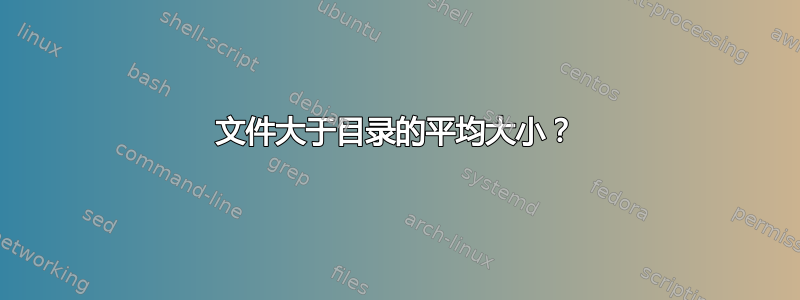
如何找到所有大小大于或等于当前目录平均文件大小的文件和目录?
答案1
avg_size=$(find . -maxdepth 1 -type f -printf %s\\n |
{ sum=0; files=0; while read size; do sum=$((sum+size)); ((files++)); done; echo "$((sum/files))"; })
echo "average file size: ${avg_size}"
find . -maxdepth 1 -type f -size +"$avg_size"c
答案2
如果您有 GNU 工具:
find -maxdepth 1 -type f -printf '%s %p\0' |
awk -v RS='\0' '{a[$0]=$1;s+=$1;}
END{m=s/NR; for(i in a){if(a[i]>=m){print i}}}'
如果你不这样做:
perl -le 'opendir(D,"."); @F=readdir(D); @files=grep{-f $_}@F;
for (@files){$s= -s $_; $t+=$s; $f{$_}=$s}
print join "\n",grep{$f{$_}>=$t/scalar(@files)}@files'
可以扩展到:
#!/usr/bin/perl
## Open the current directory as D
opendir(D,".");
## Read the contents of D into @F
@F=readdir(D);
## Collect only the files, no dirs etc.
@files=grep{-f $_}@F;
## For each file, as $_
for (@files){
## Get the size
$s= -s $_;
## Add the size to the total
$t+=$s;
## Save the size in the hash %f whose keys are the file names
$f{$_}=$s
}
## Get all files whose size is greater than the average
my @wanted=grep{$f{$_}>=$t/scalar(@files)}@files;
## Print the elements of the array @wanted with a newline after each of them
print join "\n", @wanted ;
print "\n";
答案3
这是一个特定于 bash 的解决方案;它循环遍历文件,将它们的大小和名称收集到索引的 bash 数组中,然后计算平均值并循环返回数组,仅打印那些严格大于(整数)平均大小的文件名。
#!/bin/bash
declare -i sum=0
declare -i nfiles=0
declare -a filenames
declare -a filesizes
for file in *
do
[ -f "$file" ] || continue
size=$(stat -c %s -- "$file")
filenames[$nfiles]="$file"
filesizes[$nfiles]=$size
sum+=$size
nfiles+=1
done
[ $nfiles -eq 0 ] && exit
avg=$(( sum / nfiles ))
for((index=0; index < ${#filenames[*]}; ++index))
do
[ ${filesizes[$index]} -gt $avg ] && printf "%s\n" "${filenames[$index]}"
done
答案4
#!/bin/bash
no=$(ls -l | wc -l)
find_files(){
if [ $(ls -l | awk '{print $5}' | awk 'FNR=='$1'') -ge $2 ]
then
echo $(ls -l | awk '{print $9}' | awk 'FNR=='$1'')
fi
}
average(){
local count=2
sum=0
while [ $count -le $no ]
do
let sum=sum+$(ls -l | awk '{print $5}' | awk 'FNR=='$count'')
((count++))
done
let num=$no-1
let avg=$sum/$num
echo $avg
}
main(){
a=$(average)
i=2
while [ $i -le $no ]
do
find_files $i $a
((i++))
d
}
main