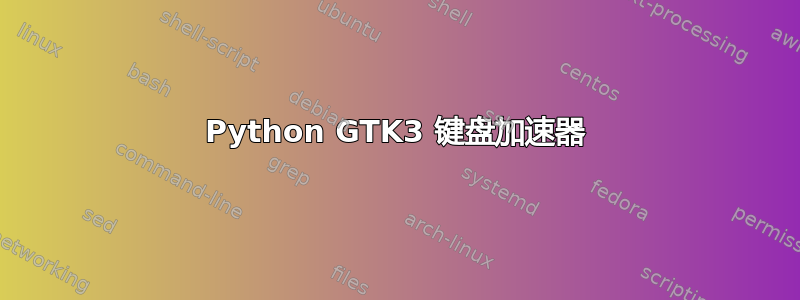
我正在使用 Python3 和 Gtk3 为 Ubuntu 创建一个应用程序。
我正在尝试向程序中添加键盘加速器,但不幸的是,我不断遇到问题。
这是我的 atm 代码:
#! /bin/python
from gi.repository import Gtk, Gdk
class DeSedit(Gtk.Window):
def __init__(self):
Gtk.Window.__init__(self, title="DeSedit")
self.set_default_size(550, 350)
# FileChooserDialog - Open File
dialog = Gtk.FileChooserDialog("Select file to be opened", self,
Gtk.FileChooserAction.OPEN,
(Gtk.STOCK_CANCEL, Gtk.ResponseType.CANCEL,
Gtk.STOCK_OK, Gtk.ResponseType.OK))
# keyboard shortcuts
accel = Gtk.AccelGroup()
self.add_accel_group(accel)
self.add_accelerator(dialog, "<Control>o", signal="open")
def add_accelerator(self, widget, accelerator, signal="activate"):
""" adds <Control>o as accelerator for open-file dialog """
if accelerator is not None:
key, mod = Gtk.accelerator_parse(accelerator)
widget.add_accelelator(signal, accel, key, mod, Gtk.AccelFlags.VISIBLE)
print("works")
# grid to organize widgets
self.box = Gtk.Box()
self.add(self.box)
# text view
self.textview = Gtk.TextView()
self.textview.set_wrap_mode(True)
# scroll bar
scrollwindow = Gtk.ScrolledWindow()
scrollwindow.add(self.textview)
self.box.pack_start(scrollwindow, True, True, 0)
window = DeSedit() # create DeSedit object
window.connect("delete-event", Gtk.main_quit)
window.show_all()
Gtk.main()
我收到此错误:
文件“file.py”,第 41 行,位于 window = DeSedit() # 创建 DeSedit 对象 文件“file.py”,第 19 行,位于 __init__ 中 self.add_accelerator(self.dialog,“o”,信号=“open”) TypeError:add_accelerator()得到了一个意外的关键字参数“signal”
答案1
最有可能的是,您关注的是 PyGtk2 指导尝试实现这一点时:根据 GTK 3 文档,没有名为 的函数gtk_window_add_accelerator
,但有一个名为 的函数gtk_accel_group_connect
看起来可以完成这项工作。使用 GTK 文档时,通常 80% 依靠直觉,20% 依靠检查文档以查看是否正确。不要期待任何花哨的教程或指南(大多数时候你会失望)……
无论如何:看看gtk_accel_group_connect
文档中,我们看到这些参数:accel_group
,,,和。accel_key
accel_mods
accel_flags
closure
那么如何从 Python 调用此函数?让我们一步一步来:
- 因为我们使用的是 Python(面向对象语言),所以我们可以预期该
accel_group
参数是隐式的,因为它是第一个参数,也是对我们尝试修改的对象的引用。 - 下一个参数(
accel_key
)更棘手:它是一个整数,文档只是告诉我们加速器键值这基本上意味着自己弄清楚如何找到加速键值。幸运的是,一些快速搜索揭示了该功能gdk_keyval_from_name
这似乎满足了我们的要求。(调用Gdk.keyval_from_name('O')
实际上返回了正确的结果。) - 接下来是参数
accel_mods
:通过单击其类型(GdkModifierType
)我们得到了所有可能的修饰符类型的列表。由于您想使用修饰符,因此<Control>
我们只需使用Gdk.ModifierType.CONTROL_MASK
并继续即可。 accel_flags
还有可点击的类型(GtkAccelFlags
) 打开其描述后发现这些参数都不是必需的,因此我们可以安全地使用0
该参数。- 最后我们来到了最后一个参数(
closure
):由于closure
它只是回调的一个花哨词(当某些事情发生时应该调用的东西),我们可以利用 Python GTK 绑定为我们抽象闭包的事实,并在这里传递一些可调用函数,只要<Control>O
按下它就会被调用。
在上下文中,代码现在看起来像这样(已验证可以正常工作):
#!/usr/bin/python3
from gi.repository import Gtk, Gdk
class DeSedit(Gtk.Window):
def __init__(self):
Gtk.Window.__init__(self, title="DeSedit")
self.set_default_size(550, 350)
# FileChooserDialog - Open File
dialog = Gtk.FileChooserDialog("Select file to be opened", self,
Gtk.FileChooserAction.OPEN,
(Gtk.STOCK_CANCEL, Gtk.ResponseType.CANCEL,
Gtk.STOCK_OK, Gtk.ResponseType.OK))
# keyboard shortcuts
accel = Gtk.AccelGroup()
accel.connect(Gdk.keyval_from_name('O'), Gdk.ModifierType.CONTROL_MASK, 0, self.on_accel_pressed)
self.add_accel_group(accel)
# grid to organize widgets
self.box = Gtk.Box()
self.add(self.box)
# text view
self.textview = Gtk.TextView()
self.textview.set_wrap_mode(True)
# scroll bar
scrollwindow = Gtk.ScrolledWindow()
scrollwindow.add(self.textview)
self.box.pack_start(scrollwindow, True, True, 0)
def on_accel_pressed(self, *args):
dialog = Gtk.FileChooserDialog("Select file to be opened", self,
Gtk.FileChooserAction.OPEN,
(Gtk.STOCK_CANCEL, Gtk.ResponseType.CANCEL,
Gtk.STOCK_OK, Gtk.ResponseType.OK))
dialog.show()
window = DeSedit() # create DeSedit object
window.connect("delete-event", Gtk.main_quit)
希望这能帮助您开始使用 GTK+3!