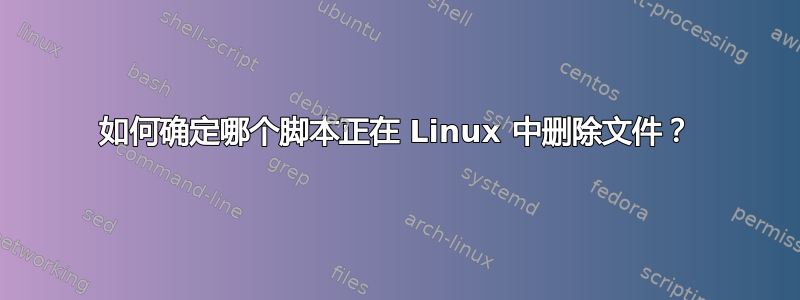
我在 ubuntu 22.04 系统上运行 Plex 媒体服务器 (PMS)。PMS 库已经有一段时间没有更新了,最近我开始向收藏中添加一些电影。但我发现,在添加目录/文件几个小时后,它会从系统中删除,没有任何痕迹。它似乎没有被移动或存档 - 只是被删除了。
有问题的父目录是 /svr/PlexMedia/Movies。例如,我最近添加了这些,但它们在几个小时内就消失了。此后我重新添加了它们:
> find /svr/PlexMedia/Movies/Makin* -ls
195952644 4 drwxrwxr-x 2 dennis dennis 4096 Nov 30 05:10 /svr/PlexMedia/Movies/Making\ Dark\ Side\ of\ the\ Moon\ (2002)
201326598 5449764 -rw-rw-r-- 2 dennis dennis 5580551395 Nov 29 12:43 /svr/PlexMedia/Movies/Making\ Dark\ Side\ of\ the\ Moon\ (2002)/Making\ Dark\ Side\ of\ the\ Moon\ (2002).mp4
由于这个问题,我创建了以下 PHP 脚本来监视文件夹
#!/usr/bin/env php
<?php
// Watch for new media in the specified folder
define('REPORT_WATCH_COUNT', 0x00000001) ;
define('DONT_REPORT_WATCH_COUNT', 0x000000) ;
require_once('lovefunctions.php') ;
$lov->setLogName(LoveFunctions::MONTHLY_LOG_WITH_HEADER) ;
$topFolder = $argv[1] ;
$lov->setLogName(loveFunctions::MONTHLY_LOG) ;
$watchList = inotify_init();
$watchedFolders = [] ;
$watchMask = IN_CREATE | IN_MOVED_TO | IN_MOVED_FROM | IN_DELETE | IN_DELETE_SELF ;
$lov->writeLogLine( [ 'Starting %s. Top directory is %s, watch mask is %08x', basename($lov->pageFile()), $topFolder, $watchMask ] ) ;
addWatch($watchList, $topFolder) ;
// We'll watch our own script file... if our file changes, then we'll resubmit and end - old script is not viable anymore.
$watchMe = inotify_add_watch($watchList, $lov->pageFile(), IN_CREATE | IN_CLOSE_WRITE) ;
$watchedFolders[$watchMe] = [ 'path' => $lov->pageFile() ] ;
$lov->writeLogLine( [ 'Watching for changes to this script (%s). Watch ID is %d.', $lov->pageFile(), $watchMe ] ) ;
watchLoop($watchList, $watchMe, $watchMask) ;
array_reverse($watchedFolders) ;
foreach ($watchedFolders as $watchId => $watchItem) {
inotify_rm_watch($watchList, $watchId) ;
}
fclose($watchList);
function addWatch($watchList, string $folderName, int $flags = REPORT_WATCH_COUNT) {
global $lov, $watchedFolders, $watchMask ;
if (is_dir($folderName)) {
if (substr($folderName, -1) != DIRECTORY_SEPARATOR) $folderName .= DIRECTORY_SEPARATOR ;
$watchId = inotify_add_watch($watchList, $folderName, $watchMask) ;
if ($watchId) {
$watchedFolders[$watchId] = [ 'path' => $folderName
, 'events' => 0
] ;
$files = scandir($folderName) ;
$lov->writeLogLine( [ 'Added %s to the watch list (id=%d).', $folderName, $watchId ] ) ;
foreach ($files as $file) {
if ($file == '.' || $file == '..')
continue ;
$file = $folderName . $file ;
if (is_dir($file))
addWatch($watchList, $file, DONT_REPORT_WATCH_COUNT) ;
}
}
}
if (($flags & REPORT_WATCH_COUNT) == REPORT_WATCH_COUNT)
reportCount(count($watchedFolders) - 1) ;
return ;
}
function removeWatch($watchList, $watchId, int $flags = REPORT_WATCH_COUNT, int $mask = 0) {
global $lov, $watchedFolders ;
if (isset($watchedFolders[$watchId])) {
$watch = $watchedFolders[$watchId] ;
if ($watch['events'] > 0)
$msg = sprintf('Removing watch %d (folder %s) after %d events triggered.', $watchId, $watch['path'], $watch['events']) ;
else
$msg = sprintf('Removing unused watch %d (folder %s)', $watchId, $watch['path']) ;
$lov->writeLogLine( $msg ) ;
unset($watchedFolders[$watchId]) ;
if (($mask & IN_DELETE_SELF) != IN_DELETE_SELF) // Seems the system already removes this for us
inotify_rm_watch($watchList, $watchId) ;
}
if (($flags & REPORT_WATCH_COUNT) == REPORT_WATCH_COUNT)
reportCount(count($watchedFolders) - 1) ;
}
function reportCount($count) {
global $lov ;
$lov->writeLogLine( [ 'There %s now %d folder%s in the watch list (plus the self-monitor)', $count == 1 ? 'is' : 'are', $count, $count == 1 ? '' : 's' ] ) ;
}
function checkSyntax(string $scriptName) : bool {
global $lov ;
$output = [] ;
$rc = -1 ;
$cmd = sprintf('php -l %s 2>&1', escapeshellarg($scriptName)) ;
exec($cmd, $output, $rc) ;
if ($rc != 0) // Not successful
return false ;
foreach ($output AS $line) {
if (preg_match('%^(.*error: |Errors parsing )%', $line))
return false ; // Syntax error or other error
}
return true ;
}
function watchLoop($watchList, int $watchMe, int $watchMask) {
global $lov, $watchedFolders , $pdo, $topFolder ;
$floodStart = null ;
while (true) {
$mtimeStart = microtime(true) ;
$events = inotify_read($watchList);
$mtimeEnd = microtime(true) ;
if (! $events) {
$lov->writeLogLine( [ 'Something is seriously wrong! inotify_read returned false/null/empty response. Giving up.' ] ) ;
return false ;
}
if (($elapsed = ($mtimeEnd - $mtimeStart)) < 1) { // Less than a second since prior - may not be a problem, but let's watch closely...
if (is_null($floodStart)) {
$floodStart = $mtimeStart ;
$floodCount = count($events) ;
} else {
$floodCount += count($events) ;
if ($floodCount > 100) { // More than one hundred events, all within a second of each other. We seem to be looping.
$lov->writeLogLine( [ 'In %.3f seconds, there have been %d events. Too much too fast. Giving up.', microtime() - $floodStart, $floodCount ] ) ;
}
}
} else { // Elapsed > 1 second. This is normal.
$floodStart = null ; // Call off the dogs
}
foreach ($events as $event) {
$mask = $event['mask'] ;
$watchId = $event['wd'] ;
if ( ! array_key_exists($watchId, $watchedFolders)) {
$lov->writeLogLine( [ 'Received event %08x for watch ID %d but watchId is not in our watchedFolders array.', $mask, $watchId ] ) ;
continue ;
}
$watch = &$watchedFolders[$watchId] ;
if (is_null($watch)) {
$lov->writeLogLine( [ 'Wanted to access $watchedFolders[%d] but it\'s null. Removing it.', $watchId ] ) ;
unset($watchedFolders[$watchId]) ;
continue ;
}
$filePath = $watch['path'] . $event['name'] ;
$accessed = $mask & IN_ACCESS ;
$modified = $mask & IN_MODIFY ;
$attrChange = $mask & IN_ATTRIB ;
$closeWrite = $mask & IN_CLOSE_WRITE ;
$closeNowrite = $mask & IN_CLOSE_NOWRITE ;
$opened = $mask & IN_OPEN ;
$movedTo = $mask & IN_MOVED_TO ;
$movedFrom = $mask & IN_MOVED_FROM ;
$created = $mask & IN_CREATE ;
$deleted = $mask & IN_DELETE ;
$deleteSelf = $mask & IN_DELETE_SELF ;
$movedSelf = $mask & IN_MOVE_SELF ;
$moved = $mask & IN_MOVE ;
$unmount = $mask & IN_UNMOUNT ;
$overflow = $mask & IN_Q_OVERFLOW ;
$ignored = $mask & IN_IGNORED ;
$isDir = $mask & IN_ISDIR ;
$onlyDir = $mask & IN_ONLYDIR ;
$dontFollow = $mask & IN_DONT_FOLLOW ;
$lov->writeLogLine( [ 'iNotify alert (%08x) for file: %s:%s'
, $mask
, $filePath
, ($accessed ? ' accessed' : '')
. ($modified ? ' modified' : '')
. ($attrChange ? ' attrChange' : '')
. ($closeWrite ? ' closeWrite' : '')
. ($closeNowrite ? ' closeNoWrite' : '')
. ($opened ? ' opened' : '')
. ($movedTo ? ' movedTo' : '')
. ($movedFrom ? ' movedFrom' : '')
. ($created ? ' created' : '')
. ($deleted ? ' deleted' : '')
. ($deleteSelf ? ' deleteSelf' : '')
. ($movedSelf ? ' movedSelf' : '')
. ($moved ? ' moved' : '')
. ($unmount ? ' unmount' : '')
. ($overflow ? ' overflow' : '')
. ($ignored ? ' ignored' : '')
. ($isDir ? ' isDir' : '')
. ($onlyDir ? ' onlyDir' : '')
. ($dontFollow ? ' dontFollow' : '')
] ) ;
if ($overflow)
$lov->writeLogLine( [ 'Overflow has been indicated (%X). Not much I can do about it, but I need to let you know.', $overflow ] ) ;
if ($watchId == $watchMe) {
// OK, our script has proably been chenged. Before we do anything crazy, let's check the syntax. If all is well,
// then we will submit a new process and end this one.
if (checkSyntax($lov->pageFile())) { // Good to go.... let's let the new guy take over from here
$lov->writeLogLine( "Hey, that's me! Better resubmit myself to start a new life!") ;
$cmd = sprintf('sleep 2 && nohup php %s %s >> %s 2>&1 &', escapeshellarg($lov->pageFile()), escapeshellarg($topFolder), escapeshellarg($lov->logNameOverride)) ;
$lov->writeLogLine( [ 'Command is %s', $cmd ] ) ;
$output = [] ;
exec($cmd, $output) ;
foreach($output as $line) {
if (strlen(trim($line)) > 0)
$lov->continueLogLine( $line) ;
}
break 2 ;
} else { // Syntax errors in new script
$lov->writeLogLine( [ "That's me, but looks like there are syntax errors in the new file, so I'll just hang out here for a while." ] ) ;
}
continue ;
} elseif ($deleteSelf) { // Deletion of a watched directory
removeWatch($watchList, $watchId, REPORT_WATCH_COUNT, $mask) ;
continue ;
} elseif ($deleted)
continue ; // Ignore file deletions (other than $deleteSelf, which we have already accommodated)
elseif ($created && $isDir) { // If it's a new directory, we need only to add it to the watch list
// Need to watch this folder
$watch['events'] ++ ; // Record this event in the parent folder
addWatch($watchList, $filePath) ; // Then add the child
continue ;
} elseif ($created || $closeWrite) {
$watch['events'] ++ ;
// This is where we check to see if it's a movie, etc, etc....
}
} // foreach
} // while (true)
} // End of watchLoop
我以 /svr/PlexMedia/Movies 作为监视文件夹来运行该脚本,果然,在我添加了几部电影大约一小时后,它们就被删除了:
2023-11-30 00:25:03 /usr/local/bin/watchFolder: iNotify alert (00000200) for file: /svr/PlexMedia/Movies/Passion of the Christ, The (1982)/Passion of the Christ, The (1982).mp4: deleted
2023-11-30 00:25:03 /usr/local/bin/watchFolder: iNotify alert (00000400) for file: /svr/PlexMedia/Movies/Passion of the Christ, The (1982)/: deleteSelf
2023-11-30 00:25:03 /usr/local/bin/watchFolder: Removing watch 690 (folder /svr/PlexMedia/Movies/Passion of the Christ, The (1982)/) after 1 events triggered.
2023-11-30 00:25:03 /usr/local/bin/watchFolder: There are now 688 folders in the watch list (plus the self-monitor)
2023-11-30 00:25:03 /usr/local/bin/watchFolder: Received event 00008000 for watch ID 690 but watchId is not in our watchedFolders array.
2023-11-30 00:25:03 /usr/local/bin/watchFolder: iNotify alert (40000200) for file: /svr/PlexMedia/Movies/Passion of the Christ, The (1982): deleted isDir
2023-11-30 00:25:03 /usr/local/bin/watchFolder: iNotify alert (00000200) for file: /svr/PlexMedia/Movies/Making Dark Side of the Moon (2002)/Making Dark Side of the Moon (2002).mp4: deleted
2023-11-30 00:25:03 /usr/local/bin/watchFolder: iNotify alert (00000400) for file: /svr/PlexMedia/Movies/Making Dark Side of the Moon (2002)/: deleteSelf
2023-11-30 00:25:03 /usr/local/bin/watchFolder: Removing watch 689 (folder /svr/PlexMedia/Movies/Making Dark Side of the Moon (2002)/) after 1 events triggered.
2023-11-30 00:25:03 /usr/local/bin/watchFolder: There are now 687 folders in the watch list (plus the self-monitor)
2023-11-30 00:25:03 /usr/local/bin/watchFolder: Received event 00008000 for watch ID 689 but watchId is not in our watchedFolders array.
2023-11-30 00:25:03 /usr/local/bin/watchFolder: iNotify alert (40000200) for file: /svr/PlexMedia/Movies/Making Dark Side of the Moon (2002): deleted isDir
2023-11-30 00:25:03 /usr/local/bin/watchFolder: iNotify alert (00000200) for file: /svr/PlexMedia/Movies/nohup.out: deleted
(我希望日志中的时间可以帮助我通过 syslog 识别某些进程(我当时想也许是来自 cron 的某些东西;现在我很确定不是,因为在任何一小时后的 25 分钟内都没有什么不寻常的安排。)
因此,我希望找到一种方法来审计这些删除。我遇到了 auditd(我现在已经安装了它),并通过以下命令开始监控:
sudo auditctl -w /svr/PlexMedia/Movies/ -p rw -k var-run-pids
然后我创建并删除了一个文件:touch /svr/PlexMedia/Movies/me rm /svr/PlexMedia/Movies/me
果然,我得到了该事件的审计日志。
----
time->Thu Nov 30 05:07:29 2023
type=PROCTITLE msg=audit(1701338849.085:1607): proctitle=746F756368002F7376722F506C65784D656469612F4D6F766965732F6D65
type=PATH msg=audit(1701338849.085:1607): item=1 name="/svr/PlexMedia/Movies/me" inode=189727122 dev=08:01 mode=0100664 ouid=1000 ogid=1000 rdev=00:00 nametype=CREATE cap_fp=0 cap_fi=0 cap_fe=0 cap_fver=0 cap_frootid=0
type=PATH msg=audit(1701338849.085:1607): item=0 name="/svr/PlexMedia/Movies/" inode=189726727 dev=08:01 mode=044777 ouid=997 ogid=0 rdev=00:00 nametype=PARENT cap_fp=0 cap_fi=0 cap_fe=0 cap_fver=0 cap_frootid=0
type=CWD msg=audit(1701338849.085:1607): cwd="/home/dennis"
type=SYSCALL msg=audit(1701338849.085:1607): arch=c000003e syscall=257 success=yes exit=3 a0=ffffff9c a1=7ffe6103cbb1 a2=941 a3=1b6 items=2 ppid=4936 pid=502375 auid=1000 uid=1000 gid=1000 euid=1000 suid=1000 fsuid=1000 egid=1000 sgid=1000 fsgid=1000 tty=pts0 ses=6 comm="touch" exe="/usr/bin/touch" key="var-run-pids"
----
time->Thu Nov 30 05:07:32 2023
type=PROCTITLE msg=audit(1701338852.085:1608): proctitle=726D002F7376722F506C65784D656469612F4D6F766965732F6D65
type=PATH msg=audit(1701338852.085:1608): item=1 name="/svr/PlexMedia/Movies/me" inode=189727122 dev=08:01 mode=0100664 ouid=1000 ogid=1000 rdev=00:00 nametype=DELETE cap_fp=0 cap_fi=0 cap_fe=0 cap_fver=0 cap_frootid=0
type=PATH msg=audit(1701338852.085:1608): item=0 name="/svr/PlexMedia/Movies/" inode=189726727 dev=08:01 mode=044777 ouid=997 ogid=0 rdev=00:00 nametype=PARENT cap_fp=0 cap_fi=0 cap_fe=0 cap_fver=0 cap_frootid=0
type=CWD msg=audit(1701338852.085:1608): cwd="/home/dennis"
type=SYSCALL msg=audit(1701338852.085:1608): arch=c000003e syscall=263 success=yes exit=0 a0=ffffff9c a1=557df82784d0 a2=0 a3=0 items=2 ppid=4936 pid=502383 auid=1000 uid=1000 gid=1000 euid=1000 suid=1000 fsuid=1000 egid=1000 sgid=1000 fsgid=1000 tty=pts0 ses=6 comm="rm" exe="/usr/bin/rm" key="var-run-pids"
从上面的日志中很容易看出,这是由 uid 1000 完成的,没有 setuid 等。所以我抱有希望。
但当真实情况发生时,日志并没有给我带来我所希望的结果。这是我的 littls 脚本报告的内容:
2023-11-30 08:25:06 /usr/local/bin/watchFolder: iNotify alert (00000200) for file: /svr/PlexMedia/Movies/Passion of the Christ, The (1982)/Passion of the Christ, The (1982).mp4: deleted
2023-11-30 08:25:06 /usr/local/bin/watchFolder: iNotify alert (00000400) for file: /svr/PlexMedia/Movies/Passion of the Christ, The (1982)/: deleteSelf
2023-11-30 08:25:06 /usr/local/bin/watchFolder: Removing watch 692 (folder /svr/PlexMedia/Movies/Passion of the Christ, The (1982)/) after 1 events triggered.
2023-11-30 08:25:06 /usr/local/bin/watchFolder: There are now 688 folders in the watch list (plus the self-monitor)
2023-11-30 08:25:06 /usr/local/bin/watchFolder: Received event 00008000 for watch ID 692 but watchId is not in our watchedFolders array.
2023-11-30 08:25:06 /usr/local/bin/watchFolder: iNotify alert (40000200) for file: /svr/PlexMedia/Movies/Passion of the Christ, The (1982): deleted isDir
2023-11-30 08:25:06 /usr/local/bin/watchFolder: iNotify alert (00000200) for file: /svr/PlexMedia/Movies/Making Dark Side of the Moon (2002)/Making Dark Side of the Moon (2002).mp4: deleted
2023-11-30 08:25:06 /usr/local/bin/watchFolder: iNotify alert (00000400) for file: /svr/PlexMedia/Movies/Making Dark Side of the Moon (2002)/: deleteSelf
2023-11-30 08:25:06 /usr/local/bin/watchFolder: Removing watch 691 (folder /svr/PlexMedia/Movies/Making Dark Side of the Moon (2002)/) after 1 events triggered.
2023-11-30 08:25:06 /usr/local/bin/watchFolder: There are now 687 folders in the watch list (plus the self-monitor)
2023-11-30 08:25:06 /usr/local/bin/watchFolder: Received event 00008000 for watch ID 691 but watchId is not in our watchedFolders array.
2023-11-30 08:25:06 /usr/local/bin/watchFolder: iNotify alert (40000200) for file: /svr/PlexMedia/Movies/Making Dark Side of the Moon (2002): deleted isDir
但审计日志完全没有提及这个时间范围:
sudo ausearch -f "/svr/PlexMedia/Movies/Making Dark Side of the Moon (2002)"
[sudo] password for dennis:
----
time->Thu Nov 30 05:16:23 2023
type=PROCTITLE msg=audit(1701339383.653:4763): proctitle=66696E64002F7376722F506C65784D656469612F4D6F766965732F4D616B696E67204461726B2053696465206F6620746865204D6F6F6E20283230303229002D6C73
type=PATH msg=audit(1701339383.653:4763): item=0 name=2F7376722F506C65784D656469612F4D6F766965732F4D616B696E67204461726B2053696465206F6620746865204D6F6F6E20283230303229 inode=195952644 dev=08:01 mode=040775 ouid=1000 ogid=1000 rdev=00:00 nametype=NORMAL cap_fp=0 cap_fi=0 cap_fe=0 cap_fver=0 cap_frootid=0
type=CWD msg=audit(1701339383.653:4763): cwd="/svr/plex-safeguard-against-deletion"
type=SYSCALL msg=audit(1701339383.653:4763): arch=c000003e syscall=257 success=yes exit=4 a0=ffffff9c a1=55b159c93d40 a2=b0900 a3=0 items=1 ppid=431537 pid=504053 auid=1000 uid=1000 gid=1000 euid=1000 suid=1000 fsuid=1000 egid=1000 sgid=1000 fsgid=1000 tty=pts6 ses=549 comm="find" exe="/usr/bin/find" key="var-run-pids"
----
time->Thu Nov 30 08:03:05 2023
type=PROCTITLE msg=audit(1701349385.858:4983): proctitle=2F7573722F6C69622F706C65786D656469617365727665722F506C6578204D6564696120536572766572
type=PATH msg=audit(1701349385.858:4983): item=0 name=2F7376722F506C65784D656469612F4D6F766965732F4D616B696E67204461726B2053696465206F6620746865204D6F6F6E20283230303229 inode=195952644 dev=08:01 mode=040775 ouid=1000 ogid=1000 rdev=00:00 nametype=NORMAL cap_fp=0 cap_fi=0 cap_fe=0 cap_fver=0 cap_frootid=0
type=CWD msg=audit(1701349385.858:4983): cwd="/"
type=SYSCALL msg=audit(1701349385.858:4983): arch=c000003e syscall=2 success=yes exit=59 a0=7f500d8b55b0 a1=98000 a2=0 a3=0 items=1 ppid=1 pid=874 auid=4294967295 uid=997 gid=997 euid=997 suid=997 fsuid=997 egid=997 sgid=997 fsgid=997 tty=(none) ses=4294967295 comm=506C6578204D656469612053657276 exe=2F7573722F6C69622F706C65786D656469617365727665722F506C6578204D6564696120536572766572 key="var-run-pids"
----
time->Thu Nov 30 08:03:05 2023
type=PROCTITLE msg=audit(1701349385.858:4984): proctitle=2F7573722F6C69622F706C65786D656469617365727665722F506C6578204D6564696120536572766572
type=PATH msg=audit(1701349385.858:4984): item=0 name=2F7376722F506C65784D656469612F4D6F766965732F4D616B696E67204461726B2053696465206F6620746865204D6F6F6E202832303032292F4D616B696E67204461726B2053696465206F6620746865204D6F6F6E202832303032292E6D7034 inode=201326598 dev=08:01 mode=0100664 ouid=1000 ogid=1000 rdev=00:00 nametype=NORMAL cap_fp=0 cap_fi=0 cap_fe=0 cap_fver=0 cap_frootid=0
type=CWD msg=audit(1701349385.858:4984): cwd="/"
type=SYSCALL msg=audit(1701349385.858:4984): arch=c000003e syscall=2 success=yes exit=59 a0=7f500f450b90 a1=88000 a2=0 a3=0 items=1 ppid=1 pid=874 auid=4294967295 uid=997 gid=997 euid=997 suid=997 fsuid=997 egid=997 sgid=997 fsgid=997 tty=(none) ses=4294967295 comm=506C6578204D656469612053657276 exe=2F7573722F6C69622F706C65786D656469617365727665722F506C6578204D6564696120536572766572 key="var-run-pids"
----
time->Thu Nov 30 08:03:27 2023
type=PROCTITLE msg=audit(1701349407.057:6549): proctitle=2F7573722F6C69622F706C65786D656469617365727665722F506C6578204D6564696120536572766572
type=PATH msg=audit(1701349407.057:6549): item=0 name=2F7376722F506C65784D656469612F4D6F766965732F4D616B696E67204461726B2053696465206F6620746865204D6F6F6E20283230303229 inode=195952644 dev=08:01 mode=040775 ouid=1000 ogid=1000 rdev=00:00 nametype=NORMAL cap_fp=0 cap_fi=0 cap_fe=0 cap_fver=0 cap_frootid=0
type=CWD msg=audit(1701349407.057:6549): cwd="/"
type=SYSCALL msg=audit(1701349407.057:6549): arch=c000003e syscall=2 success=yes exit=68 a0=7f500f89af50 a1=98000 a2=0 a3=0 items=1 ppid=1 pid=874 auid=4294967295 uid=997 gid=997 euid=997 suid=997 fsuid=997 egid=997 sgid=997 fsgid=997 tty=(none) ses=4294967295 comm=506C6578204D656469612053657276 exe=2F7573722F6C69622F706C65786D656469617365727665722F506C6578204D6564696120536572766572 key="var-run-pids"
----
time->Thu Nov 30 08:03:27 2023
type=PROCTITLE msg=audit(1701349407.057:6550): proctitle=2F7573722F6C69622F706C65786D656469617365727665722F506C6578204D6564696120536572766572
type=PATH msg=audit(1701349407.057:6550): item=0 name=2F7376722F506C65784D656469612F4D6F766965732F4D616B696E67204461726B2053696465206F6620746865204D6F6F6E202832303032292F4D616B696E67204461726B2053696465206F6620746865204D6F6F6E202832303032292E6D7034 inode=201326598 dev=08:01 mode=0100664 ouid=1000 ogid=1000 rdev=00:00 nametype=NORMAL cap_fp=0 cap_fi=0 cap_fe=0 cap_fver=0 cap_frootid=0
type=CWD msg=audit(1701349407.057:6550): cwd="/"
type=SYSCALL msg=audit(1701349407.057:6550): arch=c000003e syscall=2 success=yes exit=68 a0=7f500f450a90 a1=88000 a2=0 a3=0 items=1 ppid=1 pid=874 auid=4294967295 uid=997 gid=997 euid=997 suid=997 fsuid=997 egid=997 sgid=997 fsgid=997 tty=(none) ses=4294967295 comm=506C6578204D656469612053657276 exe=2F7573722F6C69622F706C65786D656469617365727665722F506C6578204D6564696120536572766572 key="var-run-pids"
也许 auditd 可以帮我做这件事,但我不熟悉这个工具,所以无法做到……我不知道。在我进入另一个兔子洞之前,我想在这里问一下,是否有某种神奇的方法可以找出触发删除这些文件的脚本或事件。它似乎很有针对性——正如我所暗示的,这个库已经存在很久了,而且(我能确定的是)这个长期存在的库是完好无损的。但它似乎坚持不添加任何新内容(或者说,确实不会添加很长时间)。
有什么帮助吗?