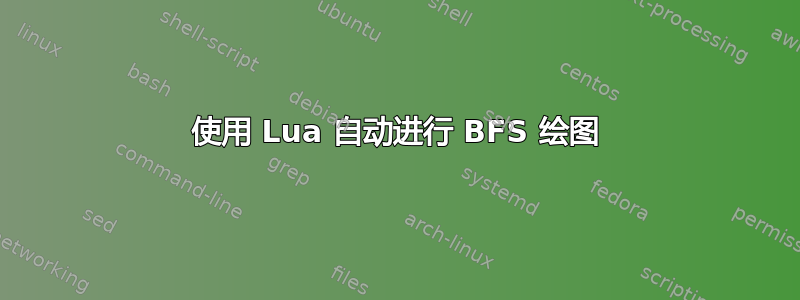
我尝试用 TeX 来学习 Lua。为此,我尝试以某种方式实现 BFS,以便它可以自动创建 Tikz 图片。下面是我所做的一个简短示例:
\documentclass{article}
\usepackage{luacode}
\usepackage{tikz}
\usetikzlibrary{graphs,graphdrawing}
\usegdlibrary{force}
\begin{luacode}
tp=tex.print
bfs_initial_graph = {}
bfs_current = {}
bfs_visited = {}
bfs_in_queue = {}
bfs_unvisited = {}
function bfs_init(graph, start)
-- initialize bfs_current with start node
bfs_current = {start}
-- initialize bfs_visited as an empty set
bfs_visited = {}
-- initialize bfs_in_queue with nodes reachable from start node
bfs_in_queue = {}
for _, neighbor in ipairs(graph[start]) do
table.insert(bfs_in_queue, neighbor)
end
bfs_unvisited = {}
for node, neighbors in pairs(graph) do
if node ~= start then
table.insert(bfs_unvisited, node)
end
end
end
function bfs_step()
-- check if bfs_in_queue is empty
if #bfs_in_queue == 0 then
return false
end
-- move current node from bfs_current to bfs_visited
local current_node = table.remove(bfs_current, 1)
table.insert(bfs_visited, current_node)
-- move first node from bfs_in_queue to bfs_current
local next_node = table.remove(bfs_in_queue, 1)
table.insert(bfs_current, next_node)
-- update bfs_unvisited and bfs_in_queue
for _, neighbor in ipairs(bfs_initial_graph[next_node]) do
if bfs_unvisited[neighbor] then
bfs_unvisited[neighbor] = nil
table.insert(bfs_in_queue, neighbor)
end
end
return true
end
function bfs_draw_graph()
-- start TikZ picture
tp("\\begin{tikzpicture}[>=stealth, every node/.style={circle, draw, minimum size=0.75cm}]")
-- loop through nodes in the initial graph and draw them
for node, neighbors in pairs(bfs_initial_graph) do
-- determine color based on BFS list membership
local color = "gray"
if node == bfs_current[1] then
color = "red"
elseif table.contains(bfs_in_queue, node) then
color = "yellow"
elseif table.contains(bfs_visited, node) then
color = "green"
end
-- draw node with appropriate color
tp(string.format("%s [color=%s]", node, color))
-- draw edges to neighbors
for _, neighbor in ipairs(neighbors) do
tp(string.format("%s -- %s", node, neighbor))
end
end
-- end TikZ picture
tp("\\end{tikzpicture}")
end
function bfs_run()
-- run BFS until bfs_in_queue is empty
while #bfs_in_queue > 0 do
bfs_next_step()
end
end
\end{luacode}
\newcommand{\BfsInit}[2]{\luaexec{bfs_init(#1, #2)}}
\newcommand{\BfsNextStep}{\luaexec{bfs_draw_graph()}}
\newcommand{\BfsAllSteps}{\luaexec{bfs_run()}}
\begin{document}
\BfsInit{
a -- {b,c},
b -- {a,c},
c -- {a,b},}
{a}
\BfsNextStep
\end{document}
遗憾的是它无法运行,我不知道如何让它运行。对于我这个初学者来说,它看起来是正确的,但我不知道错误在哪里。有人能帮我运行并向我解释我的错误在哪里吗?非常感谢!!!