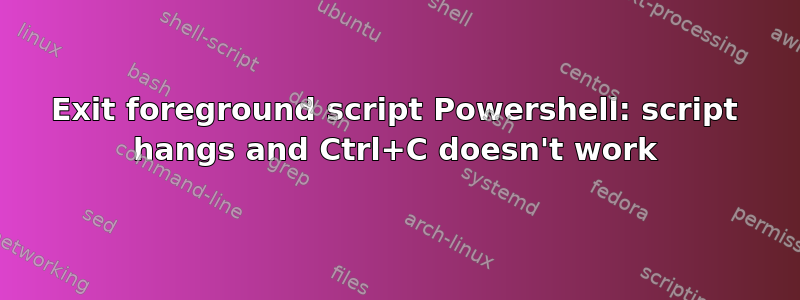
I have a powershell script running as follows:
> $client = New-Object System.Net.Sockets.TCPClient('x.x.x.x',443);$stream = $client.GetStream();[byte[]]$bytes = 0..65535|%{0};while(($i = $stream.Read($bytes, 0, $bytes.Length)) -ne 0){;$data = (New-Object -TypeName System.Text.ASCIIEncoding).GetString($bytes,0, $i);[Sytem.IO.File]::AppendAllText('<path\to\file>', $data)};$client.Close();
It connects, reads the stream, writes to file, but does not exit. I then try to use Ctrl+C and it does not work.
I want it to exit when it has finished reading the file, also would like to know why it doesn't exit using Ctrl+C like on Linux.
答案1
If you are in a PowerShell session and run this code, it is in the current session. Once your code completes, you get returned to the Powershell prompt from where you started the code. Thus your code is closed, not your session.
CRTL+C does not close PowerShell after your code has run, exit does.
You are only closing the Socket connection object. You are not exiting the PowerShell session from where you started it.
# Open the TCP socket
$client = New-Object System.Net.Sockets.TCPClient('x.x.x.x',443)
# Initialize the stream
$stream = $client.GetStream()
[byte[]]$bytes = 0..65535 |
ForEach{0}
while(($i = $stream.Read($bytes, 0, $bytes.Length)) -ne 0)
{
# Collect the data
$data = (New-Object -TypeName System.Text.ASCIIEncoding).GetString($bytes,0, $i)
# Write data to file
[Sytem.IO.File]::AppendAllText('<path\to\file>', $data)
}
# Clear the stream
$stream.Flush()
# Close the TCP socket
$client.Close()
# Code complete, exiting PowerShell
Exit
If you want to run a foreground code block from a PowerShell session, You can use Start-Process.
For example:
# Start a new foreground PowerShell session to run this code
Start-Process -FilePath PowerShell -ArgumentList Get-Date
You don't need exit doing this or CRTL+C efforts, as it will autoclose the session once the code is done.
See the help files on how to leverage Start-Process options and the PowerShell command-line options.
# Get specifics for a module, cmdlet, or function
(Get-Command -Name Start-Process).Parameters
(Get-Command -Name Start-Process).Parameters.Keys
Get-help -Name Start-Process -Examples
Get-help -Name Start-Process -Full
Get-help -Name Start-Process -Online
Powershell /?