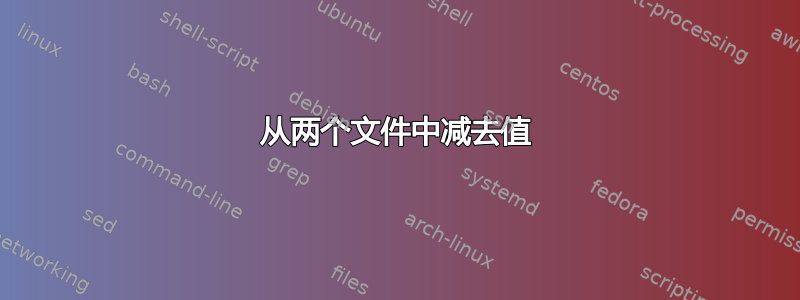
假设我有两个如下所示的文件,我想从两个文件中减去值:-
文件 1 -
emcas_bdl_migrate=2
emcas_biaas_dev=691
emcas_brs_ba=462
emcas_cc_analytics=1985
emcas_clm_reporting=0
emcas_collab_xsat=3659
emcas_cpsd_cee=10
emcas_cpsd_hcp=0
emcas_e2ep_ba=81
emcas_edservices_bi=643
和文件 2 -
emcas_bdl_migrate=2
emcas_biaas_dev=63
emcas_brs_ba=430
emcas_cc_analytics=2148
emcas_clm_reporting=16
emcas_collab_xsat=4082
emcas_cpsd_cee=11
emcas_cpsd_hcp=0
emcas_cs_logistics=0
emcas_e2ep_ba=195
emcas_edservices_bi=1059
文件 2 可以有额外的值(例如,emcas_cs_logistics=0
在第二个文件额外中)。它将合并到最终的输出文件中。
想要所需的输出作为文件 3 -
emcas_bdl_migrate=0
emcas_biaas_dev=-628
emcas_brs_ba=-32
emcas_cc_analytics=163
emcas_clm_reporting=16
emcas_collab_xsat=423
emcas_cpsd_cee=11
emcas_cpsd_hcp=0
emcas_cs_logistics=0
emcas_e2ep_ba=-114
emcas_edservices_bi=416
答案1
您可以awk
为此使用:
awk -F= 'NR==FNR{a[$1]=$2;next}{printf "%s=%s\n",$1,$2-a[$1]}' file1 file2
答案2
也试试
awk -F= -vOFS="=" 'NR==FNR {T[$1] = $2; next} {$2 -= T[$1]} 1' file[12]
emcas_bdl_migrate=0
emcas_biaas_dev=-628
emcas_brs_ba=-32
emcas_cc_analytics=163
emcas_clm_reporting=16
emcas_collab_xsat=423
emcas_cpsd_cee=1
emcas_cpsd_hcp=0
emcas_cs_logistics=0
emcas_e2ep_ba=114
emcas_edservices_bi=416
这会在 T 数组中收集 file1 的值 $2,该数组由 $1 中的标记进行索引。然后,读取 file2,在打印之前从 file2 的值中减去相应的 T 元素(如果不存在则为 0)。结果与您期望的输出有两个偏差;您可能需要仔细检查是否有拼写错误。
答案3
一种方法是使用关联数组。这个这个:
#!/bin/bash
# Declare a to be an associative array
declare -A a
# Read in file2, populating the associative array
while read l; do
k=${l%=*}
v=${l#*=}
a[${k}]=${v}
done <file2.txt
# Read in file1. Subtract the value for a key in file1
# from what was populated into the array from file2.
while read l; do
k=${l%=*}
v=${l#*=}
(( a[${k}] -= ${v} ))
done <file1.txt
# Print out the resulting associative array.
for k in ${!a[@]}; do
echo "${k}: ${a[${k}]}"
done
exit 0
答案4
这是使用 Python 的方法。请注意,这是一个经典案例专柜收藏
#!/usr/bin/env python3
import argparse
from collections import Counter
def read(f):
"""Reads the contents of param=value file and returns a dict"""
d = {}
with open(f,'r') as fin:
for item in fin.readlines():
s = item.split('=')
d[s[0]] = int(s[1])
return d
def write(f, d):
"""Writes dict d to param=value file 'f'"""
with open(f, 'w') as fout:
for k,v in sorted(d.items()):
fout.write('{}={}\n'.format(k,v))
def subtract(d1, d2):
"""
Subtracts values in d2 from d1 for all params and returns the result in result.
If an item is present in one of the inputs and not the other, it is added to
result.
Note: order of arguments plays a role in the sign of the result.
"""
c = Counter(d1.copy())
c.subtract(d2)
return c
if __name__ == "__main__":
parser = argparse.ArgumentParser()
parser.add_argument('file1', type=str, help='path to file 1')
parser.add_argument('file2', type=str, help='path to file 2')
parser.add_argument('outfile', type=str, help='path to output file')
args = parser.parse_args()
d1 = read(args.file1)
d2 = read(args.file2)
d3 = subtract(d1,d2)
write(args.outfile, d3)
将上面的代码保存到一个名为的文件中subtract_params
,并添加可执行权限。然后可以这样调用它:
subtract_params file1.txt file2.txt result.txt