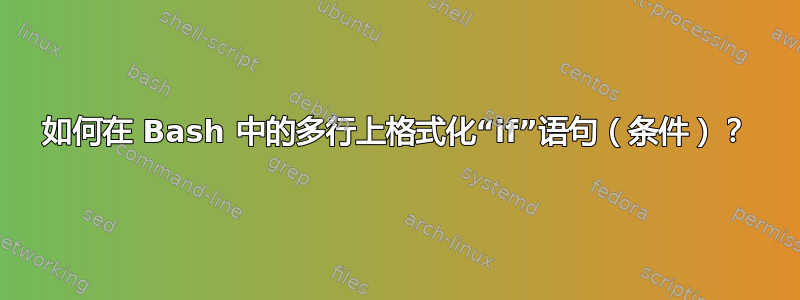
我有一个 Bash shell 函数,它接受一个参数并在需要时对其执行某些操作。
do_something() {
if [need to do something on $1]
then
do it
return 0
else
return 1
fi
}
我想用几个参数调用此方法并检查其中至少有一个是否成功。
我尝试过类似的事情:
if [ do_something "arg1" ||
do_something "arg2" ||
do_something "arg3" ]
then
echo "OK"
else
echo "NOT OK"
fi
另外,我想确保即使第一个条件为真,所有其他条件仍会被评估。
正确的语法是什么?
答案1
使用反斜杠。
if [ $(do_something "arg1") ] || \
[ $(do_something "arg2") ] || \
[ $(do_something "arg3") ]
then
echo "OK"
else
echo "NOT OK"
fi
编辑
另外 - 我想确保即使第一个条件为真,所有其他条件仍将被评估。
仅使用一个 if 语句无法实现这一点。相反,您可以使用 for 循环来迭代参数并分别评估它们。例如:
do_something() {
for x in "$@"
do
if [need to do something on $x]
then
do it
else
echo "no action required on $x"
fi
done
}
答案2
首先运行命令,然后检查其中是否至少有一个命令成功。
#!/bin/bash
success=0
do_something arg1 && success=1
do_something arg2 && success=1
do_something arg3 && success=1
if ((success)); then
printf 'Success! At least one of the three commands succeeded\n'
fi
答案3
正确的语法是:
if do_something "arg1" || \
do_something "arg2" || \
do_something "arg3"
then
echo "OK"
else
echo "NOT OK"
fi
\
用于告诉 shell 命令在下一行继续。
编辑:我认为这应该可以满足您的要求:
#!/bin/bash
do_something() {
if [need to do something on $1]
then
do it
echo "OK"
else
echo "NOT OK"
fi
}
do_something "arg1"
do_something "arg2"
do_something "arg3"
答案4
我更喜欢:
if {
do_something "arg1" ||
do_something "arg2" ||
do_something "arg3"
}; then
echo "OK"
else
echo "NOT OK"
fi