字。我正在尝试比较从文件中读取的数字。我能够正确地读取该行,并且它完全按照我想要的方式工作。但我收到一条错误消息:
./age.sh: line 8: [: age: integer expression expected
这是我的脚本:
#!/bin/bash
if [ -f $1 ] ;
then
while read -r LINE || [[ -n $LINE ]]; do
name=$( echo $LINE | cut -d " " -f1 -f2)
ago=$( echo $LINE | cut -d " " -f9)
echo "$name ----- $age"
if [ $ago -gt 30 ] ; then
echo "You get a discount"
fi
done < $1
else
echo "No file found"
fi
这是一个示例输入文件
#FirstName LastName SuperheroName Powers Weapons City Enemy isOutOfEarth Age
Bruce Wayne Batman Martial_arts No_Guns Gowtham Joker No 31
Clark Kent Superman Extreme_strength None Metropolitan Lex_Luther Yes 32
Oliver Queen Green_arrow Accuracy Bow_and_Arrow Star_city Cupid No 30
答案1
您收到的具体错误是因为您的脚本也在处理文件的标头。一个简单的解决方法是跳过以 开头的行#
:
#!/bin/bash
if [ ! -f "$1" ]; then
echo "No file found"
exit 1
fi
## Use grep -v to print lines that don't match the pattern given.
grep -v '^#' "$1" |
while read -r LINE || [ -n "$LINE" ]; do
name=$( echo "$LINE" | cut -d " " -f1,2)
age=$( echo "$LINE" | cut -d " " -f9)
echo "$name ----- $age"
if [ "$age" -gt 30 ]; then
echo "You got a discount"
fi
done
但是,由于您可能还想对其他列进行操作,因此我将直接将它们全部读入变量中:
#!/bin/bash
if [ ! -f "$1" ]; then
echo "No file found"
exit 1
fi
## read can take multiple values and splits the input line on whitespace
## automatically. Each field is assigned to one of the variables given.
## If there are more fields than variable names, the remaining fields
## are all assigned to the last variable.
grep -v '^#' "$1" | while read -r first last super powers weapons city enemy isout age; do
echo "$first $last ----- $age"
if [ "$age" -gt 30 ]; then
echo "You got a discount"
fi
done
答案2
#!/bin/bash
if [ ! -f "$1" ]; then
echo "No file found"
exit 1
fi
exec < $1
while read -r LINE || [ -n "$LINE" ]; do
name=$( echo "$LINE" | cut -d " " -f1,2)
age=$( echo "$LINE" | cut -d " " -f9)
echo "$name ----- $age"
if [ "$age" -gt 30 ]; then
echo "You got a discount"
fi
done
答案3
这就是我所做的:
#!/bin/bash
if [ -f $1 ] ;
then
sum=0
echo "#FirstName LastName City Age"
while read -r LINE || [[ -n $LINE ]]; do
name=$( echo $LINE | cut -d " " -f1 -f2)
city=$( echo $LINE | cut -d " " -f3)
age=$( echo $LINE | cut -d " " -f9)
check=$( echo $amount | grep -c "[0-9]")
if [ $check -gt 0 ]; then
if [ $age -gt 30 ] ; then
echo "You get a discount"
fi
fi
done < $1
else
echo "No file found"
fi
答案4
我将该行读入 bash 数组:
if [[ -f $1 ]] ; then
while read -ra line; do
(( ${#line[@]} > 0 )) || continue # skip empty lines
name=${line[*]:0:2}
age=${line[-1]}
echo "$name ----- $age"
if (( $age > 30 )); then
echo "You get a discount"
fi
done < "$1"
else
echo "No file found"
fi
#FirstName LastName ----- Age
Bruce Wayne ----- 31
You get a discount
Clark Kent ----- 32
You get a discount
Oliver Queen ----- 30
这也不会因为 $age 是非整数而受到困扰:
$ age=Age
$ [ $age -gt 30 ] && echo old || echo young
bash: [: Age: integer expression expected
young
$ (( $age > 30 )) && echo old || echo young
young
$ [[ $age -gt 30 ]] && echo old || echo young
young