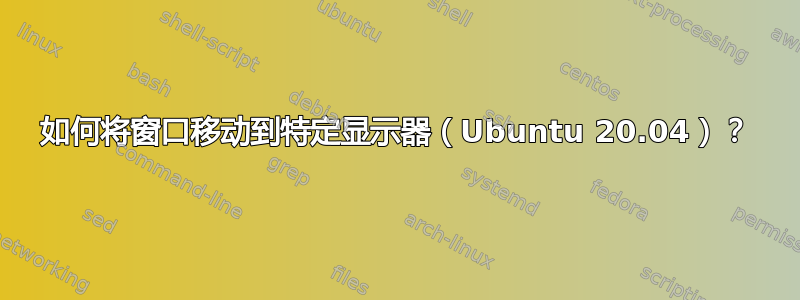
如何使用代码将窗口移动到特定显示器?我有两个屏幕,一个需要全屏运行应用程序,另一个需要全屏播放视频。
答案1
您可以使用工具来实现你想要的。
我认为没有任何设置可以移动到一个屏幕或另一个屏幕,您只需要设置每个窗口的位置(x 和 y 位置)和几何形状(大小)。例如,在终端窗口中运行以下命令
xdotool search --name "WINDOW TITLE HERE" getwindowgeometry
然后在不同的显示器之间移动窗口,您将看到它只是一个不同的(左上角)x/y 位置,具体取决于您的显示器设置和分辨率。
因此,您可以进行实验并编写脚本来获取相关的窗口 ID,然后使用命令设置位置windowmove
并使用命令设置几何windowsize
。
答案2
我创建这python 脚本读取配置 json 文件并将每个窗口放置到所需位置。
sudo apt install xdotool
git clone [email protected]:cix-code/win-pos.git
cd win-pos
cp sample.config.json config.json
nano config.json
# Run it this way or set it as delayed startup script
python3 winpos.py
配置示例:
// Postitions the Spotify window on the second screen from the left,
// on the second desktop centerly aligned.
{
"name": "Spotify",
"search_name": "^Spotify$",
"screen": 1,
"desktop": 1,
"width": "50%",
"height": "60%",
"align": "center center"
},
该脚本只是运行相关的xrandr
、xdotool
和pgrep
命令来检索屏幕布局、获取窗口 ID 并将窗口放置到所需的位置。
答案3
我创建了这个相当复杂的代码来找到我想要移动到特定屏幕的窗口,该屏幕由显示器的宽度(毫米)决定(它还将调整为正确的分辨率并将应用程序设置为全屏):
#!/usr/bin/env python3
import subprocess
import time
def get(cmd): return subprocess.check_output(cmd).decode('utf-8')
def adjust_resolution(name, res): # name = DP-2, res: 1920x1080
get(['xrandr', '--output', name, '--mode', res])
def edit_window(monitor, window_name, function):
print('moving', window_name, 'to', monitor)
get(['wmctrl', '-F', '-r', window_name, '-e', '100,' +
monitor['x'] + ',' + monitor['y'] + ',600,600'])
get(['wmctrl', '-F', '-r', window_name, '-b', function])
def get_monitors():
screendata = [l.split() for l in get(['xrandr', '--current']
).replace('primary', '').splitlines() if ' connected' in l]
monitors = []
for i in range(len(screendata)):
monitor = dict()
[size, x, y] = screendata[i][2].split('+')
monitor = {
'name': screendata[i][0],
'width': screendata[i][-3],
'height': screendata[i][-1],
'size': size,
'x': x,
'y': y
}
monitors.append(monitor)
return monitors
def find_element_where(elements, key, value):
return next((item for item in elements if item[key] == value), None)
def window_info_to_dict(info):
_, _, x, y, width, height, _, *name = info.split()
return {'x': x, 'y': y, 'width': width, 'height': height, 'name': ' '.join(name)}
def get_window_info():
return list(map(window_info_to_dict, get(['wmctrl', '-l', '-G']).splitlines()))
# Constants:
main_monitor_width = '256mm'
controller_app = 'name_of_app'
video_player = 'VLC media player'
correct_resolution = '1920x1080'
# Variables:
did_remove_fullscreen = False
while True:
try:
# Get monitors:
monitors = get_monitors()
main_monitor = next(
(monitor for monitor in monitors if monitor['width'] == main_monitor_width), None)
secondary_monitor = next(
(monitor for monitor in monitors if monitor['width'] != main_monitor_width), None)
# print(main_monitor)
# print(secondary_monitor)
# Get windows:
window_info = get_window_info()
controller_app_window_info = find_element_where(
window_info, 'name', controller_app)
video_player_window_info = find_element_where(
window_info, 'name', video_player)
# Check if secondary monitor is the right size:
if secondary_monitor and secondary_monitor['size'] != correct_resolution:
print('Wrong resolution', secondary_monitor)
adjust_resolution(secondary_monitor['name'], correct_resolution)
# print(controller_app_window_info)
# print(video_player_window_info)
if main_monitor:
if controller_app_window_info['x'] != main_monitor['x'] or controller_app_window_info['y'] != main_monitor['y'] or controller_app_window_info['width'] != '1920' or controller_app_window_info['height'] != '1080':
print('Controller app is not positioned correctly')
edit_window(main_monitor, controller_app, 'add,fullscreen')
else:
print('No primary monitor')
if secondary_monitor:
if video_player_window_info['x'] != secondary_monitor['x'] or video_player_window_info['y'] != secondary_monitor['y'] or video_player_window_info['width'] != '1920' or video_player_window_info['height'] != '1080':
print('Video player is not positioned correctly')
edit_window(secondary_monitor, video_player, 'add,fullscreen')
did_remove_fullscreen = False
else:
print('No secondary monitor')
if main_monitor and not did_remove_fullscreen:
edit_window(main_monitor, video_player, 'remove,fullscreen')
did_remove_fullscreen = True
except Exception as e:
print('An error occured', e)
time.sleep(10)