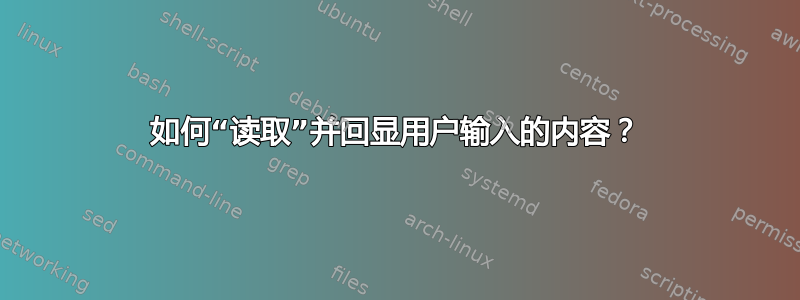
我正在编写这个脚本:当你输入
"my name is [your name]"
它告诉你
"hi, [your name]".
但是,我不知道如何制作[your name]
您输入的任何内容。我制作了一个具有特定名称的脚本,但我希望它能够回显用户输入的任何名称:
#!/bin/bash
read -p "Say something: " sth
if [[ $sth = "my name is ralph" ]]
then
echo "Hi $(echo $sth | cut -f 4 -d ' ')"
else
echo "I didn't understand that"
fi
因此这将会回显Hi ralph
,但如何让它回显Hi [your name]
您输入的任何名称?
答案1
你没有给出具体细节,但一般来说你捕捉到了姓名像这样。
#!/bin/bash
regex='[Mm]y(\ name\ is\ )(\w*)'
read -p "Say something: " response
echo $response
if [[ "$response" =~ $regex ]]; then
name=$(echo $response | cut -d' ' -f4)
echo "Hi $name"
else
echo "I didn't understand that"
fi
答案2
您可以使用正则表达式测试=~
来捕获之后的内容my name is
:
$ read -rp "Say something: "; if [[ "$REPLY" =~ [Mm]y\ name\ is\ .* ]]; then echo "Hi "${REPLY:11}"" ; fi
Say something: my name is zanna
Hi zanna
在这里我用了一个参数扩展删除前 11 个字符(my name is
)并打印其后的内容,但如果用户输入的内容不止他们的名字,结果可能不是您想要的:
Say something: my name is pixie and I eat flowers
Hi pixie and I eat flowers
乔治的回答使用仅打印第 4 个字段来处理此问题cut
(但我猜用户可能会输入My name is Super Rainbow Unicorn
并且您可能不希望 shell 仅回复Hi Super
)。
更具可读性,并带有else
:
read -rp "Say something: "
if [[ "$REPLY" =~ [Mm]y\ name\ is\ .* ]]
then
echo "Hi "${REPLY:11}""
else
echo "I didn't understand that."
fi
答案3
Zanna 和 George 都正确地使用了正则表达式,但实际上并没有使用 Bash 的正则表达式支持来提取姓名。例如:
regex='[Mm]y(\ name\ is\ )(\w*)'
read -p "Say something: " response
if [[ "$response" =~ $regex ]]; then
正则表达式测试完成后[[ ]]
,bash 会提供数组中匹配的正则表达式组BASH_REMATCH
。例如(输入My name is foo bar baz
):
$ printf "%s\n" "${BASH_REMATCH[@]}"
My name is foo
name is
foo
因此,稍微修改一下组:
$ regex='My name is (\w.*)'
$ [[ "$response" =~ $regex ]]
$ printf "%s\n" "${BASH_REMATCH[@]}"
My name is foo bar baz
foo bar baz
更好的是,你可以告诉 bash 使用不区分大小写的正则表达式匹配:
shopt -s nocasematch
综合以上所有:
regex='My name is (\w.*)'
read -rp "Say something: "
shopt -s nocasematch
if [[ $REPLY =~ $regex ]]
then
echo "Hi ${BASH_REMATCH[1]}"
else
echo "I didn't understand that."
fi
(此外,这有助于很容易地提取名字的第一个单词或最后一个单词。)
答案4
从其他人的回复中得到这个,然后我自己做了一些修改。这只是一个简单的东西,你可以轻松地将其变成命令。没有对格式的解释,它不会防止无效输入等。
read -p username: username ; curl -s "https://api.github.com/users/$username/repos?per_page=100" | grep -o 'git@[^"]*'