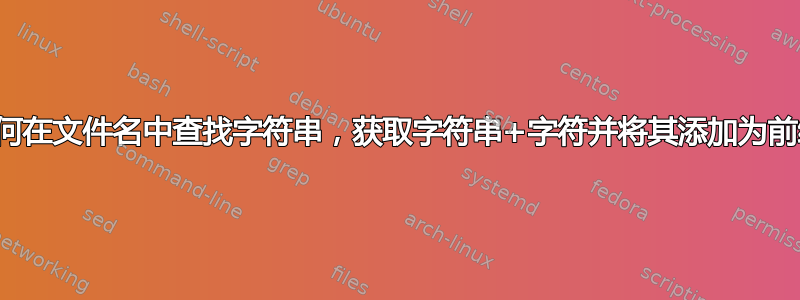
我如何编辑一个文件夹并通过查找固定的字符串重命名该文件夹中的所有文件,然后将该字符串加上四个字符并将其移动到文件名的前缀?
举个例子,我有一个图片文件夹,里面有各种名称,但每个名称中都有日期。我想取出该日期字符串并将其移动到文件名的开头。
这是文件名:
AK_ADVOUTPOSTB1CHARACTER_20171202_406828017946.jpg
如您所见,此文件中有一串“20171202”,但每个文件可能有不同的月份和日期,但它们都是“2017”。
所以我想找到以“2017”开头的字符串,然后将它加上接下来的四个字符移动到文件的开头。
作为最终的解决方案,我希望能够找到字符串“_2017”,取该字符串加上接下来的四个字符,将其编辑为“2017 12-02”,然后将其放在名称的开头。
文件名示例:
AK_ADVOUTPOSTB1CHARACTER_20171202_406828017946.jpg
2017 12-02 AK_ADVOUTPOSTB1CHARACTER_406828017946.jpg
如果需要几个命令才能完成,那对我来说没问题,我只是想尽可能地完成它。
(如果您知道一个可以实现这一点的实用程序,那也会很有帮助。)
答案1
以下 Python 程序将执行这些重命名。
在程序开始时,在配置部分,您需要指定包含年份的模式(尤其是当它从“2017”变为2018时)。
如果您需要更改文件名中年/月/日的位置,可以在函数中更改这些数字check_and_update
。foundAt.start()
(可以使用和访问字符串“_20171202”的索引位置foundAt.end()
)。
#!/usr/bin/env python
import os
import sys
import traceback
import re
# ----------------------------------------------------------------------
# Configuration
# The date string that will be prefixed at the beginning of the file
year_pattern = "_2017[\d]{2}[\d]{2}"
# Only modify files that match this pattern
file_patterns = ["(.*)\.jpg$"]
# Directory to search
start_dirs = ["./"]
# Recursive, check sub-directories
recursive = False
# View the list of modified files, without making any changes
view_modifications_only = True
# ----------------------------------------------------------------------
def add_ending_slash(s):
suffix = "/"
if s.endswith(suffix):
return s
return s + suffix
# ----------------------------------------------------------------------
def pattern_matches_any(filename, file_patterns):
progs = []
for file_pattern in file_patterns:
progs.append(re.compile(file_pattern))
for prog in progs:
if prog.match(filename):
return True
return False
# ----------------------------------------------------------------------
def check_all_dirs(start_dirs):
total_files = 0
try:
for start_dir in start_dirs:
total_files += check_this_dir(start_dir)
except Exception as e:
print('*** Caught exception: %s: %s' % (e.__class__, e))
traceback.print_exc()
return total_files
# ----------------------------------------------------------------------
def check_this_dir(start_dir):
total_files = 0
try:
for filename in os.listdir(start_dir):
filepath = os.path.abspath(add_ending_slash(start_dir) + filename)
# Files
if os.path.isfile(filepath):
total_files += check_and_update(start_dir, filename)
# Directories
elif recursive and os.path.isdir(filepath):
total_files += check_this_dir(filepath)
except PermissionError as e:
print('*** Caught exception: %s: %s' % (e.__class__, e))
pass
return total_files
# ----------------------------------------------------------------------
def check_and_update(start_dir, filename):
if pattern_matches_any(filename, file_patterns):
filepath = os.path.abspath(add_ending_slash(start_dir) + filename)
if (view_modifications_only):
print("[MATCH] " + filepath)
else:
foundAt = re.search(year_pattern, filename)
if (foundAt):
year = filename[foundAt.end()-8:foundAt.end()-4]
month = filename[foundAt.end()-4:foundAt.end()-2]
day = filename[foundAt.end()-2:foundAt.end()]
filename_new = year + " " + month + "-" + day + " " + filename[0:foundAt.start()] + filename[foundAt.end():]
dest = os.path.abspath(add_ending_slash(start_dir) + filename_new)
print("[UPDATE] " + filepath)
if (not os.path.isfile(dest)):
os.rename(filepath, dest)
return 1
return 0
# ----------------------------------------------------------------------
# Main program body
if (view_modifications_only):
print("[INFO] This program is being run in \"read-only\" mode.")
print("[START] The following files will be renamed:")
total_files = check_all_dirs(start_dirs)
print("[END] File count: " + str(total_files))
print()
input("Press Enter to continue...")