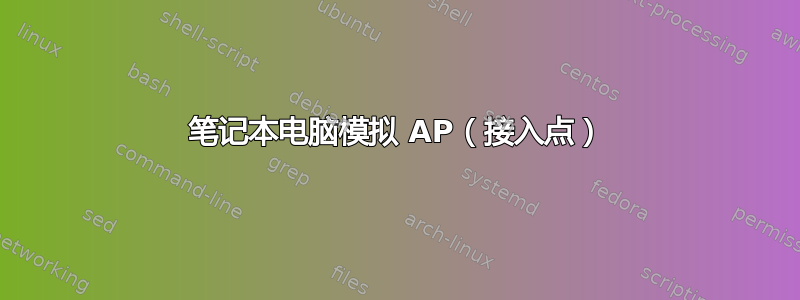
我使用Ubuntu(版本18.04)系统的笔记本电脑来模拟AP。下面是我的Python代码。hostapd.conf
file和dnsmasq.confnew
file与python文件在同一目录下。
- 手机或电脑可以发现该AP并连接
- 移动终端无法扫描到该AP的SSID,无法连接到该AP
我想知道如何解决这个问题。
import subprocess
import time
import textwrap
from scapy.all import *
def create_hostapd_conf(interface, ssid, password):
# Create hostapd.conf content
hostapd_content = textwrap.dedent(f"""
interface={interface}
driver=nl80211
ssid={ssid}
hw_mode=g
channel=8
ieee80211n=1
wpa=2
wpa_passphrase={password}
wpa_key_mgmt=WPA-PSK
wpa_pairwise=CCMP
rsn_pairwise=CCMP
""").strip()
# Write content to hostapd.conf file
with open("hostapd.conf", "w") as hostapd_file:
hostapd_file.write(hostapd_content)
def create_dnsmasq_conf(interface):
# Create dnsmasq.conf content
dnsmasq_content = textwrap.dedent(f"""
interface={interface}
dhcp-range=192.168.1.3,192.168.1.20,255.255.255.0,24h
address=/#/192.168.1.2
port=5353
""").strip()
# Write content to dnsmasq.conf file
with open("dnsmasq.confnew", "w") as dnsmasq_file:
dnsmasq_file.write(dnsmasq_content)
def start_ap(interface, ssid, password):
# Configure hostapd
subprocess.run(["sudo", "service", "network-manager", "stop"])
subprocess.run(["sudo", "ifconfig", interface, "down"])
subprocess.run(["sudo", "iw", "dev", interface, "set", "type", "__ap"])
subprocess.run(["sudo", "ifconfig", interface, "up"])
# Configure static ip
subprocess.run(["sudo","ifconfig", "wlp3s0", "192.168.1.2", "netmask", "255.255.255.0"])
# Start hostapd
print("AAAAAAAAA")
#subprocess.run(["sudo","airmon-ng", "check"])
subprocess.run(["sudo","killall", "wpa_supplicant"])
subprocess.run(["sudo", "service", "hostapd", "-B", "/home/celimuge/python-code/hostapd.conf"])
time.sleep(2)
#subprocess.run(["sudo","service", "hostapd", "start"])
# Configure dnsmasq
print("BBBBBBB")
subprocess.run(["sudo", "dnsmasq", "-C", "/home/celimuge/python-code/dnsmasq.confnew"])
def stop_ap():
# Stop hostapd and dnsmasq
subprocess.run(["sudo", "service", "hostapd", "stop"])
subprocess.run(["sudo", "service", "dnsmasq", "stop"])
subprocess.run(["sudo", "killall", "dnsmasq"])
subprocess.run(["sudo", "service", "network-manager", "start"])
def send_beacon_frame(interface, ssid):
# Create a beacon frame
beacon_frame = Dot11(type=0, subtype=8, addr1="ff:ff:ff:ff:ff:ff", addr2=get_if_hwaddr(interface), addr3=get_if_hwaddr(interface)) / Dot11Beacon(cap="ESS") / Dot11Elt(ID="SSID", info=ssid)
# Send the beacon frame
sendp(beacon_frame, iface=interface, inter=0.1, loop=1)
if __name__ == "__main__":
interface = "wlp3s0" # replace with your wireless interface
ssid = "ap"
password = "12345678"
create_hostapd_conf(interface, ssid, password)
create_dnsmasq_conf(interface)
try:
start_ap(interface, ssid, password)
#send_beacon_frame(interface, ssid)
print("CCCCCCCCC")
time.sleep(150) # Allow time for beacon frames to be sent
print("\nStopping Access Point.")
stop_ap()
except KeyboardInterrupt:
print("\nStopping Access Point.")
stop_ap()