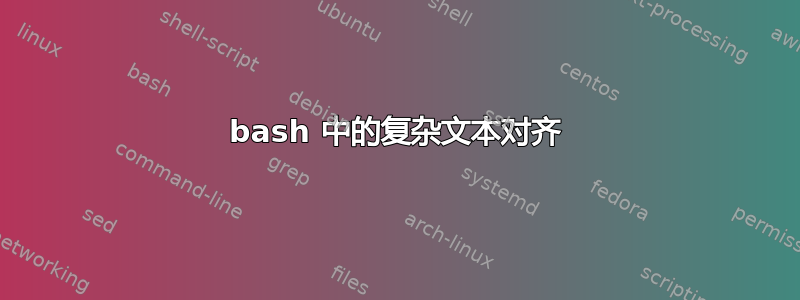
鉴于此输入:
# Lines starting with # stay the same
# Empty lines stay the same
# only lines with comments should change
ls # show all major directories
# and other things
cd # The cd command - change directory
# will allow the user to change between file directories
touch # The touch command, the make file command
# allows users to make files using the Linux CLI # example, cd ~
bar foo baz # foo foo foo
我需要保留以 开头的行#
和不包含任何注释的行,但将所有其他注释对齐在同一列上。
期望的输出:
# Lines starting with # stay the same
# Empty lines stay the same
# Only lines with # in middle should change and be aligned
ls # show all major directories
# and other things
cd # The cd command - change directory
# will allow the user to change between file directories
touch # The touch command, the make file command
# allows users to make files using the Linux CLI # exmaple, cd ~
bar foo baz # foo foo foo
到目前为止我所拥有的:
# Building an array out of input
while IFS=$'\n' read -r; do
lines+=("$REPLY")
done
# Looping through array and selecting elemnts that need change
for i in "${lines[@]}"
do
if [[ ${i:0:1} == ';' || $i != *";"* ]];
then
echo "DOESNT CHANGE: #### $i"
else
echo "HAS TO CHANGE: #### $i"
array+=( "${i%%";"*}" );
array2+=("${i##";"}")
fi
done
# Trying to find the longest line to decide how much space I need to add for each element
max = ${array[0]}
for n in "${array[@]}" ; do
((${#n} > max)) && max=${#n}
echo "Length:" ${#n} ${n}
done
#Longest line
echo $max
# Loop for populating array
for j in "${!array2[@]}" ; do
echo "${array2[j]} " | sed -e "s/;/$(echo "-%20s ;") /g"
done
我感觉我做得太多了。我认为应该有一种更简单的方法来解决这个问题。
答案1
如果您的所有命令和参数都不包含#
, 和另一个字符(例如字节 1 给出的 ASCII 字符),您可以插入该其他字符作为额外的分隔符并用于column
对齐注释(请参阅这个答案)。所以,像这样:
$ sed $'s/#/\001#/' input-file | column -ets $'\001'
# Lines starting with # stay the same
# Empty lines stay the same
# only lines with comments should change
ls # show all major directories
# and other things
cd # The cd command - change directory
# will allow the user to change between file directories
touch # The touch command, the make file command
# allows users to make files using the Linux CLI # example, cd ~
bar foo baz # foo foo foo
如果您column
不支持-e
避免消除空行,您可以向空行添加一些内容(例如,空格或上面使用的分隔符):
$ sed $'s/#/\001#/;s/^$/\001/' input-file | column -ts $'\001'
# Lines starting with # stay the same
# Empty lines stay the same
# only lines with comments should change
ls # show all major directories
# and other things
cd # The cd command - change directory
# will allow the user to change between file directories
touch # The touch command, the make file command
# allows users to make files using the Linux CLI # example, cd ~
bar foo baz # foo foo foo
答案2
单独使用 shell 进行文本处理有点尴尬,并且可能容易出错(请参阅“为什么使用 shell 循环处理文本被认为是不好的做法?")。通常最好使用其他编程语言来完成此类任务。
perl -ne 'if (/^([^#]+?)\s*#(.*)$/) { printf("%-16s#%s\n", $1, $2) } else { print }' file
这使用 Perl 捕获 前面的位#
(丢弃最后一个字和 之间的空格#
)和后面的位。如果匹配成功,它会为文本分配 16 个字符位置并打印格式化文本和注释。如果匹配不成功(因为该行为空或以 开头#
),则不加修改地打印该行。
# Lines starting with # stay the same
# Empty lines stay the same
# only lines with comments should change
ls # show all major directories
# and other things
cd # The cd command - change directory
# will allow the user to change between file directories
touch # The touch command, the make file command
# allows users to make files using the Linux CLI # example, cd ~
bar foo baz # foo foo foo
答案3
这是一个可以执行您想要的操作的 Python 脚本:
#!/usr/bin/env python
# -*- encoding: ascii -*-
"""align.py"""
import re
import sys
# Read the data from the file into a list
lines = []
with open(sys.argv[1], 'r') as textfile:
lines = textfile.readlines()
# Iterate through the data once to get the maximum indentation
max_indentation = 0
comment_block = False
for line in lines:
# Check for the end of a comment block
if comment_block:
if not re.match(r'^\s*#.*$', line):
comment_block = False
# Check for the beginning of a comment block
else:
if re.match(r'^[^#]*[^ #].*#.*$', line):
comment_block = True
indentation = line.index('#')
max_indentation = max(max_indentation, indentation)
# Iterate through the data a second time and output the reformatted text
comment_block = False
for line in lines:
if comment_block:
if re.match(r'^\s*#.*$', line):
line = ' ' * max_indentation + line.lstrip()
else:
comment_block = False
else:
if re.match(r'^[^#]*[^ #].*#.*$', line):
pre, sep, suf = line.partition('#')
line = pre.ljust(max_indentation) + sep + suf
comment_block = True
sys.stdout.write(line)
像这样运行它:
python align.py input.txt
它产生以下输出:
# Lines starting with # stay the same
# Empty lines stay the same
# only lines with comments should change
ls # show all major directories
# and other things
cd # The cd command - change directory
# will allow the user to change between file directories
touch # The touch command, the make file command
# allows users to make files using the Linux CLI # example, cd ~
bar foo baz # foo foo foo