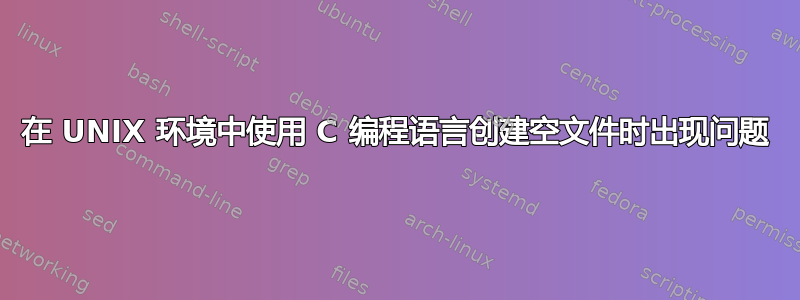
我最近开始在UNIX环境下编程。我需要编写一个程序,使用此命令创建一个空文件,其名称和大小在终端中给出
gcc foo.c -o foo.o
./foo.o result.txt 1000
这里result.txt表示新创建的文件的名称,1000表示文件的大小(以字节为单位)。
我确信查找函数移动文件偏移量,但问题是,每当我运行该程序时,它都会创建一个具有给定名称的文件,但文件的大小是0。
这是我的小程序的代码。
#include <unistd.h>
#include <stdio.h>
#include <fcntl.h>
#include <ctype.h>
#include <sys/types.h>
#include <sys/param.h>
#include <sys/stat.h>
int main(int argc, char **argv)
{
int fd;
char *file_name;
off_t bytes;
mode_t mode;
if (argc < 3)
{
perror("There is not enough command-line arguments.");
//return 1;
}
file_name = argv[1];
bytes = atoi(argv[2]);
mode = S_IWUSR | S_IWGRP | S_IWOTH;
if ((fd = creat(file_name, mode)) < 0)
{
perror("File creation error.");
//return 1;
}
if (lseek(fd, bytes, SEEK_SET) == -1)
{
perror("Lseek function error.");
//return 1;
}
close(fd);
return 0;
}
答案1
如果您要在文件末尾查找,则必须在该位置至少写入一个字节:
write(fd, "", 1);
让操作系统用零填充这个洞。
因此,如果您想使用 创建一个特定大小 1000 的空文件lseek
,请执行以下操作:
lseek(fd, 999, SEEK_SET); //<- err check
write(fd, "", 1); //<- err check
ftruncate
可能更好,而且它似乎也可以毫不费力地创建稀疏文件:
ftruncate(fd, 1000);
答案2
您没有向文件写入任何内容。
您打开文件,移动文件描述符,然后关闭它。
来自 lseek 手册页
lseek() 函数根据指令whence 将文件描述符fildes 的偏移量重新定位到参数偏移量。
答案3
从手册页来看——
The lseek() function allows the file offset to be set beyond the end of the file (but this does not change the size of the file). If
data is later written at this point, subsequent reads of the data in the gap (a "hole") return null bytes ('\0') until data is actually written into the gap.
使用truncate/ftruncate
调用来设置文件大小。