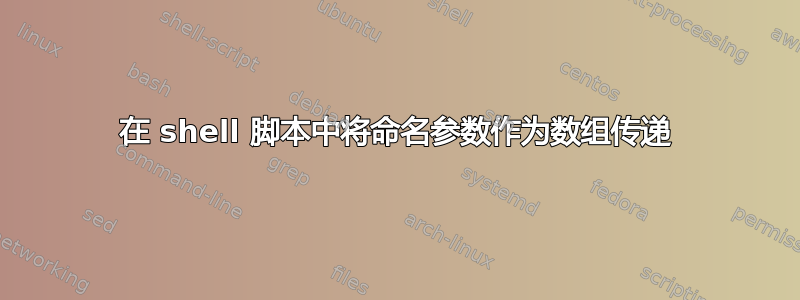
我当前正在构建的工具中有以下代码:
while [ $# -gt 0 ]; do
case "$1" in
--var1=*)
var1="${1#*=}"
;;
--var2=*)
var1="${1#*=}"
;;
--var3=*)
var1="${1#*=}"
;;
*)
printf "***************************\n
* Error: Invalid argument.*\n
***************************\n"
esac
shift
done
我有很多选项要添加,但其中五个选项应保存为数组。因此,如果我从 shell 调用该工具,可以使用如下所示的命令:
./tool --var1="2" --var1="3" --var1="4" --var1="5" --var2="6" --var3="7"
如何将 的值保存var1
为数组?那可能吗?如果是这样,如果我有太多数组,从效率角度来看,处理这些数组的最佳方法是什么?
答案1
如果在 Linux 上(util-linux
已getopt
安装实用程序,或来自 的实用程序busybox
),您可以执行以下操作:
declare -A opt_spec
var1=() var2=() var4=false
unset var3
opt_spec=(
[opt1:]='var1()' # opt with argument, array variable
[opt2:]='var2()' # ditto
[opt3:]='var3' # opt with argument, scalar variable
[opt4]='var4' # boolean opt without argument
)
parsed_opts=$(
IFS=,
getopt -o + -l "${!opt_spec[*]}" -- "$@"
) || exit
eval "set -- $parsed_opts"
while [ "$#" -gt 0 ]; do
o=$1; shift
case $o in
(--) break;;
(--*)
o=${o#--}
if ((${opt_spec[$o]+1})); then # opt without argument
eval "${opt_spec[$o]}=true"
else
o=$o:
case "${opt_spec[$o]}" in
(*'()') eval "${opt_spec[$o]%??}+=(\"\$1\")";;
(*) eval "${opt_spec[$o]}=\$1"
esac
shift
fi
esac
done
echo "var1: ${var1[@]}"
这样,您就可以将脚本称为:
my-script --opt1=foo --opt2 bar --opt4 -- whatever
--
getopt 将为您完成解析、处理和缩写的艰苦工作。
或者,您可以依赖变量的类型,而不是在$opt_spec
关联数组定义中指定它:
declare -A opt_spec
var1=() var2=() var4=false
unset var3
opt_spec=(
[opt1:]=var1 # opt with argument
[opt2:]=var2 # ditto
[opt3:]=var3 # ditto
[opt4]=var4 # boolean opt without argument
)
parsed_opts=$(
IFS=,
getopt -o + -l "${!opt_spec[*]}" -- "$@"
) || exit
eval "set -- $parsed_opts"
while [ "$#" -gt 0 ]; do
o=$1; shift
case $o in
(--) break;;
(--*)
o=${o#--}
if ((${opt_spec[$o]+1})); then # opt without argument
eval "${opt_spec[$o]}=true"
else
o=$o:
case $(declare -p "${opt_spec[$o]}" 2> /dev/null) in
("declare -a"*) eval "${opt_spec[$o]}+=(\"\$1\")";;
(*) eval "${opt_spec[$o]}=\$1"
esac
shift
fi
esac
done
echo "var1: ${var1[@]}"
您可以添加简短选项,例如:
declare -A long_opt_spec short_opt_spec
var1=() var2=() var4=false
unset var3
long_opt_spec=(
[opt1:]=var1 # opt with argument
[opt2:]=var2 # ditto
[opt3:]=var3 # ditto
[opt4]=var4 # boolean opt without argument
)
short_opt_spec=(
[a:]=var1
[b:]=var2
[c]=var3
[d]=var4
)
parsed_opts=$(
IFS=; short_opts="${!short_opt_spec[*]}"
IFS=,
getopt -o "+$short_opts" -l "${!long_opt_spec[*]}" -- "$@"
) || exit
eval "set -- $parsed_opts"
while [ "$#" -gt 0 ]; do
o=$1; shift
case $o in
(--) break;;
(--*)
o=${o#--}
if ((${long_opt_spec[$o]+1})); then # opt without argument
eval "${long_opt_spec[$o]}=true"
else
o=$o:
case $(declare -p "${long_opt_spec[$o]}" 2> /dev/null) in
("declare -a"*) eval "${long_opt_spec[$o]}+=(\"\$1\")";;
(*) eval "${long_opt_spec[$o]}=\$1"
esac
shift
fi;;
(-*)
o=${o#-}
if ((${short_opt_spec[$o]+1})); then # opt without argument
eval "${short_opt_spec[$o]}=true"
else
o=$o:
case $(declare -p "${short_opt_spec[$o]}" 2> /dev/null) in
("declare -a"*) eval "${short_opt_spec[$o]}+=(\"\$1\")";;
(*) eval "${short_opt_spec[$o]}=\$1"
esac
shift
fi
esac
done
echo "var1: ${var1[@]}"
答案2
我建议你看看我的通用 Shell 脚本 GitHub:utility_functions.sh。在那里你会看到一个名为的函数获取参数。它旨在将选项和值关联起来。
我仅在此处粘贴该函数,但它取决于该脚本中的其他几个函数
##########################
#
# Function name: getArgs
#
# Description:
# This function provides the getopts functionality
# while allowing the use of long operations and list of parameters.
# in the case of a list of arguments for only one option, this list
# will be returned as a single-space-separated list in one single string.
#
# Pre-reqs:
# None
#
# Output:
# GA_OPTION variable will hold the current option
# GA_VALUE variable will hold the value (or list of values) associated
# with the current option
#
# Usage:
# You have to source the function in order to be able to access the GA_OPTIONS
# and GA_VALUES variables
# . getArgs $*
#
####################
function getArgs {
# Variables to return the values out of the function
typeset -a GA_OPTIONS
typeset -a GA_VALUES
# Checking for number of arguments
if [[ -z $1 ]]
then
msgPrint -warning "No arguments found"
msgPrint -info "Please call this function as follows: . getArgs \$*"
exit 0
fi
# Grab the dash
dash=$(echo $1 | grep "-")
# Looking for short (-) or long (--) options
isOption=$(expr index "$dash" "-")
# Initialize the counter
counter=0
# Loop while there are arguments left
while [[ $# -gt 0 ]]
do
if [[ $dash && $isOption -eq 1 ]]
then
(( counter+=1 ))
GA_OPTIONS[$counter]=$1
shift
else
if [[ -z ${GA_VALUES[$counter]} ]]
then
GA_VALUES[$counter]=$1
else
GA_VALUES[$counter]="${GA_VALUES[$counter]} $1"
fi
shift
fi
dash=$(echo $1 | grep "-")
isOption=$(expr index "$dash" "-")
done
# Make the variables available to the main algorithm
export GA_OPTIONS
export GA_VALUES
msgPrint -debug "Please check the GA_OPTIONS and GA_VALUES arrays for options and arguments"
# Exit with success
return 0
}
如您所见,此特定函数将导出 GA_OPTIONS 和 GA_VALUES。唯一的条件是值必须是选项后面以空格分隔的列表。
您可以将该脚本称为 ./tool --var1 2 3 4 5 --var2="6" --var3="7"
或者只是使用类似的逻辑来适应您的偏好。