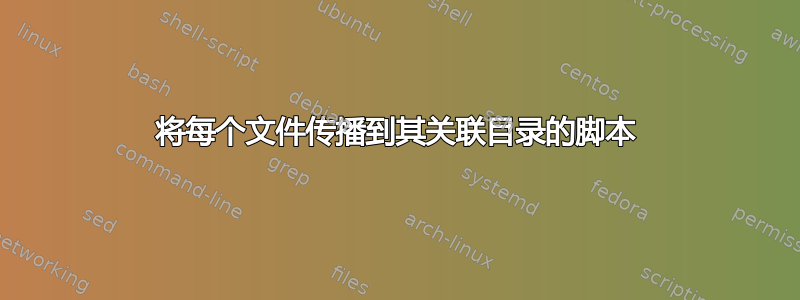
场景如下:
我有一个目录,其中包含数千张格式的图片YYYYMMDDXXX
YYYY: stands for year
MM: stands for month
DD: stands for day
XXX: stands for an ID of the picture, it ranges from 1 to n characters
需要的:
将每张图片移动到当天的文件夹中。例如,读取图片的名称(假设其名称为)201504121A
,则此图片将被移动到名为的目录中12-04-2015
,然后将其重命名201504121A
为 ID,即1A
。如果此目录未建立,则创建它。
答案1
在bash中:
#! /bin/bash
for i
do
f="${i##*/}"
y="${f:0:4}"
m="${f:4:2}"
d="${f:6:2}"
x="${f:8}"
mkdir -p "$d-$m-$y" && mv "$i" "$d-$m-$y/$x"
done
将其保存为脚本(例如mover.sh
)并执行以下操作:
/path/to/mover.sh *
或者:
find /path/to/pics -type f -exec /path/to/mover.sh {} +
使用 aecho
代替mkdir
andmv
来测试它。
f="${i##*/}"
- 获取文件名剥离目录组件。y="${f:0:4}"
,另外四个 - 获取该位置处该长度的子字符串。
或者,使用正则表达式而不是子字符串,同样可以实际测试模式:
#! /bin/bash
for i
do
f="${i##*/}"
if [[ $f =~ ([0-9]{4})([0-9]{2})([0-9]{2})(.*) ]]
then
y="${BASH_REMATCH[1]}"
m="${BASH_REMATCH[2]}"
d="${BASH_REMATCH[3]}"
x="${BASH_REMATCH[4]}"
mkdir -p "$d-$m-$y" && mv "$i" "$d-$m-$y/$x"
fi
done
答案2
您可能是一个资深的 Linux / Unix 用户。但我将考虑到您是一个像我一样的新 Linux 用户来编写说明,以便我不会遗漏我的部分内容...
警告!!!
在执行此脚本之前,请确保您已备份要运行此脚本进行操作的所有图像。有许多变量(如命名约定字数等)会影响执行结果。请确保您备份了数据。不会删除任何内容,但如果出现任何问题,脚本可能会不定期更改您的文件名。
如果任何图像包含超过 2 个字符的 ID,请不要运行此脚本。正如您之前提到的 “XXX:代表图片ID,范围是1到n个字符”
“并举了一个例子“假设它的名字是201504121A,那么这张图片将被移动到名为12-04-2015的目录中,然后将其从201504121A重命名为ID,也就是1A”
图片的 ID 应该是类似 1A 1B 1C 12 C 22 等...您明白我的意思...它应该是 2 个字符而不是一个字符,如 1 2 3 ... 或 3 个字符,如 222 666 777 等...它应该正好是 2..如果有一些这样的图像...我建议您修改我的代码以满足您的目的,并始终记得在执行脚本之前备份您的数据。
用 Bash 编写
#!/bin/bash
#Developed by Sayan Bhattacharjee Date:26th may 2015 Tuesday Time 4:46PM IST
# conatact :[email protected] or askubuntu.com Username:Sayan Bhatttacharjee
#More updates may come if required....
#Feel free to modify and redistribute this script
echo "Enter the extension of the files"
echo "like .jpg .png .bmp etc"
echo "make sure to enter . before the extension"
read extension
ls *$extension > filenames
echo "Showing all the files of the extension present in the following directory"
cat filenames
echo "Checking line read option"
## while it reads line by line it will perform the required actions
while read line
do
echo "==============================================================="
imageName=$line # updates the name of the image one by one in each loop according to how they are saved in the file
echo "Imagefile is - $name"
echo "Running file name resolver"
echo "Running year resolver"
year=${imageName:0:4 } #takes 4 lettters from the front
echo "Year is $year"
echo "Running month resolver"
abc=${imageName: (-10) } # it will extract 10 characters from the end of 201504121A.jpg to produce 04121A.jpg
month=${abc:0:2 } #It takes two characters from front of 04121A.jpg to produce 04
echo "month is $month"
echo "Running day resolver"
abc1=${imageName: (-8) } # it will extract 8 characters from the end of 201504121A.jpg to produce 121A.jpg
day=${abc1:0:2 } #It takes two characters from front of 121A.jpg to produce 12
echo "day is $day"
echo "Running ID resolver"
abc2=${imageName: (-6) } # it will extract 6 characters from the end of 201504121A.jpg to produce 1A.jpg
ID=${abc2:0:2 } #It takes two characters from front of 1A.jpg to produce 1A
echo "$ID"
echo "Present Working Directory is"
pwd > base.txt #saving present working directory
baseDirectory=`cat base.txt`
echo "$baseDirectory"
echo ".............................................................."
echo "Running Directory creation and Image Insertion"
echo "Directory will not be created if it exists"
mkdir $day-$month-$year
echo "moving image and renaming it........"
mv $baseDirectory/$year$month$day$ID$extension $baseDirectory/$day-$month-$year/$ID$extension
cd $day-$month-$year
#echo "Present Working Sub Directory is"
#pwd > subpwd.txt #saving present working directory
#presentWorkingSubDirectory=`cat subpwd.txt`
#echo "$presentWorkingSubDirectory"
echo "Returning to Base Directory"
cd $baseDirectory
echo "+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++"
done < filenames
echo "execution finished"
将文本文件中的代码块保存为dataOrganiser.sh
(或您喜欢的任何其他名称;但扩展名应为.sh
)。
确保 dataOrganiser.sh 位于所有图像所在的目录中。否则它将不起作用,您必须手动配置它...
还要注意,它一次只能处理一种扩展名的图像文件....例如,它将首先处理 .jpg,然后是 .png 和其他扩展名....扩展名的选择将在运行时提供给您..它也可以用于其他文件格式,如 .txt .doc .pdf 等
然后通过键入以下内容授予 dataOrganiser.sh 文件的可执行权限
sudo chmod 755 /path/to/dataOrganiser.sh
755 将授予您读写和执行权限,并授予其他人读写权限。您将被要求输入密码。输入密码以作为 sudo 运行。现在通过键入来执行脚本
/path/to/file/dataOrganiser.sh
脚本启动后,系统会要求您提供图片的扩展名,每次只能提供一种扩展名。扩展名应由三个字母组成,例如 jpg、bmp、png、gif 等。任何像 tiff 的东西都行不通..你必须修改脚本.....再次确保你备份了图像
当要求输入扩展名时,请将其输入为 .jpg 或 .bmp 或 .png 或 .gif,因为您需要在扩展名前加上 .(点才能起作用)。
您记得先前备份过吗?..如果你没有关闭程序备份并返回重新执行该程序.....我不想对任何人的数据造成损害
我已经给出了现在想到的所有信息。请确保你遵循上面给出的所有说明,并再次你记得备份你的照片吗