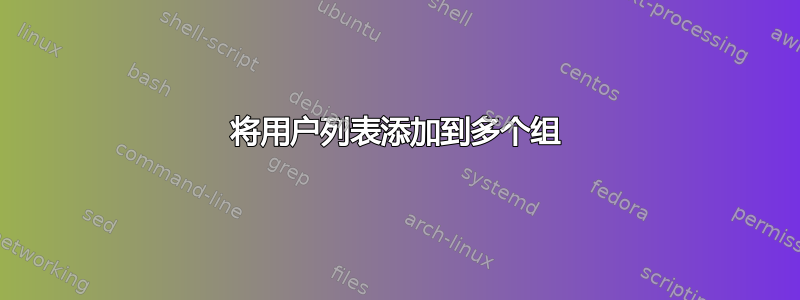
我想编写一个 shell 脚本,它将在 中定义的用户列表添加users.txt
到多个现有组中。
例如,我有a
、b
、c
、d
、 、e
、用户f
,g
这些用户将根据脚本添加到组中,并且我有p
、q
、r
、s
、t
组。以下是文件的预期输出/etc/groups
:
p:x:10029:a,c,d
q:x:10030:b,c,f,g
r:x:10031:a,b,c,e
s:x:10032:c,g
t:x:10033:a,b,c,d,e
那么如何实现这一点呢?
答案1
最好和最简单的方法是将包含所需信息的文件解析为由@DannyG 建议。虽然我自己会这样做,但另一种方法是在脚本中对用户/组组合进行硬编码。例如:
#!/usr/bin/env bash
## Set up an indexed array where the user is the key
## and the groups the values.
declare -A groups=(
["alice"]="groupA,groupB"
["bob"]="groupA,groupC"
["cathy"]="groupB,groupD"
)
## Now, go through each user (key) of the array,
## create the user and add them to the right groups.
for user in "${!groups[@]}"; do
useradd -U -G "${groups[$user]}" "$user"
done
笔记:上面假设 bash 版本 >= 4,因为关联数组在早期版本中不可用。
答案2
由于没有给出输入示例,我将假设一个非常基本的模式:
Uesrs groups
a p,r,t
b p,q
在这种情况下,您有多种选择,因为usermod -G
可以本机使用第二列。
就像是
while read line
do
usermod -G "$(cut -f2 -d" ")" $(cut -f1 -d" ")
done < users.txt
while 循环从 users.txt 中读取每一行,并将其传递给 usermod。
该命令usermod -G group1,group2,group3 user
将用户的组更改为请求的组。
cut
仅根据分隔符分隔字段-d " "
,因此第一个字段用作用户名(登录名),第二个字段用于组。如果您希望将组附加到当前(现有)组 - add -a 所以命令看起来像usermod -a -G ...
答案3
根据您的评论,您可以静态构建脚本,并在其中硬写组。该脚本需要标准输入上的用户列表,每行一个用户。所以用./script < users.txt
例如来称呼它。
#!/bin/bash
groups="p q r" # the list of all groups you want your users in
# the following function is a case statement
# it takes as first argument a user, and as second argument a group
# it returns 0 if the user must be added to the group and 1 otherwise
must_belong_to() {
case $2 in # first we explore all the groups
p)
case $1 in # and for each of them, we examine the users
a | b ) # first selection: the users that must belong to the group
true
;;
*) # second selection: all the others
false
;;
esac
q)
# same here...
;;
esac
}
# we loop on the input file, to process one entry
# (i.e. one user) at a time
while read user
do
# We add the user. You may want to give some options here
# like home directory (-d), password (-p)...
useradd $user
# then we loop on the existing groups to see in which
# one the user must be added
for g in $groups
do
# if the user must be added to the group $g
if must_belong_to $user $g
then
# we add it with the command gpasswd
gpasswd -a $user $g
fi
done
done
正如@terdon 所解释的,这个版本must_belong_to()
可以快速成长。这是使用关联数组的另一种解决方案:
#!/bin/bash
declare -A groups
# we declare all the groups and then, for each one, its members
all_the_groups="a b"
groups[a]="p q r"
groups[b]="q r s"
must_belong_to() {
# we extract a list of all users for the group in parameter
read -a all_the_users <<< "${groups["$2"]}"
# we iterate over the users from the group
for u in $all_the_users
do
# if the user belong to the group,
# we return here
[[ $u == $1 ]] && return 0
done
# in case the user dosn't belong to the group,
# we end up here
return 1
}