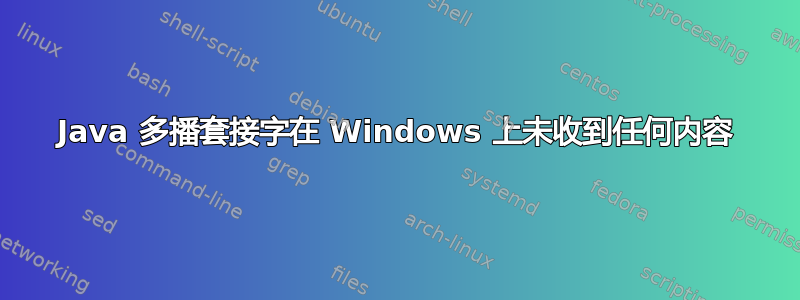
我需要以多播方式发送和接收。
我在 StackOverflow 论坛上发布了一个问题来检查我的代码:https://stackoverflow.com/questions/50131973/java-multicast-socket-doesnt-receive-anything-on-windows
我在 Ubuntu 上尝试了我的代码并且运行完美。
现在我在 Windows 10 上做实验。我使用多播套接字发送和接收 DatagramPacket。如果数据报的 InetAddress 为“230.0.0.1”进行多播,则不起作用。否则,当我使用 LAN 连接的机器的 IP 时,它可以工作。
接收者:
MulticastSocket multiSocket = new MulticastSocket(3575);
InetAddress groupMulticast = InetAddress.getByName( "230.0.0.1" );
multiSocket.joinGroup(groupMulticast);
DatagramPacket packetReceive = new DatagramPacket( bufReceive, bufReceive.length );
try {
multiSocket.receive( packetReceive );
} catch (IOException e) { e.printStackTrace(); }
发件人:
MulticastSocket multiSocket = new MulticastSocket(3575);
InetAddress groupMulticast = InetAddress.getByName( "230.0.0.1" );
multiSocket.joinGroup(groupMulticast);
byte[] bufSend = new byte[255];
DatagramPacket packetSend = new DatagramPacket( bufSend, bufSend.length,
groupMulticast, 3575 );
try {
multiSocket.send(packetSend);
} catch (IOException e) { e.printStackTrace(); }
此发送器不起作用。但如果我更改此设置:
InetAddress groupMulticast = InetAddress.getByName( "230.0.0.1" );
有了这个:
InetAddress groupMulticast = InetAddress.getByName( "192.168.0.21" );
其中 192.168.0.21 是接收器连接在 LAN 上的 IP 地址,它可以工作。
我如何进行多播发送?
答案1
尝试一下这个代码。
public class Server3 {
public static void main(String[] args) throws IOException {
MulticastSocket multiSocket = new MulticastSocket(3575);
InetAddress groupMulticast = InetAddress.getByName("224.0.0.1");
multiSocket.setBroadcast(true);
multiSocket.joinGroup(groupMulticast);
while (true) {
try {
Thread.sleep(2000);
} catch (InterruptedException e1) {
e1.printStackTrace();
}
System.out.println("Sending...");
String msg = "Hai";
byte[] bufSend = msg.getBytes();
DatagramPacket packetSend = new DatagramPacket(bufSend, bufSend.length, groupMulticast, 3575);
try {
multiSocket.send(packetSend);
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
public class Client3 {
public static void main(String[] args) throws IOException {
MulticastSocket multiSocket = new MulticastSocket(3575);
InetAddress groupMulticast = InetAddress.getByName("224.0.0.1");
multiSocket.setBroadcast(true);
multiSocket.joinGroup(groupMulticast);
byte[] bufReceive = new byte[1024];
while (true) {
try {
Thread.sleep(2000);
} catch (InterruptedException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
System.out.println("Receiving...");
DatagramPacket packetReceive = new DatagramPacket(bufReceive, bufReceive.length);
try {
multiSocket.receive(packetReceive);
System.out.println("msg...");
System.out.println(new String(bufReceive));
} catch (IOException e) {
e.printStackTrace();
}
}
}
}