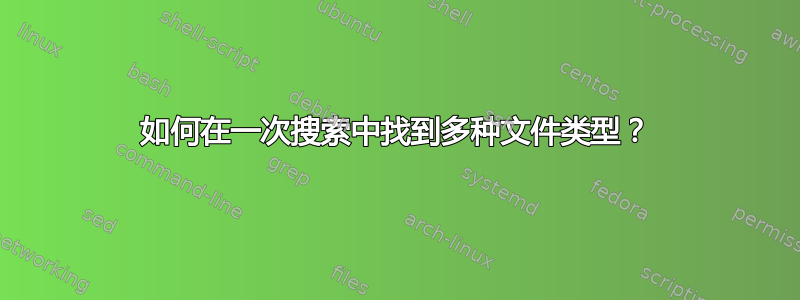
我对此进行了大量搜索,但似乎找不到满足我需求的答案。我想要一种简单的方法来搜索两种或更多文件类型(例如 *.mp3、*.aac、*.pdf 等)。我了解 Linux 命令寻找通过终端搜索来完成此操作,但我想要一个带有 GUI 的程序来满足这种特殊需求。我尝试过 Nemo 文件管理器的内置搜索,Caja、PCManFM 等也同样如此。我还尝试过鲶鱼和searchmonkey。我似乎无法获得任何富有成效的结果。非常感谢任何帮助。提前致谢。
答案1
除非您确实需要 C 的速度,否则我会推荐一种脚本语言(Ruby、Python 或类似语言)。您将能够完成复杂的任务,而无需使用 C 语言(请不要火焰)。
这就是我要做的:
使用Python-GTK界面制作GUI:您只需要4个小部件:一个Gtk.Window,一个Gtk.VBox(用于存储接下来的两项),一个用于输入文件类型的Gtk.Entry,以及一个Gtk.TreeView显示结果
在 Python 中,您可以轻松地将 Gtk.Entry 中的列表与命令
find
+ 选项 + 文件类型组合起来,以获取列表。 (您可以执行find
来自 的命令subprocess.checkcall
)。
Checkcall
返回列表,然后您可以在 Gtk.TreeView 中显示该列表。
如果您想学习 Python,网上有很多优秀的教程。这是Python 网站上的官方教程。学习Python 网站非常有趣,因为您甚至可以尝试您的程序。 本教程应该会给你提供使用 Python 中的 GUI 所需的全部内容以及大量示例。
像 Python 这样的脚本语言非常适合解决您所描述的问题。
编辑我花了一些时间,认为拥有一个紧凑的 GUI 查找器也不错。下面是一个工作代码。是的,它比20行长,主要是因为我用代码构建了整个GUI。如果我使用 Glade(GUI 设计器),代码就会减半。另外,我添加了一个对话框来选择搜索的根目录。
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
#
# test_finder.py
#
# Copyright 2016 John Coppens <[email protected]>
#
# This program is free software; you can redistribute it and/or modify
# it under the terms of the GNU General Public License as published by
# the Free Software Foundation; either version 2 of the License, or
# (at your option) any later version.
#
# This program is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU General Public License for more details.
#
# You should have received a copy of the GNU General Public License
# along with this program; if not, write to the Free Software
# Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston,
# MA 02110-1301, USA.
#
#
from gi.repository import Gtk
import os, fnmatch
class MainWindow(Gtk.Window):
def __init__(self):
super(MainWindow, self).__init__()
self.connect("destroy", lambda x: Gtk.main_quit())
self.set_size_request(600, 400)
vbox = Gtk.VBox()
# Pattern entry
self.entry = Gtk.Entry()
self.entry.connect("activate", self.can't open file 'pyfind.py'on_entry_activated)
# Initial path selection
self.path = Gtk.FileChooserButton(title = "Select start path",
action = Gtk.FileChooserAction.SELECT_FOLDER)
self.path.set_current_folder(".")
# The file list + a scroll window
self.list = self.make_file_list()
scrw = Gtk.ScrolledWindow()
scrw.add(self.list)
vbox.pack_start(self.entry, False, False, 0)
vbox.pack_start(self.path, False, False, 0)
vbox.pack_start(scrw, True, True, 0)
self.add(vbox)
self.show_all()
def make_file_list(self):
self.store = Gtk.ListStore(str, str)
filelist = Gtk.TreeView(model = self.store, enable_grid_lines = True)
renderer = Gtk.CellRendererText()
col = Gtk.TreeViewColumn("Files:", renderer, text = 0, sizing = Gtk.TreeViewColumnSizing.AUTOSIZE)
filelist.append_column(col)
col = Gtk.TreeViewColumn("Path:", renderer, text = 1)
filelist.append_column(col)
return filelist
def on_entry_activated(self, entry):
self.store.clear()
patterns = entry.get_text().split()
path = self.path.get_filename()
for root, dirs, files in os.walk(path):
for name in files:
for pat in patterns:
if fnmatch.fnmatch(name, pat):
self.store.append((name, root))
def run(self):
Gtk.main()
def main(args):
mainwdw = MainWindow()
mainwdw.run()
return 0
if __name__ == '__main__':
import sys
sys.exit(main(sys.argv))
手动的:
- 检查您是否安装了 Python3 (
python -V
)。如果没有,请安装它。 - 将上面的程序另存为
pyfind.py
- 使其可执行
chmod 755 pyfind.py
- 执行它
./pyfind.py
- 输入过滤器,然后按 Enter。
- 如果更改路径,请重复 1(如果需要,可以通过使用来自 FileChooserButton 的信号来避免这种情况)
编辑2
我不确定你的情况发生了什么,但日志显示 python3 找不到 pyfind.py 文件。您可以使该文件直接可执行:
将第一行代码修改为:
#!/usr/local/bin/python3
要使上述文件可执行,请执行以下操作(在终端窗口中,在 pyfind.py 所在的目录中):
chmod 755 pyfind.py
然后您应该能够像这样简单地执行程序(同样,在终端中):
./pyfind.py