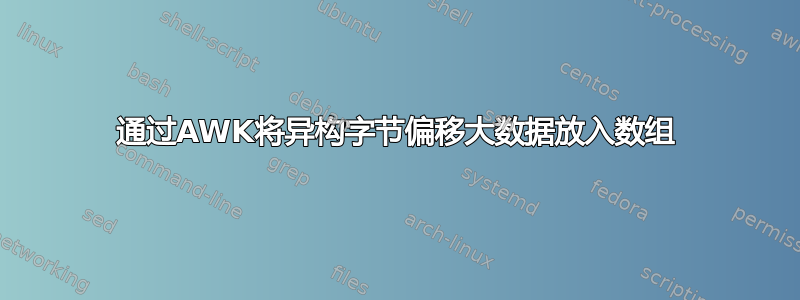
假设数据由不固定的字节偏移组成,即两个后续文件头的距离不同。该线程的要点是在数组中分别遍历每个大小的事件。
示例数据
fafafafa
00005e58
da1e5afe
00000000
*
fafafafa
00005e58
da1e5afe
00000000
*
00000001
ffffffff
555eea72
00000000
*
00000004
fafafafa
01da1300
*
00000004
02991c00
fafafafa
01da1300
fafafafa
01da1300
fafafafa
01da1300
其中字段分隔符是fafafafa
。
我的建议
#!/bin/bash
# http://stackoverflow.com/a/10383546/54964
# http://unix.stackexchange.com/a/209789/16920
myarr = ($( cat 25.6.2015_test.txt | awk -F 'fafafafa' '$1~/^[a-z0-9*]+$/ {print $1}') )
# http://stackoverflow.com/a/15105237/54964
# Now access elements of an array (change "1" to whatever you want)
echo ${myarr[1]}
# Or loop through every element in the array
for i in "${myarr[@]}"
do
:
echo $i
done
脚本整体运行
输出
awk2array.sh: line 5: syntax error near unexpected token `('
awk2array.sh: line 5: `myarr = ($( cat 25.6.2015_test.txt | awk -F 'fafafafa' '$1~/^[a-z0-9*]+$/ {print $1}') ) '
我不明白,因为甚至括号。我想将输出放入数组中或将每个事件存储到以算术命名的文件中(0.txt,1.text,...,n.txt)。我现在分别描述一些命令和一些我不确定的代码部分。
AWK命令单独运行
单独运行时,AWK 命令会省略字段分隔符,从而给出
00005e58
da1e5afe
00000000
*
00005e58
da1e5afe
00000000
*
00000001
ffffffff
555eea72
00000000
*
00000004
01da1300
*
00000004
02991c00
01da1300
01da1300
01da1300
想要的输出是将所有数据放在数组中,其中字段分隔符fafafafa
应fafafafa
包含在单元格中,例如
Value of first cell in array
----------------------------
fafafafa
00005e58
da1e5afe
00000000
*
Value of second cell
--------------------
fafafafa
00005e58
da1e5afe
00000000
*
00000001
ffffffff
555eea72
00000000
*
00000004
3rd cell
--------
01da1300
*
00000004
02991c00
4th cell
--------
fafafafa
01da1300
5th cell
--------
fafafafa
01da1300
6th cell
--------
fafafafa
01da1300
如何通过AWK将大数据存储到N数组中?您还可以在读取每个事件后将其存储到文件中,而无需再次开始读取文件并从左侧的点继续。
答案1
问题
这里有很多问题
#!/bin/bash
myarr = (
它之间有一个空格,这意味着即使它运行,也不会分配任何内容。
cat 25.6.2015_test.txt | awk
awk可以打开自己的文件,不需要cat
-F 'fafafafa' '$1~/^[a-z0-9*]+$/
-F 是字段分隔符而不是记录,因此所做的只是删除文本fafafafa
,它仍然将每一行作为记录读取,因此您的下一个条件完全没有意义。
myarr = ($( cat 25.6.2015_test.txt | awk -F 'fafafafa' '$1~/^[a-z0-9*]+$/ {print $1}') )
这将打印多行,这些行都将是数组中的单独元素,因为它们在换行符上分割,并且看不到 awk 中的记录是什么(如果您实际上是在记录而不是字段上分割)。
echo ${myarr[1]}
echo $i
除非您想在每次回显时查看目录中的所有文件(由于*
记录中的),否则请引用这些文件
:
为什么 ?
解决方案
# Create an array
myarr=()
# Save the number of different blocks to be saved, notice the
# `-vRS` which sets the field separator
blocks=$(awk -vRS='fafafafa' '$1~/^[a-z0-9*]+$/{x++}END{print x}' file)
# While the the counter is less than the number of blocks.
while [[ $x -le $blocks ]] ;do
# Increase the counter
((x++))
# Add the value for that block to the array, notice the quotes around
# `$()`, they are important in keeping all the block as one array
# element. The awk also increments its own counter for each
# occurrence of 'fafafafa' and your condition for '$1'. When both
# counters match the block is saved to the array.
myarr+=("$(awk -vRS='fafafafa' -vN="$x" '$1~/^[a-z0-9*]+$/{x++}
x==N{print RS$0}' test)")
done
答案2
while read -d '&' -r data
do
myarr[${#myarr[@]}]="$data"
done < <(sed '1! s/fafafafa/\&&/' 25.6.2015_test.txt)
会将文件中的所有数据放入25.6.2015_test.txt
数组中,myarr
并fafafafa
与其一起分隔开。
sed
用于在第一行之外放置定界符&
(您可以使用文本中不需要的任何字符)fafafafa
(在相反的情况下,我们收到数组的空第一个成员)。read
将由 分隔的文本部分放入&
中间变量 中data
。${#myarr[@]}
产生 array 中的元素数量myarr
。由于计数从这里开始,0
因此我们可以接收数组下一个元素的索引:
- 数组为空,元素数量为 0,因此第一个元素的索引 == 0
- 数组有 1 个索引为 0 的元素,因此元素数为 1,下一个索引 == 1
- 数组有 2 个元素,索引为 0,1,因此元素数为 2,下一个索引 == 2
- ……
答案3
线路
myarr = ($( cat 25.6.2015_test.txt | awk -F 'fafafafa' '$1~/^[a-z0-9*]+$/ {print $1}')
是错的。使用下面的行:
myarr=$(awk -F 'fafafafa' '$1~/^[a-z0-9*]+$/ {print $1}' 25.6.2015_test.txt)
你应该使用"
:
echo "${myarr[1]}"
和
echo "$i"
你可以使用这个awk
命令
和
fafafafa
:awk '{if ($1 ~ /^fafafafa$/) {line+=1; print ""; print "cell "line;print "--------"; print $1} else {print $1}}' 25.6.2015_test.txt
没有
fafafafa
awk '{if ($1 ~ /^fafafafa$/) {line+=1; print ""; print "cell "line;print "--------";} else {print $1}}' 25.6.2015_test.txt
输出示例没有fafafafa
cell 1
--------
00005e58
da1e5afe
00000000
*
cell 2
--------
00005e58
da1e5afe
00000000
*
00000001
ffffffff
555eea72
00000000
*
00000004
cell 3
--------
01da1300
*
00000004
02991c00
cell 4
--------
01da1300
cell 5
--------
01da1300
cell 6
--------
01da1300