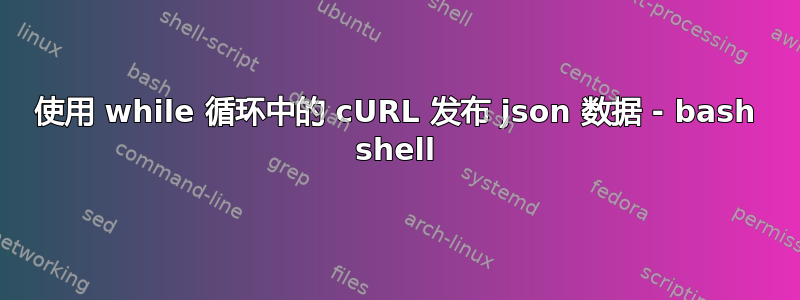
我有一个 JSON 输出,我需要从中提取 ID 并迭代它们,并向 API 发送多个请求,使用curl 执行 REST API。例如:
JSON 输出如下所示:
{
"glossary": [
{
"Title": "example glossary1",
"id": 1,
"description": "Hello Glossary1"
},
{
"Title": "example glossary2",
"id": 2,
"description": "Hello Glossary2"
},
{
"Title": "example glossary3",
"id": 3,
"description": "Hello Glossary3"
},
{
"Title": "example glossary4",
"id": 4,
"description": "Hello Glossary4"
}
]
}
shell 脚本应循环遍历此 JSON 文件,提取 ID 并循环遍历并使用 CURL 执行 REST API 调用。
这是示例:
for (( i = 0 ; i < ${#id[@]} ; i++ ))
do
POST REST API
done
答案1
如果您在名为 tmp.json 的文件中输出,请使用杰克获取 id 列表,每行一个,然后使用一个简单的 for 循环向您的 api 发布一个帖子
for i in `cat tmp.json | jq .glossary[].id`; do
curl -X POST http://host/api/$i"
done
答案2
这是一个示例,仅使用awk
:
#!/bin/bash
for id in $(awk '/"id":/ {sub(/,/, ""); print $2}' inputfile.json); do
curl -X POST ...
done
答案3
没有 awk 最多 3 位 id。只需通过 STDIN 推送 JSON 结果并使用 while 循环读取即可:
digits=0;
while read line
do
foo=`echo $line|grep id|cut -c 7-$((7+digits))`
if [[ ! $foo -eq '' ]]
then
echo "curl http://webaddress/api?id=$foo"
if [[ '$foo' == '9' ]] || [[ '$foo' == '99' ]]
then
digits=$((digits+1))
fi
fi
done<your-json-output.json
答案4
{...}
Curl 允许您通过在 URL 中使用大括号 ( ) 一次向同一端点发出多个请求。
例如,如果您的端点是
http://example.com/api/endpoint
那么您可以使用某个参数的三个值来调用它,id
如下所示:
curl 'http://example.com/api/endpoint?id={123,345,456}'
要将 ID 作为大括号中以逗号分隔的整数集形式从 JSON 文档中获取,请jq
像这样使用:
jq -r '.glossary | map(.id) | "{\(join(","))}"' file
对于问题中给定的文档,这将返回字符串{1,2,3,4}
。
然后,您可以在单次调用中使用它curl
:
ids=$( jq -r '.glossary | map(.id) | "{\(join(","))}"' file )
curl -s "http://example.com/api/endpoint?id=$ids"