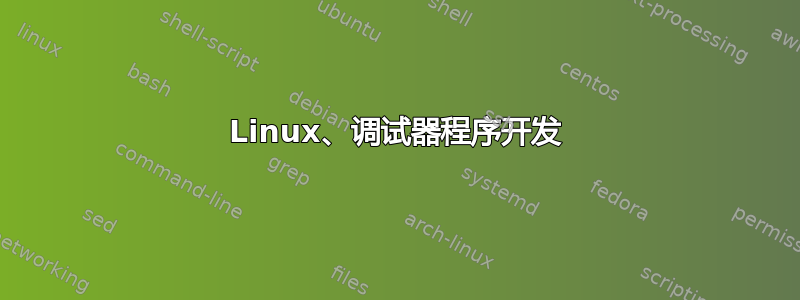
我们正在尝试实现调试器程序,该程序将 PID 或程序名称作为输入,并使用 PID 调用 gdb。下面是编写的两个小程序,无法弄清楚这里的确切问题是什么...通过PID后,结果显示执行了5000+条指令。
调试.c
#include <stdio.h>
#include <stdarg.h>
#include <stdlib.h>
#include <signal.h>
#include <syscall.h>
#include <sys/ptrace.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <sys/reg.h>
#include <sys/user.h>
#include <unistd.h>
#include <errno.h>
/* Print a message to stdout, prefixed by the process ID
*/
void procmsg(const char* format, ...)
{
va_list ap;
fprintf(stdout, "[%d] ", getpid());
va_start(ap, format);
vfprintf(stdout, format, ap);
va_end(ap);
}
void run_target(const char* programname)
{
procmsg("target started. will run '%s'\n", programname);
/* Allow tracing of this process */
if (ptrace(PTRACE_TRACEME, 0, 0, 0) < 0) {
perror("ptrace");
return;
}
/* Replace this process's image with the given program */
execl(programname, programname, 0);
}
void run_debugger(pid_t child_pid)
{
int wait_status;
unsigned icounter = 0;
procmsg("debugger started\n");
/* Wait for child to stop on its first instruction */
wait(&wait_status);
while (WIFSTOPPED(wait_status)) {
icounter++;
struct user_regs_struct regs;
ptrace(PTRACE_GETREGS, child_pid, 0, ®s);
unsigned instr = ptrace(PTRACE_PEEKTEXT, child_pid, regs.eip, 0);
procmsg("icounter = %u. EIP = 0x%08x. instr = 0x%08x\n",
icounter, regs.eip, instr);
/* Make the child execute another instruction */
if (ptrace(PTRACE_SINGLESTEP, child_pid, 0, 0) < 0) {
perror("ptrace");
return;
}
/* Wait for child to stop on its next instruction */
wait(&wait_status);
}
procmsg("the child executed %u instructions\n", icounter);
}
int main(int argc, char** argv)
{
pid_t child_pid_attach;
if (argc < 2) {
fprintf(stderr, "Expected a program name as argument\n");
return -1;
}
sscanf(argv[1],"%d",&child_pid_attach);
//Attaching to running process
if(ptrace(PTRACE_ATTACH,child_pid_attach,0,0)<)
{
perror("ptrace");
return;
}
else
{
printf("%d",child_pid_attach);
}
if (child_pid_attach== 0)
run_target(argv[1]);
else if (child_pid_attach > 0)
run_debugger(child_pid_attach);
else {
perror("fork");
return -1;
}
ptrace(PTRACE_DETACH,child_pid_attach,0,0);
return 0;
}
上面的程序已用于调试由以下程序创建的进程(即两个数字的和)。测试.c
#include<stdio.h>
void main()
{
int a, b, c;
scanf("%d", &a);
scanf("%d", &b);
printf("\n Sum of Two Numbers is:");
c=a+b;
printf("%d",c);
}
首先,我们运行 ./test 然后检查它的 pid。下一步我们将运行 ./Debug [pid]。上述执行的结果是,显示子进程已执行 5000 多条指令,并且始终打印相同的指令。
请让我知道是否有其他方法可以做到这一点,并让我知道如何读取另一个进程的数据。在这种情况下,“如何读取 ./test 创建的进程的数据(变量值)?”。
答案1
事实上,这是正确的行为。
以下引用自这里:
答案很有趣。默认情况下,Linux 上的 gcc 动态地将程序链接到 C 运行时库。这意味着,执行任何程序时首先运行的事情之一就是查找所需共享库的动态库加载器。这是相当多的代码 - 请记住,我们的基本跟踪器会查看每条指令,不仅仅是主函数,而是整个过程。
答案2
“如何读取 ./test 创建的进程的数据(变量值)?”。
您可能想研究一下 DWARF 调试格式。第 3 部分调试器如何工作下面的链接简要讨论了 DWARF。还有其他方法可以解析符号,但为什么不像 GDB 那样使用 DWARF 呢? [编辑:从 gdb 源代码中提取函数以在更简单的程序中使用它们并不是一件简单的任务]。无论如何,源代码都是可用的,请检查'GDB 如何加载符号文件' 下面的链接,链接就指向它。第三个选项是使用您自己的函数手动解析 ELF 符号表。它更丑陋,可能是更复杂的道路,但它不依赖 dwarf 提供的调试符号。
至于断点,您可以使用 ptrace 和陷阱 = 内存 & 0xffffff00 | 0xcc将指令保存在地址处并在命中陷阱后恢复指令后,如下调试器如何工作描述。 0xcc字节是操作码整数 3.
要了解 gdb 如何执行此操作,请点击以下链接: GDB 如何加载符号文件
以下只是通过类似的跟踪器运行没有链接库的汇编程序来暗示这 5000 多个步骤的来源:
;hello.asm
section .text
global _start
_start:
mov edx,5
mov ecx,msg
mov ebx,1
mov eax,4
int 0x80
mov eax,1
int 0x80
msg:
db "Hello"
我用来计数的程序是类似的(来自调试器如何工作)
#include <sys/ptrace.h>
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/wait.h>
void run_debugger(pid_t child_pid)
{
int wait_status;
unsigned icounter = 0;
printf("debugger started\n");
/* Wait for child to stop on its first instruction */
wait(&wait_status);
while (WIFSTOPPED(wait_status)) {
icounter++;
/* Make the child execute another instruction */
if (ptrace(PTRACE_SINGLESTEP, child_pid, 0, 0) < 0) {
perror("ptrace");
return;
}
/* Wait for child to stop on its next instruction */
wait(&wait_status);
}
printf("\nthe child executed %u instructions\n", icounter);
}
void run_target(const char* programname)
{
printf("target started. will run '%s'\n", programname);
/* Allow tracing of this process */
if (ptrace(PTRACE_TRACEME, 0, 0, 0) < 0) {
perror("ptrace");
return;
}
/* Replace this process's image with the given program */
execl(programname, programname, NULL);
}
int main(int argc, char** argv)
{
pid_t child_pid;
if (argc < 2) {
fprintf(stderr, "Expected a program name as argument\n");
return -1;
}
child_pid = fork();
if (child_pid == 0)
run_target(argv[1]);
else if (child_pid > 0)
run_debugger(child_pid);
else {
perror("fork");
return -1;
}
return 0;
}
将其编译为 a.out 并运行:
$ ./a.out helloasm
debugger started
target started. will run 'helloasm'
Hello
the child executed 7 instructions
与
#include <stdio.h>
int main()
{
printf("Hello World\n");
return 0;
}
总共 141 690 条指令。