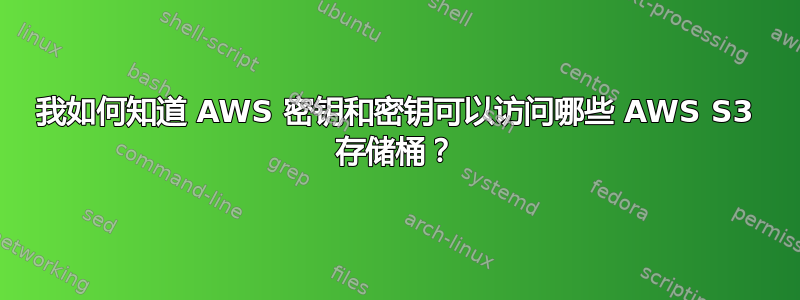
我有一个 AWS 密钥和密钥,可以访问一些 AWS S3 存储桶。我如何知道哪些存储桶?
答案1
这是一个好问题,我认为没有一个特别简单的答案。
如果你只有访问密钥和密钥,你可能需要使用 aws cli 命令,例如列表桶. 鉴于不同IAM 权限对于 S3 以及您正在寻找的确切内容,这可能是也可能不是一个可行的方法。S3 ACL 进一步增加了复杂性。
如果您也可以访问 AWS UI,假设您有足够的权限,您可以分析 IAM 策略来找出您被授予的有效权限。S3 的 IAM 访问分析器也是一种选择。
答案2
如果您需要以编程方式执行此操作,例如,如果您有很多凭证或存储桶,那么我之前编写的这段 Python 代码可能会有所帮助:
import botocore
import boto3
import os
from pathlib import Path
import configparser
#-----------------------------------------------------------------------------------------
# Check credentials and return resource & session
#-----------------------------------------------------------------------------------------
def Establish_Connection():
#-----------------------------------------------------------------------------------------
# Check credentials environment variable
#-----------------------------------------------------------------------------------------
try:
Env_Variable = os.environ['AWS_SHARED_CREDENTIALS_FILE']
except:
print("The environment variable AWS_SHARED_CREDENTIALS_FILE is not set.")
return None, None
#-----------------------------------------------------------------------------------------
# Check credentials file
#-----------------------------------------------------------------------------------------
Credential_File_Path = Path( Env_Variable )
try:
Absolute_File_Path = Credential_File_Path.resolve(strict=True)
except FileNotFoundError:
print("The file named by the environment variable,", Credential_File_Path, "cannot be resolved to an absolute path.")
return None, None
if not Absolute_File_Path.is_file():
print("The resolved file name,", Absolute_File_Path, "cannot be found.")
return None, None
#-----------------------------------------------------------------------------------------
# Check what profiles are present in the credentials file
#-----------------------------------------------------------------------------------------
Cred_Config = configparser.ConfigParser()
try:
Cred_Config.read(Absolute_File_Path)
except Exception as err:
print("Exception reading credentials file {}".format(err))
return None, None
Profile = "s3_user"
# Check the profiles present in the credentials file:
if not Cred_Config.has_section(Profile):
print("Credentials for s3_user profile not found in the configuration file.")
Profile = "default"
if not Cred_Config.has_section(Profile):
print("Credentials for default profile not found in the configuration file.")
return None, None
#-----------------------------------------------------------------------------------------
# Try to set up the AWS session and resource objects
#-----------------------------------------------------------------------------------------
try:
S3_Session = boto3.Session(profile_name=Profile)
except botocore.exceptions.ProfileNotFound :
print("Could not establish AWS session using", Profile, " credentials.")
return None, None
# Create the S3 resource object
try:
S3_Resource = S3_Session.resource('s3')
except Exception as e:
print("Can't create S3 resource object {}", e)
return None, None
# Create the S3 client object
try:
S3_Client = S3_Session.client('s3')
except Exception as e:
print("Can't create S3 client object {}", e)
return None, None
return S3_Resource, S3_Client
#-----------------------------------------------------------------------------------------
# Extract bucket information
#-----------------------------------------------------------------------------------------
def Extract_Basics(Bucket, Location_Response ):
Name = Bucket.name
Bucket_Information = {
'Name' : Name,
'Location' : Location_Response['LocationConstraint'],
'Website' : {
'isWebsite' : False,
'Index' : '',
'Error' : ''
},
'Directories' : -1,
'Files' : -1,
'Volume' : -1,
'Tags' : {},
'Storage_Class' : {},
'Owner' : {},
'File_Types' : {},
'Age_Count' : [],
'Age_Count_Size': [],
'Age_Volume' : []
}
return Bucket_Information
#====================================================================================================
# Main function
#====================================================================================================
def main():
#-----------------------------------------------------------------------------------------
# Check credentials environment variable
#-----------------------------------------------------------------------------------------
S3_Resource, S3_Client = Establish_Connection()
if S3_Resource == None:
return
#-----------------------------------------------------------------------------------------
# List buckets
#-----------------------------------------------------------------------------------------
Bucket_List = []
Bucket_Information = {}
try:
Buckets = list(S3_Resource.buckets.all())
except Exception as e:
print("Could not list buckets ", e )
return
for Bucket in Buckets:
Name = Bucket.name
Location_Response = S3_Client.get_bucket_location(Bucket=Name)
Bucket_Information = Extract_Basics( Bucket, Location_Response )
Bucket_List.append( Bucket_Information )
print("Bucket list of info")
print( Bucket_List )
return
if __name__ == '__main__':
main()