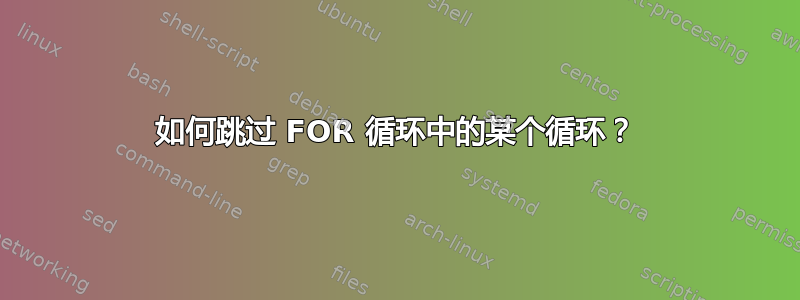
我正在运行一个批处理脚本来处理一些文件。当它确定当前文件不需要处理时,我想跳过该文件的其余命令并直接转到下一个命令。怎么做?Goto :EOF
两者exit /b
都退出整个脚本。
这是脚本。它获取一个目录并重命名所有文件以包含父目录名称中的所有单词(文件名中已包含的单词除外)。
@echo off
setlocal enabledelayedexpansion
rem set input directory (add check for validity)
set "inputDir=%~1"
set "confirmation=false"
:proceed
for /r "%inputDir%" %%F in (*.*) do (
rem use path to file as keywords
set "words=%%~pF"
rem replace known useless marks with spaces
set "words=!words:\= !"
set "words=!words:_= !"
set "words=!words:,= !"
set "words=!words: = !"
rem remove known useless words
set "words=!words: The = !"
set "words=!words: A = !"
set "words=!words: An = !"
set "words=!words: Video = !"
set "words=!words: New Folder = !"
rem remove word that already exists in the filename
for %%W in (!words!) do echo %%~nF | find /i "%%W" >nul && set "words=!words: %%W = !"
rem replace leading and trailing spaces with brackets
set "words=[!words:~1,-1!]"
rem if all words already included end task for current file
if "!words!"=="[]" exit /b
rem build the new filename
set "newName=%%~nF !words!%%~xF"
rem fix "] [" caused by repeated renaming, causes fusion with non-related bracketed sets, which is fine
set "newName=!newName:] [= !"
rem task for displaying the name change for confirmation
if /i not "%confirmation%"=="yes" echo old "%%F" && echo new "!newName!"
rem task for doing the actual rename if confirmed
if /i "%confirmation%"=="yes" echo ren "%%~nxF" "!newName!" & echo ren "%%F" "!newName!"
)
rem fails to rename files with ! mark in the filename. no other trouble, just won't rename those. error: the syntax of the command is incorrect
rem if coming from second (completed) run then exit
if /i "%confirmation%"=="yes" exit /b
rem ask for confirmation to run again for real
set /p confirmation="confirm you want to perform all these rename tasks (type yes or no)?: "
if /i not "%confirmation%"=="yes" echo confirmation denied
if /i "%confirmation%"=="yes" goto proceed
endlocal
我也正在尝试一种有条件地运行最后一个命令的替代方法,如下所示
@echo off
setlocal enabledelayedexpansion
rem set input directory (add check for validity)
set "inputDir=%~1"
set "confirmation=false"
:proceed
for /r "%inputDir%" %%F in (*.*) do (
rem use path to file as keywords
set "words=%%~pF"
rem replace known useless marks with spaces
set "words=!words:\= !"
set "words=!words:_= !"
set "words=!words:,= !"
set "words=!words: = !"
rem remove known useless words
set "words=!words: The = !"
set "words=!words: A = !"
set "words=!words: An = !"
set "words=!words: Video = !"
set "words=!words: New Folder = !"
rem remove word that already exists in the filename
for %%W in (!words!) do echo %%~nF | find /i "%%W" >nul && set "words=!words: %%W = !"
rem replace leading and trailing spaces with brackets
set "words=[!words:~1,-1!]"
rem if all words not already included in the filename do
if not "!words!"=="[]" (
rem build the new filename
set "newName=%%~nF !words!%%~xF"
rem fix "] [" caused by repeated renaming, causes fusion with non-related bracketed sets, which is fine
set "newName=!newName:] [= !"
rem task for displaying the name change for confirmation
if /i not "%confirmation%"=="yes" echo old "%%F" && echo new "!newName!"
rem task for doing the actual rename if confirmed
if /i "%confirmation%"=="yes" echo ren "%%~nxF" "!newName!" & echo ren "%%F" "!newName!"
)
)
rem fails to rename files with ! mark in the filename. no other trouble, just won't rename those. error: the syntax of the command is incorrect
rem if coming from second (completed) run then exit
if /i "%confirmation%"=="yes" exit /b
rem ask for confirmation to run again for real
set /p confirmation="confirm you want to perform all these rename tasks (type yes or no)?: "
if /i not "%confirmation%"=="yes" echo confirmation denied
if /i "%confirmation%"=="yes" goto proceed
endlocal
但这是一场灾难,导致文件名被从文件名中看似随机的部分剪切下来并words
添加。
答案1
只需将标签:nextiteration
(或任何您喜欢的其他名称)作为 for 循环主体中的最后一条语句,goto
当您决定可以跳过 for 主体的其余部分时,就将标签放在该标签上。
因为 FOR 主体上的右括号前面必须有一个常规语句(标签不行),所以您必须在标签和右括号之间放置一个伪语句(如 REM)。
FOR xxxxxxxxx DO (
some statements (executed on each iteration)
IF {some condition is met} GOTO nextiteration
statements that will be skipped if conditon is met
:nextiteration
REM to prevent syntax error
)
答案2
Windows 批处理没有太多选项可以跳过循环迭代。您可以使用的方法是:
将 IF 块放在您想要跳过的循环部分周围:
@Echo Off if A equ A ( IF B==C ( Echo shouldn't go here ) )
用于
call
调用包含整个内部循环的例程,其中exit/B
将起作用:@Echo Off Setlocal For %%A in (alpha beta gamma) DO ( Echo Outer loop %%A Call :inner ) Goto :eof :inner For %%B in (U V W X Y Z) DO ( if %%B==X ( exit /b 2 ) Echo Inner loop Outer=%%A Inner=%%B )
使用命令
goto :Label
并将其放在Label:
循环末尾。由于已知错误,此方法可能不适用于您的情况:在括号内使用 GOTO(包括 FOR 和 IF 命令)会破坏它。
以下示例显示了此错误:@Echo Off if A equ A ( IF B==C GOTO specialcase Echo shouldn't go here goto:eof :specialcase )
参考 :
答案3
尝试使用For /F...
和... | findstr /c:"string1" /c:"string2"...
使用循环可以过滤字符串,从而更好地控制结果中间文件名的操作,这样可以更轻松地进行交互来重命名它们,而无需尝试跳过循环,因为它变得更加精确:
@echo off & setlocal
2>nul cd /d "%~1" || exit
setlocal enabledelayedexpansion
for %%G in =;(
"_",","," "," The "," A "," An "," Video "," New Folder "
);= do if not defined _str2find =;( set "_str2find=/c:"%%~G""
);= else call set "_str2find=!_str2find! /c:"%%~G""
setlocal disabledelayedexpansion
for /f usebackq^delims^= %%G in (`
2^>nul where /r . *.* ^| findstr %_str2find% `);= do =;(
echo/%%~G
:: So you don't need to use skip, the rest of your
:: interactions continue here and within your loop
);=
endlocal