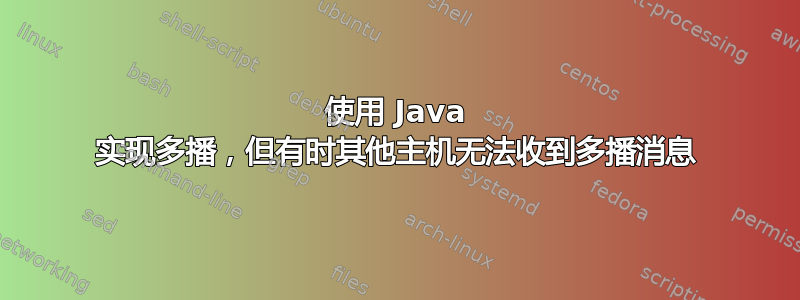
我正在使用 Java 实现多播,但遇到了一些问题。当我从 [主机 A 接收器 -> 主机 B 接收器 -> 主机 A 发送器] 开始时,主机 B 无法接收多播消息。但是,当我从 [主机 B 接收器 -> 主机 A 接收器 -> 主机 A 发送器] 开始时,主机 B 可以接收多播消息。主机 A 和主机 B 位于同一个 LAN 中。这可能是什么原因造成的?
这是我的接收器和发送器代码。
import java.io.IOException;
import java.net.DatagramPacket;
import java.net.InetAddress;
import java.net.MulticastSocket;
/**
* @author 肖嘉威 [email protected]
* @date 2024/4/23 11:33
*/
public class MulticastSender {
public static void main(String[] args) throws IOException {
InetAddress group = InetAddress.getByName("234.2.3.4");
int port = 12122;
MulticastSocket ms = null;
try {
ms = new MulticastSocket(port);
ms.joinGroup(group);
while (true) {
String message = "Hello " + new java.util.Date();
byte[] buffer = message.getBytes();
DatagramPacket dp = new DatagramPacket(buffer, buffer.length, group, port);
ms.send(dp);
System.out.println("发送数据报给" + group + ":" + port);
Thread.sleep(2000);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (ms != null) {
try {
ms.leaveGroup(group);
ms.close();
} catch (IOException e) {
}
}
}
}
}
import java.io.IOException;
import java.net.DatagramPacket;
import java.net.InetAddress;
import java.net.MulticastSocket;
/**
* @author 肖嘉威 [email protected]
* @date 2024/4/23 11:34
*/
public class MulticastReceiver {
public static void main(String[] args) throws Exception {
InetAddress group = InetAddress.getByName("234.2.3.4");
int port = 12122;
MulticastSocket ms = null;
try {
ms = new MulticastSocket(port);
ms.joinGroup(group);
byte[] buffer = new byte[8192];
while (true) {
DatagramPacket dp = new DatagramPacket(buffer, buffer.length);
ms.receive(dp);
String s = new String(dp.getData(), 0, dp.getLength());
System.out.println(s);
}
}catch (IOException e){
e.printStackTrace();
}finally
{
if (ms !=null)
{
try
{
ms.leaveGroup(group);
ms.close();
} catch (IOException e) { }
}
}
}
}
我使用了 wireshark。这是第一次启动顺序的结果
主机B
主机A
这是第二次启动顺序的结果