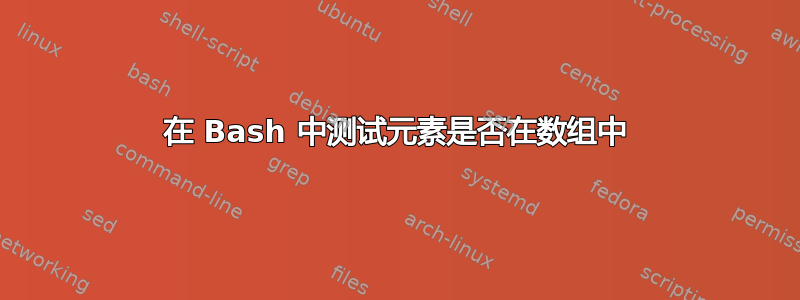
有没有一种好的方法来检查 bash 中一个数组是否有一个元素(比循环更好)?
或者,是否有另一种方法来检查数字或字符串是否等于一组预定义常量中的任何一个?
答案1
在 Bash 4 中,可以使用关联数组:
# set up array of constants
declare -A array
for constant in foo bar baz
do
array[$constant]=1
done
# test for existence
test1="bar"
test2="xyzzy"
if [[ ${array[$test1]} ]]; then echo "Exists"; fi # Exists
if [[ ${array[$test2]} ]]; then echo "Exists"; fi # doesn't
要最初设置数组,您也可以进行直接分配:
array[foo]=1
array[bar]=1
# etc.
或者这样:
array=([foo]=1 [bar]=1 [baz]=1)
答案2
这是一个老问题,但我认为最简单的解决方案尚未出现:test ${array[key]+_}
。例如:
declare -A xs=([a]=1 [b]="")
test ${xs[a]+_} && echo "a is set"
test ${xs[b]+_} && echo "b is set"
test ${xs[c]+_} && echo "c is set"
输出:
a is set
b is set
要了解这项工作如何进行,请检查这。
答案3
有一种方法可以测试关联数组的元素是否存在(未设置),这与空不同:
isNotSet() {
if [[ ! ${!1} && ${!1-_} ]]
then
return 1
fi
}
然后使用它:
declare -A assoc
KEY="key"
isNotSet assoc[${KEY}]
if [ $? -ne 0 ]
then
echo "${KEY} is not set."
fi
答案4
#!/bin/bash
function in_array {
ARRAY=$2
for e in ${ARRAY[*]}
do
if [[ "$e" == "$1" ]]
then
return 0
fi
done
return 1
}
my_array=(Drupal Wordpress Joomla)
if in_array "Drupal" "${my_array[*]}"
then
echo "Found"
else
echo "Not found"
fi