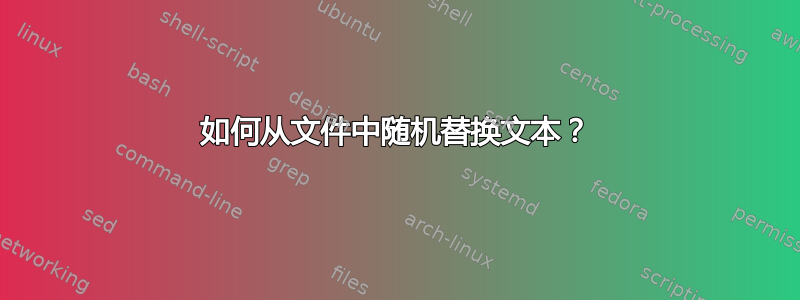
如何用另一个文件中的字符串随机替换一个文本文件中的特定字符串?例如:
file1.txt(file has more than 200 lines):
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
file2.txt(file has 10-20 lines):
@adress1.com
@adress2.com
@adress3.com
@adress4.com
@adress5.com
output.txt:
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
答案1
你可以实现这个算法:
- 加载内容
file2.txt
到数组中 - 对于中的每一行
file1.txt
:- 提取姓名部分
- 获取随机地址
- 打印格式正确的输出
像这样:
mapfile -t addresses < file2.txt
while IFS='' read -r orig || [[ -n "$orig" ]]; do
((index = RANDOM % ${#addresses[@]}))
name=${orig%%@*}
echo "$name${addresses[index]}"
done < file1.txt
(特别感谢@GlennJackman 和@dessert 的改进。)
答案2
如果你真的想要随机选择,那么这里有一种方法awk
:
awk '
BEGIN{FS="@"; OFS=""}
NR==FNR{a[NR]=$0; n++; next}
{$2=a[int(1 + n * rand())]; print}
' file2.txt file1.txt
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
另一方面,如果你想要随机排列地址,我建议
paste -d '' <(cut -d'@' -f1 file1.txt) <(sort -R file2.txt)
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
答案3
您可以使用shuf
(您可能需要sudo apt install shuf
)来随机排列第二个文件的行,然后使用它们来替换:
$ awk -F'@' 'NR==FNR{a[NR]=$1;next}{print a[FNR]"@"$2} ' file1 <(shuf file2)
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
shuf
只是随机化输入行的顺序。该awk
命令将首先读取 file1 的所有内容(NR==FNR
仅在读取第一个文件时才为真),并将第二个字段(字段由 定义@
,因此这是域)保存在关联数组中,a
该数组的值是域,其键是行号。然后,当我们到达下一个文件时,它将简单地打印此行号存储的内容a
,以及文件 2 中相同行号的内容。
请注意,这假设两个文件的行数完全相同,并且实际上并不是“随机的”,因为它不允许任何内容重复。但这看起来就是您想要的。
答案4
这是一个 perl 方法:
#!/usr/bin/perl
use warnings;
use strict;
use Tie::File;
tie my @file1,'Tie::File','file1.txt' or die "Can't open file1.txt\n";
tie my @file2,'Tie::File','file2.txt' or die "Can't open file2.txt\n";
for my $file_index (0..$#file1) {
my $suffix = $file2[int(rand($#file2+1))];
$file1[$file_index] =~ s/@.*$/$suffix/;
}
untie @file1;
untie @file2;