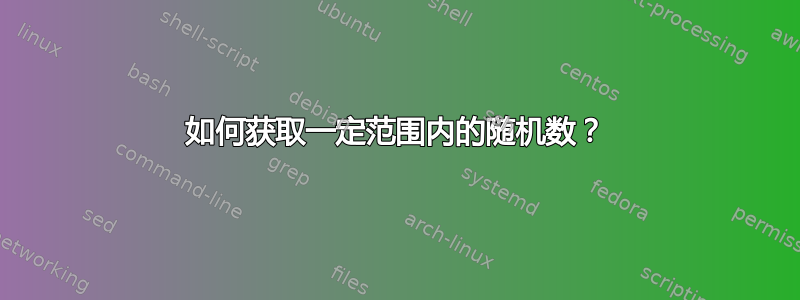
我想创建一个脚本,创建从 0 到 30,000 的随机数并将它们保存在文本文件中。
我希望所有数字都在一行中。我还希望数字在 1 到 1,000,000 之间(我的意思是文件中应该有 1 到 1,000,000 个数字)。
我该怎么做?我希望该脚本能够测试我编写的程序,并且我希望该脚本能够自动创建数字,这样我就不需要花时间自己编写它们了。
我对 Linux 脚本也一无所知,所以如果您可以在代码中添加一些注释,我会很高兴接受!:)
答案1
使用shuf
命令:
shuf -e {1..N} | tr '\n' ' ' > some-file
例如:
$ shuf -e {1..10} | tr '\n' ' '
4 9 1 7 8 6 3 5 10 2
对于非常大的数字,您可能需要使用循环for
,然后对其进行打乱:
for i in {1..1000000}
do
echo $i
done |
shuf | tr '\n' ' ' > some-file
答案2
#!/usr/bin/env python
# The line above should always be on the top of
# an executable python file. It describes the
# program this file should be interpreted with
# Import let's you extend the core functunality
# of python with code in extra "modules"
import random
# result is a string variable. Python is not strict
# about variable declaration or typing. They can
# be declared like this at any point in the programm
# and may hold a variaty of data types. This one holds
# text now.
result = ""
# The imported module random offers the function randint.
# This function generates a number, where: 1 <= n <= 1000000
loop_iterations = random.randint(1,1000000)
# counter starts of at zero, counts up and,
# on the last round, reaches loop_iterations -1
for counter in range(loop_iterations):
new_random_integer = random.randint(0,30000)
result += ", "
# We can't add a number to a string, so we need
# to convert it into a text representation first.
result += str(new_random_integer)
# A file handler let's you interact with an opened file.
# open takes a path and a setting wether you want to
# read/write. w means write.
file_handler = open('/tmp/output.txt', 'w')
file_handler.write(result)
file_handler.close()
答案3
生成特定范围内的随机数的一种非常常用的方法是取随机整数,然后计算整数除法与范围限值差的余数。基本思想是余数只能从 0 到除数,因此我们得到余数,然后将结果值偏移下限。我在用 C 编写代码时多次使用过这种技术。
当然,这个问题被标记为scripts
,并且完全可以在中实现相同的技术bash
。我们可以使用$RANDOM
变量生成器,然后使用计算器将整数取到所需范围内bc
。下面的脚本正是这样做的:
#!/usr/bin/env bash
get_random_int(){
echo "$low + $RANDOM % ( $high - $low + 1 )" | bc
}
main(){
local howmany=$1
local low=$2
local high=$3
local counter=0
while [ $counter -lt $howmany ]
do
get_random_int
counter=$(($counter+1))
done
}
main "$@"
基本用法:
$ ./random_in_range.sh 3 100 200
184
110
179
第一个参数是您想要的整数个数,第二个参数是下限,第三个参数是上限。