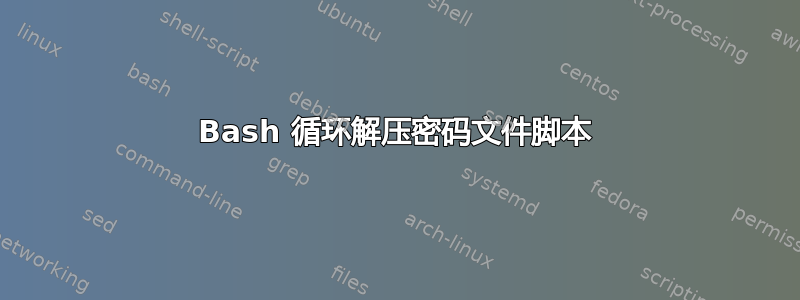
我正在尝试制作一个脚本来解压缩受密码保护的文件,密码是解压缩时得到的文件的名称
例如。
- file1.zip包含file2.zip,其密码为file2。
- file2.zip包含file3.zip,其密码为file3
如何解压file1.zip
并读取名称file2.zip
以便将其输入到脚本中?
以下是命令行的屏幕截图:
root@kaliVM:~/Desktop# unzip 49805.zip Archive: 49805.zip [49805.zip] 13811.zip password:
我只需要 Bash 读取该输出即可知道新密码(在本例中密码是 13811)。
这是我到目前为止所做的
#!/bin/bash
echo First zip name:
read firstfile
pw=$(zipinfo -1 $firstfile | cut -d. -f1)
nextfile=$(zipinfo -1 $firstfile)
unzip -P $pw $firstfile
rm $firstfile
nextfile=$firstfile
现在我怎样才能让它循环呢?
答案1
如果您没有并且zipinfo
由于任何原因无法安装,您可以通过使用unzip
with-Z
选项来模仿它。要列出 zip 的内容,请使用unzip -Z1
:
pw="$(unzip -Z1 file1.zip | cut -f1 -d'.')"
unzip -P "$pw" file1.zip
将其放入循环中:
zipfile="file1.zip"
while unzip -Z1 "$zipfile" | head -n1 | grep "\.zip$"; do
next_zipfile="$(unzip -Z1 "$zipfile" | head -n1)"
unzip -P "${next_zipfile%.*}" "$zipfile"
zipfile="$next_zipfile"
done
或递归函数:
unzip_all() {
zipfile="$1"
next_zipfile="$(unzip -Z1 "$zipfile" | head -n1)"
if echo "$next_zipfile" | grep "\.zip$"; then
unzip -P "${next_zipfile%%.*}" "$zipfile"
unzip_all "$next_zipfile"
fi
}
unzip_all "file1.zip"
-Z zipinfo(1) 模式。如果命令行上的第一个选项是 -Z,则其余选项将被视为 zipinfo(1) 选项。有关这些选项的说明,请参阅相应的手册页。
-1:仅列出文件名,每行一个。此选项排除所有其他选项;标题、预告片和 zip 文件注释永远不会被打印。它旨在用于 Unix shell 脚本。
答案2
问zipinfo
获取 zip 文件中列出的文件名,然后捕获它作为密码。使用该密码解压缩文件:
pw=$(zipinfo -1 file1.zip | cut -d. -f1)
unzip -P "$pw" file1.zip
请注意,该标志为zipinfo
是一不是一个埃尔。
慷慨地借钱吉尔斯对类似问题的回答,这是一个 bash 循环,它将提取受密码保护的嵌套 zip 文件,直到不再有 zip 文件:
shopt -s nullglob
while set -- *.zip; [ $# -eq 1 ]
do
unzippw "$1" && rm -- "$1"
done
我将函数定义unzippw
为上面的zipinfo
和unzip
命令的包装器:
unzippw ()
{
local pw=$(zipinfo -1 "$1" | cut -d. -f1)
unzip -P "$pw" "$1"
}