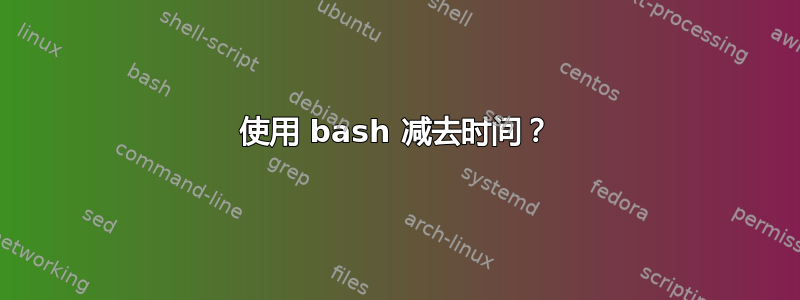
是否可以使用bash减去包含24小时时间的变量?
#!/bin/bash
var1="23:30" # 11:30pm
var2="20:00" # 08:00pm
echo "$(expr $var1 - $var2)"
运行它会产生以下错误。
./test
expr: non-integer argument
我需要输出以十进制形式显示,例如:
./test
3.5
答案1
该date
命令的输入非常灵活。您可以利用它来发挥自己的优势:
#!/bin/bash
var1="23:30"
var2="20:00"
# Convert to epoch time and calculate difference.
difference=$(( $(date -d "$var1" "+%s") - $(date -d "$var2" "+%s") ))
# Divide the difference by 3600 to calculate hours.
echo "scale=2 ; $difference/3600" | bc
输出:
$ ./test.bash
3.50
答案2
仅使用bash
,没有外部程序,您可以这样做:
#!/bin/bash
# first time is the first argument, or 23:30
var1=${1:-23:30}
# second time is the second argument, or 20:00
var2=${2:-20:00}
# Split variables on `:` and insert pieces into arrays
IFS=':' read -r -a t1 <<< "$var1"
IFS=':' read -r -a t2 <<< "$var2"
# strip leading zeros (so it's not interpreted as octal
t1=("${t1[@]##0}")
t2=("${t2[@]##0}")
# check if the first time is before the second one
if (( t1[0] > t2[0] || ( t1[0] == t2[0] && t1[1] > t2[1]) ))
then
# if the minutes on the first time are less than the ones on the second time
if (( t1[1] < t2[1] ))
then
# add 60 minutes to time 1
(( t1[1] += 60 ))
# and subtract an hour
(( t1[0] -- ))
fi
# now subtract the hours and the minutes
echo $((t1[0] -t2[0] )):$((t1[1] - t2[1]))
# to get a decimal result, multiply the minutes by 100 and divide by 60
echo $((t1[0] -t2[0] )).$(((t1[1] - t2[1])*100/60))
else
echo "Time 1 should be after time 2" 2>&1
fi
测试:
$ ./script.sh
3:30
3.50
$ ./script.sh 12:10 11:30
0:40
0.66
$ ./script.sh 12:00 11:30
0:30
0.50
如果您想要更复杂的时差,可能跨越不同的日期等,那么最好使用 GNU 日期。
答案3
使用 Awk,您可以将分隔符设置为空格或冒号来完成所需的计算:
#!/bin/bash
var1="23:30"
var2="20:00"
echo "$var1" "$var2" | awk -F":| " '{print (60*($1-$3)+($2-$4))/60 }'
答案4
手动计算应该很容易:
$ printf '%02d:%02d\n' "$(((d=(${var1/:/*60+})-(${var2/:/*60+})),d/60))" "$((d%60))"
03:30
这里假设$var1
是在之后$var2
,否则你会得到类似的-02:-21
结果。
或者对于 zsh / ksh93 / yash 中的浮点数:
$ echo "$((((${var1/:/*60+})-${var2/:/*60+})/60.))"
3.5
如果必须使用bash
(不支持浮点运算):
$ awk 'BEGIN{print ARGV[1]/60}' "$(((${var1/:/*60+})-${var2/:/*60+}))"
3.5
手动计算浮点部分,不过你也可以进行整个计算awk
。