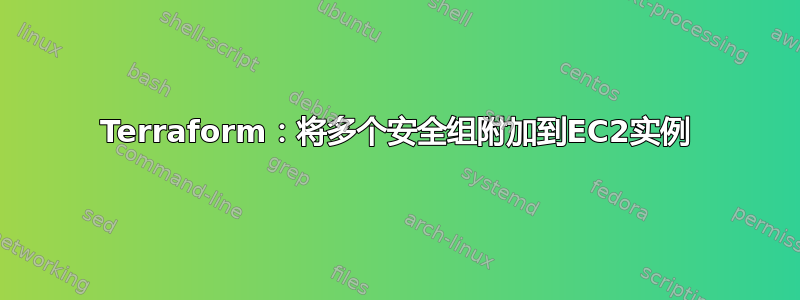
如何在创建 EC2 时附加多个安全组?我已将其模块化如下:
**networking/main.tf**
# Web Server Security Group
resource "aws_security_group" "web_sg" {
name = "web_sg"
description = "This security group will control the private Web Servers"
vpc_id = aws_vpc.perf_vpc.id
egress {
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["0.0.0.0/0"]
}
}
# Load Balancer Security Group
resource "aws_security_group" "alb_sg" {
name = "alb_sg"
description = " This secruity group is for Application Load Balancer"
vpc_id = aws_vpc.perf_vpc.id
egress {
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["0.0.0.0/0"]
}
}
resource "aws_security_group" "perf_pvt_sg" {
name = "perf_pvt_sg"
description = "Aptean_Base-Perf_Pvt"
vpc_id = aws_vpc.perf_vpc.id
depends_on = [aws_security_group.bastion_sg]
ingress {
description = "kaspersky"
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["10.176.0.35/32"]
}
egress {
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["0.0.0.0/0"]
}
}
**networking/outputs.tf**
output "perf_pvt_sg" {
value = aws_security_group.perf_pvt_sg.id
}
output "web_sg" {
value = aws_security_group.web_sg.id
}
output "alb_sg" {
value = aws_security_group.alb_sg.id
}
**root/main.tf**
# Deploy Web Servers
module "web_servers" {
source = "./web_servers"
keyname = module.domain_controllers.key_name
public_key_path = var.public_key_path
web_count = var.web_count
web_inst_type = var.web_inst_type
pvtsubnets = module.networking.private_subnets
webserver_sg = [
module.networking.web_sg,
module.networking.perf_pvt_sg,
module.networking.alb_sg
]
}
一切都很顺利,并按预期创建,terraform 输出也显示了资源,但所有 EC2 实例(在本例中,我只是指向 Web 服务器)都附加了默认安全组。我确实看到,虽然没有附加任何安全组,但创建了所有其他安全组。我还尝试将 web_sg 切换为 id 和 name 属性:
web_sg = [
module.networking.web_sg.id,
module.networking.perf_pvt_sg.id,
module.networking.alb_sg.id
]
这将引发错误(*.id 和 *.name 的错误相同):
Error: Unsupported attribute
on main.tf line 46, in module "web_servers":
46: module.networking.web_sg.name,
|----------------
| module.networking.web_sg is "sg-008001301c71877a9"
This value does not have any attributes.
Error: Unsupported attribute
on main.tf line 47, in module "web_servers":
47: module.networking.perf_pvt_sg.name,
|----------------
| module.networking.perf_pvt_sg is "sg-0a50f754aceaae6cd"
This value does not have any attributes.
Error: Unsupported attribute
on main.tf line 48, in module "web_servers":
48: module.networking.alb_sg.name
|----------------
| module.networking.alb_sg is "sg-05c898e0b6873c411"
This value does not have any attributes.
我究竟做错了什么?
编辑1: web_servers/main.tf
#Web Server
resource "aws_instance" "web" {
count = var.web_count
ami = data.aws_ami.server_ami.id
ebs_optimized = true
instance_type = var.web_inst_type
subnet_id = element(var.pvtsubnets, count.index)
credit_specification {
cpu_credits = "standard"
}
root_block_device {
volume_type = "gp2"
volume_size = 80
encrypted = true
kms_key_id = "1d9ef127-cc8f-4dda-9bdf-abdad498ea6f"
}
ebs_block_device {
device_name = "/dev/sdf"
volume_type = "gp2"
volume_size = 40
encrypted = true
kms_key_id = "1d9ef127-cc8f-4dda-9bdf-abdad498ea6f"
}
tags = {
Name = "PerformanceWeb0${count.index + 1}"
}
}
web_servers/变量.tf
variable "keyname" {}
variable "public_key_path" {}
variable "web_count" {}
variable "web_inst_type" {}
variable "pvtsubnets" {
type = list(string)
}
variable "webserver_sg" {}
答案1
已经解决了。不确定这是否是唯一的方法。当我查看代码时,它似乎因为我已经注释掉了安全组 ID在 web_servers 模块中,它采用了 VPC 默认安全组。做了以下几处更改:
web_servers/main.tf
#Web_servers
resource "aws_instance" "web" {
count = var.web_count
ami = data.aws_ami.server_ami.id
ebs_optimized = true
instance_type = var.web_inst_type
subnet_id = element(var.pvtsubnets, count.index)
vpc_security_group_ids = [
var.web_sg,
var.perf_pvt_sg,
var.alb_traffic_sg
]
web_servers/变量.tf
variable "web_sg" {}
variable "perf_pvt_sg" {}
variable "alb_traffic_sg" {}
根/main.tf
#Deploy Web Servers
module "web_servers" {
source = "./web_servers"
keyname = module.domain_controllers.key_name
public_key_path = var.public_key_path
web_count = var.web_count
web_inst_type = var.web_inst_type
pvtsubnets = module.networking.private_subnets
web_sg = module.networking.web_sg
perf_pvt_sg = module.networking.perf_pvt_sg
alb_traffic_sg = module.networking.alb_traffic_sg
}