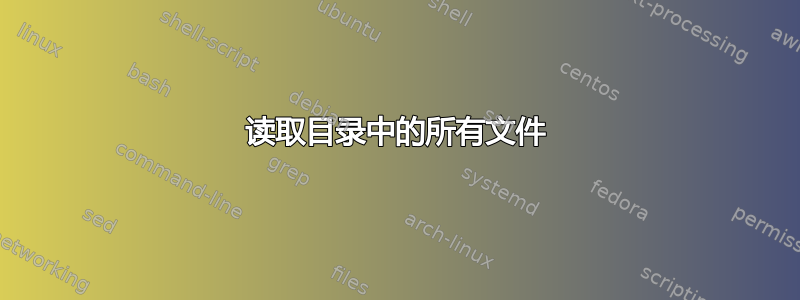
以下代码是读取下载目录中的所有文件,但是当我执行此代码时它不会打印(显示),此代码有什么问题?
import glob
path = '/home/mypc/download/*.html'
files=glob.glob(path)
for file in files:
f=open(file, 'r')
f.readlines()
f.close()
答案1
文件对象的方法readlines()
返回一个 Python 列表。它不会自动将文件内容写入 stdout:Python 是一种脚本语言,但不是 shell 脚本语言!
您应该替换:
f.readlines()
和:
sys.stdout.write(f.read())
请注意,我使用的read()
是 而不是readlines()
。正如我所说,readlines()
返回一个列表,但这里我们想要打印一个字符串——并read()
执行我们想要的操作:它读取整个文件并返回一个字符串。当文件很大时,它不是最佳选择(因为它将使用大量内存),但它可以工作。
值得注意的是,你的代码是有缺陷的。你说:以下代码是读取下载目录中的所有文件。您的代码实际上会尝试读取以 结尾的文件和目录.html
。如果您的代码找到以 结尾的目录.html
,它就会崩溃。
最后,打开文件时,您应该优先使用with
语句,尤其是打开多个文件时。该with
语句将确保您在完成操作后立即关闭文件,即使发生错误。
因此你的代码应该是这样的:
import sys
import glob
import errno
path = '/home/mypc/download/*.html'
files = glob.glob(path)
for name in files: # 'file' is a builtin type, 'name' is a less-ambiguous variable name.
try:
with open(name) as f: # No need to specify 'r': this is the default.
sys.stdout.write(f.read())
except IOError as exc:
if exc.errno != errno.EISDIR: # Do not fail if a directory is found, just ignore it.
raise # Propagate other kinds of IOError.
答案2
你可能想要使用至少一个print
。例如:
#!/usr/bin/env python
import glob
path = '/home/mypc/download/*.html'
files=glob.glob(path)
for file in files:
f=open(file, 'r')
print '%s' % f.readlines()
f.close()
#if you want to print only the filenames, use 'print file' instead of three previous lines
请阅读一些有关Python 中的输入和输出。
答案3
您只编写了读取文件的代码,而不是打印内容。如果您想打印内容,可以替换
f.readlines()
和:
for line in f:
print line
希望有所帮助。
答案4
如果你想显示文件名,你需要使用打印命令
import glob
path = '/home/mypc/download/*.html'
files=glob.glob(path)
for file in files:
print file