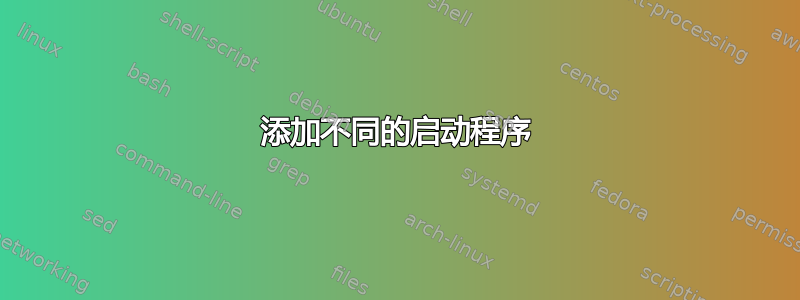
我该如何在计算机上添加一个完全不同的启动程序?会是这样的吗?
My.Computer.Registry.LocalMachine.OpenSubKey("SOFTWARE\Microsoft\Windows\CurrentVersion\Run", True).SetValue(Application.ProductName, "C:\APPLICATIONSPATH.fileextension")
PS:抱歉,我是菜鸟。
答案1
有多种方法可以添加启动程序。
首先,您使用的是什么操作系统?从外观上看,是 Windows 版本吗?
你想使用什么编程语言?C Sharp?
假设您有权限写入注册表,那么您写的内容看起来基本正确。您尝试过测试吗?还有一个 RunOnce 子项,允许程序仅在下次启动时运行。我之前确实做过您想做的事情,但是我目前使用的计算机权限有限,所以我无法检查您写的内容是否完全正确。虽然这个想法应该没问题 :)
您可能还想为 Windows 开始菜单“启动”文件夹编写一个快捷方式。您可以编写一小段代码来为要运行的应用程序创建快捷方式文件,然后尝试将快捷方式文件写入正确的启动目录?如果不是快捷方式文件,请编写一个脚本文件,这要容易得多。也许是这样的:
@echo off
echo Running a startup program!
pause
::load program
start /b "" "C:\APPLICATIONSPATH.fileextension"
并以编程方式将其写入扩展名为 .vbs 的文件中。这样,如果不懂计算机的用户想要手动删除启动项,他们就可以轻松看到它。(我认为这可能是一种不需要管理权限就可以工作的方法。这可以成为写入注册表的有用替代方法吗?)
如果您使用 Windows 8,则此启动文件夹不再存在于开始菜单下。相反,您可以在此处找到它:C:\Users\YOURUSER\AppData\Roaming\Microsoft\Windows\Start Menu\Programs\Startup
或者使用 Windows 运行提示:Win+R
并运行shell:startup
点击此处查看Windows 8
答案2
我开发了这个模块,它有助于通过注册表方法在 Windows 启动中添加或删除外部应用程序。
它涵盖启动范围(运行/运行一次)、用户范围(当前用户/所有用户)、注册表范围(x86/x64),还能够添加“安全模式”Windows 登录的旁路。
用法示例:
WinStartupUtil.Add(UserScope.CurrentUser, StartupScope.Run, RegistryScope.System32,
title:="Application Title",
filePath:="C:\Application.exe",
arguments:="/Arg1",
secureModeByPass:=True)
WinStartupUtil.Remove(UserScope.CurrentUser, StartupScope.Run, RegistryScope.System32,
title:="Application Title",
throwOnMissingValue:=True)
源代码:
' ***********************************************************************
' Author : Elektro
' Modified : 12-October-2015
' ***********************************************************************
' <copyright file="WinStartupUtil.vb" company="Elektro Studios">
' Copyright (c) Elektro Studios. All rights reserved.
' </copyright>
' ***********************************************************************
#Region " Option Statements "
Option Explicit On
Option Strict On
Option Infer Off
#End Region
#Region " Imports "
Imports System
Imports Microsoft.Win32
#End Region
#Region " WinStartup Util "
''' ----------------------------------------------------------------------------------------------------
''' <summary>
''' Adds or removes an application from Windows Startup.
''' </summary>
''' ----------------------------------------------------------------------------------------------------
Public Module WinStartupUtil
#Region " Constants "
''' ----------------------------------------------------------------------------------------------------
''' <summary>
''' The 'Run' registry subkey path.
''' </summary>
''' ----------------------------------------------------------------------------------------------------
Public Const RunSubKeyPath As String = "Software\Microsoft\Windows\CurrentVersion\Run"
''' ----------------------------------------------------------------------------------------------------
''' <summary>
''' The 'Run' registry subkey path for x86 appications on x64 operating system.
''' </summary>
''' ----------------------------------------------------------------------------------------------------
Public Const RunSubKeyPathSysWow64 As String = "Software\Wow6432Node\Microsoft\Windows\CurrentVersion\Run"
''' ----------------------------------------------------------------------------------------------------
''' <summary>
''' The 'RunOnce' registry subkey path.
''' </summary>
''' ----------------------------------------------------------------------------------------------------
Public Const RunOnceSubKeyPath As String = "Software\Microsoft\Windows\CurrentVersion\RunOnce"
''' ----------------------------------------------------------------------------------------------------
''' <summary>
''' The 'RunOnce' registry subkey path for x86 appications on x64 operating system.
''' </summary>
''' ----------------------------------------------------------------------------------------------------
Public Const RunOnceSubKeyPathSysWow64 As String = "Software\Wow6432Node\Microsoft\Windows\CurrentVersion\RunOnce"
#End Region
#Region " Enumerations "
''' ----------------------------------------------------------------------------------------------------
''' <summary>
''' Specifies an user scope.
''' </summary>
''' ----------------------------------------------------------------------------------------------------
Public Enum UserScope As Integer
''' <summary>
''' 'HKEY_CURRENT_USER' root key.
''' </summary>
CurrentUser = &H1
''' <summary>
''' 'HKEY_LOCAL_MACHINE' root key.
''' </summary>
AllUsers = &H2
End Enum
''' ----------------------------------------------------------------------------------------------------
''' <summary>
''' Specifies a Startup scope.
''' </summary>
''' ----------------------------------------------------------------------------------------------------
Public Enum StartupScope As Integer
''' <summary>
''' 'Run' registry subkey.
''' </summary>
Run = &H1
''' <summary>
''' 'RunOnce' registry subkey.
''' </summary>
RunOnce = &H2
End Enum
''' ----------------------------------------------------------------------------------------------------
''' <summary>
''' Specifies a registry scope.
''' </summary>
''' ----------------------------------------------------------------------------------------------------
Public Enum RegistryScope As Integer
''' <summary>
''' 'System32' registry subkey.
''' </summary>
System32 = &H1
''' <summary>
''' 'SysWow64' registry subkey.
''' </summary>
SysWow64 = &H2
End Enum
#End Region
#Region " Public Methods "
''' ----------------------------------------------------------------------------------------------------
''' <summary>
''' Adds an application to Windows Startup.
''' </summary>
''' ----------------------------------------------------------------------------------------------------
''' <param name="userScope">
''' The user scope.
''' </param>
'''
''' <param name="startupScope">
''' The startup scope.
''' </param>
'''
''' <param name="registryScope">
''' The registry key scope.
''' </param>
'''
''' <param name="title">
''' The registry entry title.
''' </param>
'''
''' <param name="filePath">
''' The application file path.
''' </param>
'''
''' <param name="secureModeByPass">
''' If set to <see langword="True"/>, the file is ran even when the user logs into 'Secure Mode' on Windows.
''' </param>
''' ----------------------------------------------------------------------------------------------------
''' <exception cref="System.ArgumentNullException">
''' title or filePath
''' </exception>
''' ----------------------------------------------------------------------------------------------------
<DebuggerHidden>
<DebuggerStepThrough>
Public Sub Add(ByVal userScope As UserScope,
ByVal startupScope As StartupScope,
ByVal registryScope As RegistryScope,
ByVal title As String,
ByVal filePath As String,
Optional ByVal arguments As String = "",
Optional secureModeByPass As Boolean = False)
If String.IsNullOrEmpty(title) Then
Throw New ArgumentNullException(paramName:="title")
ElseIf String.IsNullOrEmpty(filePath) Then
Throw New ArgumentNullException(paramName:="filePath")
Else
If secureModeByPass Then
title = title.TrimStart("*"c).Insert(0, "*")
End If
Dim regKey As RegistryKey = Nothing
Try
regKey = WinStartupUtil.GetRootKey(userScope).OpenSubKey(GetSubKeyPath(startupScope, registryScope), writable:=True)
regKey.SetValue(title, String.Format("""{0}"" {1}", filePath, arguments), RegistryValueKind.String)
Catch ex As Exception
Throw
Finally
If regKey IsNot Nothing Then
regKey.Close()
End If
End Try
End If
End Sub
''' ----------------------------------------------------------------------------------------------------
''' <summary>
''' Removes an application from Windows Startup.
''' </summary>
''' ----------------------------------------------------------------------------------------------------
''' <param name="userScope">
''' The user scope.
''' </param>
'''
''' <param name="startupScope">
''' The startup scope.
''' </param>
'''
''' <param name="registryScope">
''' The registry scope.
''' </param>
'''
''' <param name="title">
''' The registry entry to find.
''' </param>
'''
''' <param name="throwOnMissingValue">
''' if set to <see langword="true"/>, throws an exception on missing value.
''' </param>
''' ----------------------------------------------------------------------------------------------------
''' <exception cref="System.ArgumentNullException">
''' title
''' </exception>
'''
''' <exception cref="System.ArgumentException">
''' Registry value not found.;title
''' </exception>
''' ----------------------------------------------------------------------------------------------------
<DebuggerHidden>
<DebuggerStepThrough>
Friend Sub Remove(ByVal userScope As UserScope,
ByVal startupScope As StartupScope,
ByVal registryScope As RegistryScope,
ByVal title As String,
Optional ByVal throwOnMissingValue As Boolean = False)
If String.IsNullOrEmpty(title) Then
Throw New ArgumentNullException(paramName:="title")
Else
Dim valueName As String = String.Empty
Dim regKey As RegistryKey = Nothing
Try
regKey = WinStartupUtil.GetRootKey(userScope).OpenSubKey(GetSubKeyPath(startupScope, registryScope), writable:=True)
If (regKey.GetValue(title, defaultValue:=Nothing) IsNot Nothing) Then
valueName = title
ElseIf (regKey.GetValue(title.TrimStart("*"c).Insert(0, "*"), defaultValue:=Nothing) IsNot Nothing) Then
valueName = title.TrimStart("*"c).Insert(0, "*")
ElseIf throwOnMissingValue Then
Throw New ArgumentException(paramName:="title", message:="Registry value not found.")
End If
regKey.DeleteValue(valueName, throwOnMissingValue)
Catch ex As Exception
Throw
Finally
If regKey IsNot Nothing Then
regKey.Close()
End If
End Try
End If
End Sub
#End Region
#Region " Private Methods "
''' ----------------------------------------------------------------------------------------------------
''' <summary>
''' Gets a <see cref="RegistryKey"/> instance of the specified root key.
''' </summary>
''' ----------------------------------------------------------------------------------------------------
''' <param name="userScope">
''' The user scope.
''' </param>
''' ----------------------------------------------------------------------------------------------------
''' <returns>
''' A <see cref="RegistryKey"/> instance of the specified root key.
''' </returns>
''' ----------------------------------------------------------------------------------------------------
''' <exception cref="System.ArgumentException">
''' Invalid enumeration value.;userScope
''' </exception>
''' ----------------------------------------------------------------------------------------------------
<DebuggerHidden>
<DebuggerStepThrough>
Private Function GetRootKey(ByVal userScope As UserScope) As RegistryKey
Select Case userScope
Case WinStartupUtil.UserScope.CurrentUser
Return Registry.CurrentUser
Case WinStartupUtil.UserScope.AllUsers
Return Registry.LocalMachine
Case Else
Throw New ArgumentException("Invalid enumeration value.", "userScope")
End Select ' userScope
End Function
''' ----------------------------------------------------------------------------------------------------
''' <summary>
''' Gets the proper registry subkey path from the parameters criteria.
''' </summary>
''' ----------------------------------------------------------------------------------------------------
''' <param name="startupScope">
''' The startup scope.
''' </param>
'''
''' <param name="registryScope">
''' The registry key scope.
''' </param>
''' ----------------------------------------------------------------------------------------------------
''' <returns>
''' The registry subkey path.
''' </returns>
''' ----------------------------------------------------------------------------------------------------
''' <exception cref="System.ArgumentException">
''' Invalid enumeration value.;startupScope or
''' Invalid enumeration value.;registryScope
''' </exception>
''' ----------------------------------------------------------------------------------------------------
<DebuggerHidden>
<DebuggerStepThrough>
Private Function GetSubKeyPath(ByVal startupScope As StartupScope,
ByVal registryScope As RegistryScope) As String
Select Case registryScope
Case WinStartupUtil.RegistryScope.System32
Select Case startupScope
Case WinStartupUtil.StartupScope.Run
Return WinStartupUtil.RunSubKeyPath
Case WinStartupUtil.StartupScope.RunOnce
Return WinStartupUtil.RunOnceSubKeyPath
Case Else
Throw New ArgumentException("Invalid enumeration value.", "startupScope")
End Select ' startupScope
Case WinStartupUtil.RegistryScope.SysWow64
Select Case startupScope
Case WinStartupUtil.StartupScope.Run
Return WinStartupUtil.RunSubKeyPathSysWow64
Case WinStartupUtil.StartupScope.RunOnce
Return WinStartupUtil.RunOnceSubKeyPathSysWow64
Case Else
Throw New ArgumentException("Invalid enumeration value.", "startupScope")
End Select ' startupScope
Case Else
Throw New ArgumentException("Invalid enumeration value.", "registryScope")
End Select ' registryScope
End Function
#End Region
End Module
#End Region