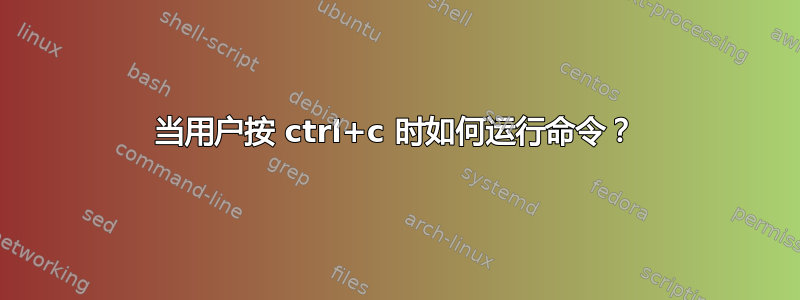
用户选择任何文本并按 ctrl+c。command
此操作后如何自动运行?
我需要以下解决方案:
- 如何获取有关剪贴板状态的通知/检查
- 通知/检查后将自动运行命令
我不知道。
答案1
可以监控X11剪贴板。这仅适用于 X11,不适用于控制台复制和粘贴、tmux 或其他任何东西。因此,可移植性可能值得怀疑,您可能需要监视所有三个剪贴板,具体取决于您的需求。
// whenclipchange.c
// Run something when a X11 clipboard changes. Note that PRIMARY tends
// to be the traditional default, while certain software instead uses
// CLIPBOARD for I don't know what incompatible reason. There is also
// SECONDARY to make your life more interesting.
#define WHATCLIP "PRIMARY"
#include <sys/wait.h>
#include <assert.h>
#include <err.h>
#include <limits.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <X11/Xlib.h>
#include <X11/extensions/Xfixes.h>
void WatchSelection(Display *display, Window window, const char *bufname,
char *argv[]);
int
main(int argc, char *argv[])
{
#ifdef __OpenBSD__
if (pledge("exec inet proc rpath stdio unix", NULL) == -1)
err(1, "pledge failed");
#endif
if (argc < 2) err(1, "need a command to run");
argv++; // skip past command name of this program
Display *display = XOpenDisplay(NULL);
unsigned long color = BlackPixel(display, DefaultScreen(display));
Window window = XCreateSimpleWindow(display, DefaultRootWindow(display),
0, 0, 1, 1, 0, color, color);
WatchSelection(display, window, WHATCLIP, argv);
/* NOTREACHED */
XDestroyWindow(display, window);
XCloseDisplay(display);
exit(EXIT_FAILURE);
}
void
WatchSelection(Display *display, Window window, const char *bufname,
char *argv[])
{
int event_base, error_base;
XEvent event;
Atom bufid = XInternAtom(display, bufname, False);
assert(XFixesQueryExtension(display, &event_base, &error_base));
XFixesSelectSelectionInput(display, DefaultRootWindow(display), bufid,
XFixesSetSelectionOwnerNotifyMask);
while (1) {
XNextEvent(display, &event);
if (event.type == event_base + XFixesSelectionNotify &&
((XFixesSelectionNotifyEvent *) &event)->selection ==
bufid) {
pid_t pid = fork();
if (pid < 0) err(1, "fork failed");
if (pid) {
// NOTE this will block until the
// command finishes... so it might miss
// clipboard events?
int status;
wait(&status);
} else {
execvp(*argv, argv);
exit(EXIT_FAILURE);
}
}
}
}
编译并运行类似...
$ cc -std=c99 -I/usr/X11R6/include -L/usr/X11R6/lib -lX11 -lXft -lXfixes -o whenclipchange whenclipchange.c
$ ./whenclipchange echo clipboard changed