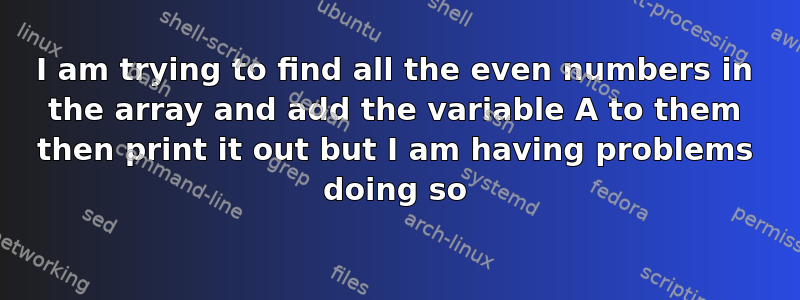
#!/bin/bash
declare -i A=2
ADD()
{
declare -a Arr
count=1
MAXCOUNT=4
#declare -a arr
while [ "$count" -le "$MAXCOUNT" ]; do
Arr[$count]=$(( RANDOM % 10 + 1 ))
(( count++ )) || true
done
for i in "${Arr[@]}"; do
if [ $((Arr[i]%2)) -eq 0 ]; then
A+=$(Arr[i])
fi
done
echo "$A"
}
ADD
./math1804262.sh: line 35: Arr[*]: command not found
./math1804262.sh: line 35: Arr[*]: command not found
no
./math1804262.sh: line 35: Arr[*]: command not found
答案1
Code 1 :
#!/bin/bash
declare -i A=2
ADD()
{
start_value=$1
end_value=$2
dynamic_array=()
for (( i=$start_value ; i<=$end_value ; i++ ))
{
rem=$(( $i % 2 ))
if [ $rem -eq 0 ]
then
#echo "Number : $i print's even number"
dynamic_array+=("$i")
else
# echo "Not a even number : $i"
fi
}
# Printing all even numbers
echo ${dynamic_array[@]}
}
ADD 1 7
Output 1: 2 4 6
The code 2 will print the A alternatively , just little bit change made in dynamic array storing
Code 2 :
#!/bin/bash
declare -i A=2
ADD()
{
start_value=$1
end_value=$2
dynamic_array=()
for (( i=$start_value ; i<=$end_value ; i++ ))
{
rem=$(( $i % 2 ))
if [ $rem -eq 0 ]
then
#echo "Number : $i print's even number"
dynamic_array+=("$i A")
else
echo "Not a even number : $i"
fi
}
# Printing all even numbers
echo ${dynamic_array[@]}
}
ADD 1 7
Output 2 :
2 A 4 A 6 A