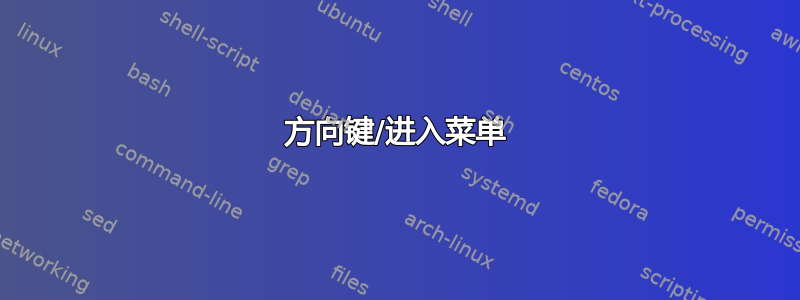
如何在 shell 脚本中创建一个菜单,该菜单将显示 3 个选项,用户将使用箭头键移动突出显示光标并按 Enter 键选择一个选项?
答案1
这是函数bash
形式的纯脚本解决方案select_option
,仅依赖于ANSI 转义序列和内置的read
.
适用于 OSX 上的 Bash 4.2.45。据我所知,在所有环境中可能无法同样有效地工作的时髦部分是get_cursor_row()
, key_input()
(用于检测向上/向下键)和cursor_to()
功能。
#!/usr/bin/env bash
# Renders a text based list of options that can be selected by the
# user using up, down and enter keys and returns the chosen option.
#
# Arguments : list of options, maximum of 256
# "opt1" "opt2" ...
# Return value: selected index (0 for opt1, 1 for opt2 ...)
function select_option {
# little helpers for terminal print control and key input
ESC=$( printf "\033")
cursor_blink_on() { printf "$ESC[?25h"; }
cursor_blink_off() { printf "$ESC[?25l"; }
cursor_to() { printf "$ESC[$1;${2:-1}H"; }
print_option() { printf " $1 "; }
print_selected() { printf " $ESC[7m $1 $ESC[27m"; }
get_cursor_row() { IFS=';' read -sdR -p $'\E[6n' ROW COL; echo ${ROW#*[}; }
key_input() { read -s -n3 key 2>/dev/null >&2
if [[ $key = $ESC[A ]]; then echo up; fi
if [[ $key = $ESC[B ]]; then echo down; fi
if [[ $key = "" ]]; then echo enter; fi; }
# initially print empty new lines (scroll down if at bottom of screen)
for opt; do printf "\n"; done
# determine current screen position for overwriting the options
local lastrow=`get_cursor_row`
local startrow=$(($lastrow - $#))
# ensure cursor and input echoing back on upon a ctrl+c during read -s
trap "cursor_blink_on; stty echo; printf '\n'; exit" 2
cursor_blink_off
local selected=0
while true; do
# print options by overwriting the last lines
local idx=0
for opt; do
cursor_to $(($startrow + $idx))
if [ $idx -eq $selected ]; then
print_selected "$opt"
else
print_option "$opt"
fi
((idx++))
done
# user key control
case `key_input` in
enter) break;;
up) ((selected--));
if [ $selected -lt 0 ]; then selected=$(($# - 1)); fi;;
down) ((selected++));
if [ $selected -ge $# ]; then selected=0; fi;;
esac
done
# cursor position back to normal
cursor_to $lastrow
printf "\n"
cursor_blink_on
return $selected
}
这是一个用法示例:
echo "Select one option using up/down keys and enter to confirm:"
echo
options=("one" "two" "three")
select_option "${options[@]}"
choice=$?
echo "Choosen index = $choice"
echo " value = ${options[$choice]}"
输出如下所示,其中使用反 ansi 着色突出显示当前选定的选项(此处很难在 Markdown 中传达)。如果需要,可以在print_selected()
函数中对此进行调整。
Select one option using up/down keys and enter to confirm:
[one]
two
three
更新:select_opt
这是一个包装上述函数的小扩展,select_option
以便于在语句中使用它case
:
function select_opt {
select_option "$@" 1>&2
local result=$?
echo $result
return $result
}
具有 3 个文字选项的用法示例:
case `select_opt "Yes" "No" "Cancel"` in
0) echo "selected Yes";;
1) echo "selected No";;
2) echo "selected Cancel";;
esac
如果存在一些已知条目(本例中是和否),您还可以混合使用,并利用$?
通配符情况的退出代码:
options=("Yes" "No" "${array[@]}") # join arrays to add some variable array
case `select_opt "${options[@]}"` in
0) echo "selected Yes";;
1) echo "selected No";;
*) echo "selected ${options[$?]}";;
esac
答案2
对话是实现您想要实现的目标的绝佳工具。下面是一个简单的三项选择菜单的示例:
dialog --menu "Choose one:" 10 30 3 \
1 Red \
2 Green \
3 Blue
语法如下:
dialog --menu <text> <height> <width> <menu-height> [<tag><item>]
选择将被发送至stderr
。这是使用 3 种颜色的示例脚本。
#!/bin/bash
TMPFILE=$(mktemp)
dialog --menu "Choose one:" 10 30 3 \
1 Red \
2 Green \
3 Blue 2>$TMPFILE
RESULT=$(cat $TMPFILE)
case $RESULT in
1) echo "Red";;
2) echo "Green";;
3) echo "Blue";;
*) echo "Unknown color";;
esac
rm $TMPFILE
在 Debian 上,您可以dialog
通过以下方式安装同名包。
答案3
问题仅涉及一项选择。
如果您正在寻找多选菜单,这里有纯bash其实施:
使用
j/k或↑/↓箭头键向上或向下导航
⎵(空格)以切换选择,并
⏎使用 (Enter) 确认选择。
可以这样调用:
my_options=( "Option 1" "Option 2" "Option 3" )
preselection=( "true" "true" "false" )
multiselect result my_options preselection
该函数的最后一个参数multiselect
是可选的,可用于预先选择某些选项。
结果将作为数组存储在作为第一个参数传递的变量中multiselect
。下面是将选项与结果结合起来的示例:
idx=0
for option in "${my_options[@]}"; do
echo -e "$option\t=> ${result[idx]}"
((idx++))
done
function multiselect {
# little helpers for terminal print control and key input
ESC=$( printf "\033")
cursor_blink_on() { printf "$ESC[?25h"; }
cursor_blink_off() { printf "$ESC[?25l"; }
cursor_to() { printf "$ESC[$1;${2:-1}H"; }
print_inactive() { printf "$2 $1 "; }
print_active() { printf "$2 $ESC[7m $1 $ESC[27m"; }
get_cursor_row() { IFS=';' read -sdR -p $'\E[6n' ROW COL; echo ${ROW#*[}; }
local return_value=$1
local -n options=$2
local -n defaults=$3
local selected=()
for ((i=0; i<${#options[@]}; i++)); do
if [[ ${defaults[i]} = "true" ]]; then
selected+=("true")
else
selected+=("false")
fi
printf "\n"
done
# determine current screen position for overwriting the options
local lastrow=`get_cursor_row`
local startrow=$(($lastrow - ${#options[@]}))
# ensure cursor and input echoing back on upon a ctrl+c during read -s
trap "cursor_blink_on; stty echo; printf '\n'; exit" 2
cursor_blink_off
key_input() {
local key
IFS= read -rsn1 key 2>/dev/null >&2
if [[ $key = "" ]]; then echo enter; fi;
if [[ $key = $'\x20' ]]; then echo space; fi;
if [[ $key = "k" ]]; then echo up; fi;
if [[ $key = "j" ]]; then echo down; fi;
if [[ $key = $'\x1b' ]]; then
read -rsn2 key
if [[ $key = [A || $key = k ]]; then echo up; fi;
if [[ $key = [B || $key = j ]]; then echo down; fi;
fi
}
toggle_option() {
local option=$1
if [[ ${selected[option]} == true ]]; then
selected[option]=false
else
selected[option]=true
fi
}
print_options() {
# print options by overwriting the last lines
local idx=0
for option in "${options[@]}"; do
local prefix="[ ]"
if [[ ${selected[idx]} == true ]]; then
prefix="[\e[38;5;46m✔\e[0m]"
fi
cursor_to $(($startrow + $idx))
if [ $idx -eq $1 ]; then
print_active "$option" "$prefix"
else
print_inactive "$option" "$prefix"
fi
((idx++))
done
}
local active=0
while true; do
print_options $active
# user key control
case `key_input` in
space) toggle_option $active;;
enter) print_options -1; break;;
up) ((active--));
if [ $active -lt 0 ]; then active=$((${#options[@]} - 1)); fi;;
down) ((active++));
if [ $active -ge ${#options[@]} ]; then active=0; fi;;
esac
done
# cursor position back to normal
cursor_to $lastrow
printf "\n"
cursor_blink_on
eval $return_value='("${selected[@]}")'
}
信用:这个bash函数是一个定制版本Denis Semenenko 的实施。
答案4
这里有一些构建交互式 shell 菜单的绝佳解决方案;尤其是@miu 和@alexanderklimitschek 的作品。我正在寻找类似的东西,但代码稍微少一点,并且它必须可以与 ZSH 一起使用(使用 ZSH shebang #!/usr/bin/env zsh
)。此外,我不想要像dialog
.
但是这里和类似网站上所有那些非常酷的脚本都是为纯bash。由于数组索引、转义序列Enter和一些其他键或内置read
命令中的差异等原因,这使得它们与 ZSH 不兼容。因此,我必须自己为ZSH写一个。我从用户 @Guss 那里采用了这些简单的 bash 方法询问Ubuntu并针对 ZSH 进行了调整。也许对纯 ZSH 脚本有类似需求的人也可以使用它。
#!/usr/bin/env zsh
############################################################################
# zsh script which offers interactive selection menu
#
# based on the answer by Guss on https://askubuntu.com/a/1386907/1771279
function choose_from_menu() {
local prompt="$1" outvar="$2"
shift
shift
# count had to be assigned the pure number of arguments
local options=("$@") cur=1 count=$# index=0
local esc=$(echo -en "\033") # cache ESC as test doesn't allow esc codes
echo -n "$prompt\n\n"
# measure the rows of the menu, needed for erasing those rows when moving
# the selection
menu_rows=$#
total_rows=$(($menu_rows + 1))
while true
do
index=1
for o in "${options[@]}"
do
if [[ "$index" == "$cur" ]]
then echo -e " \033[38;5;41m>\033[0m\033[38;5;35m$o\033[0m" # mark & highlight the current option
else echo " $o"
fi
index=$(( $index + 1 ))
done
printf "\n"
# set mark for cursor
printf "\033[s"
# read in pressed key (differs from bash read syntax)
read -s -r -k key
if [[ $key == k ]] # move up
then cur=$(( $cur - 1 ))
[ "$cur" -lt 1 ] && cur=1 # make sure to not move out of selections scope
elif [[ $key == j ]] # move down
then cur=$(( $cur + 1 ))
[ "$cur" -gt $count ] && cur=$count # make sure to not move out of selections scope
elif [[ "${key}" == $'\n' || $key == '' ]] # zsh inserts newline, \n, for enter - ENTER
then break
fi
# move back to saved cursor position
printf "\033[u"
# erase all lines of selections to build them again with new positioning
for ((i = 0; i < $total_rows; i++)); do
printf "\033[2k\r"
printf "\033[F"
done
done
# pass choosen selection to main body of script
eval $outvar="'${options[$cur]}'"
}
# explicitly declare selections array makes it safer
declare -a selections
selections=(
"Selection A"
"Selection B"
"Selection C"
"Selection D"
"Selection E"
)
# call function with arguments:
# $1: Prompt text. newline characters are possible
# $2: Name of variable which contains the selected choice
# $3: Pass all selections to the function
choose_from_menu "Please make a choice:" selected_choice "${selections[@]}"
echo "Selected choice: $selected_choice"
这里有一个小演示。移动到带有 和 的行j并k选择带有 的选项Enter: